How do you use bcrypt for hashing passwords in PHP?
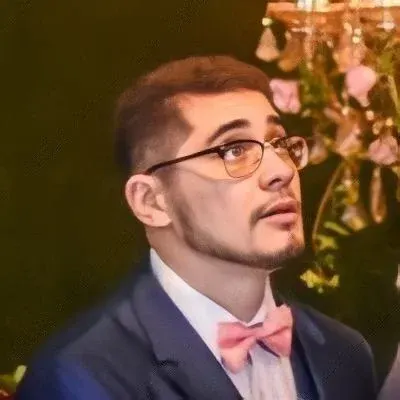
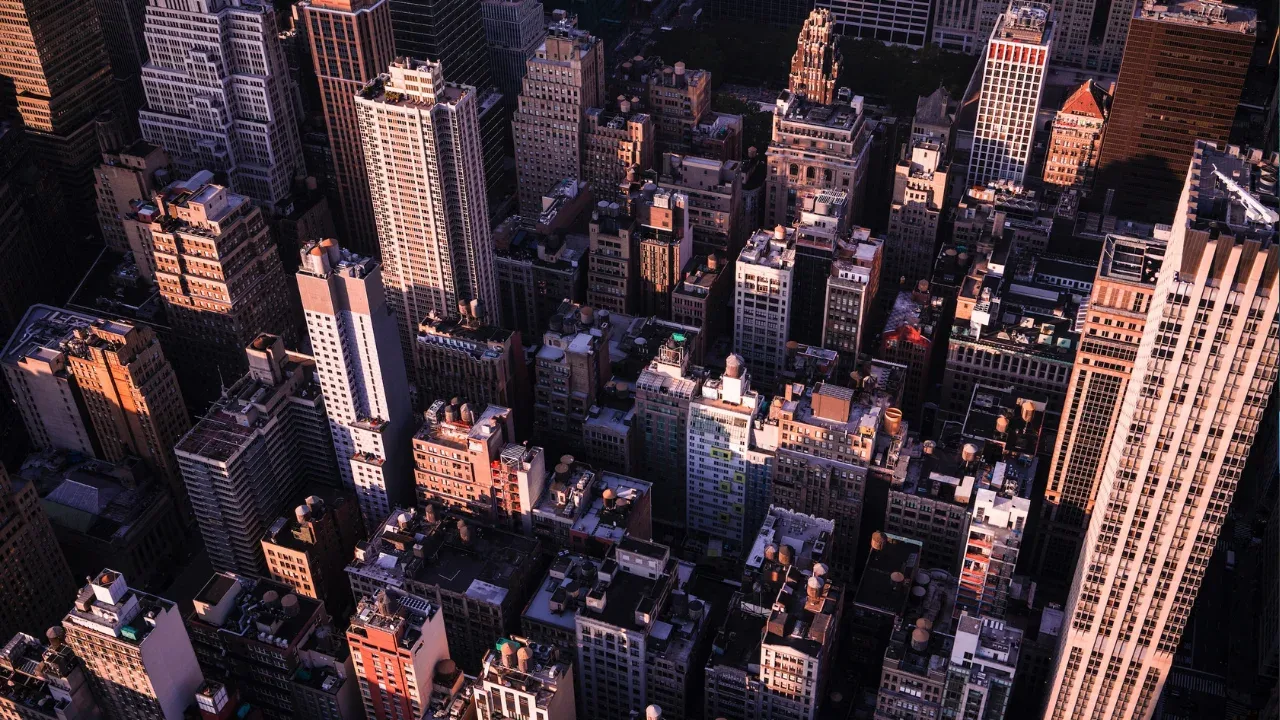
How to Use bcrypt for Hashing Passwords in PHP 😎🔐💻
Every now and then, you may come across the advice to "Use bcrypt for storing passwords in PHP, bcrypt rules." But what exactly is bcrypt, and how can it help you securely store passwords in your PHP applications? 🤷♂️
Understanding the Problem ❓🔍
PHP itself doesn't provide a built-in function for bcrypt, which can lead to confusion. When you search for bcrypt, you might stumble upon discussions about file encryption utilities or various Blowfish implementations in different programming languages. While Blowfish is available in PHP through the mcrypt
extension, it doesn't directly address the need for one-way hashing of passwords. 😕
Introducing bcrypt 🎉🔒
In reality, bcrypt is not a PHP-specific function, but rather a widely used password hashing algorithm designed for secure storage. It utilizes the Blowfish cipher and incorporates a salt to further enhance password security. bcrypt is considered one of the most secure ways to hash passwords and is highly recommended for PHP applications.
How to Implement bcrypt in PHP 🛠️🔨
To use bcrypt in PHP, you can leverage the password_hash()
function, introduced in PHP 5.5. This function simplifies the process of hashing passwords using bcrypt. Here's an example:
$password = "supersecret";
$hashedPassword = password_hash($password, PASSWORD_BCRYPT);
The password_hash()
function takes two parameters: the password you want to hash and the algorithm you want to use (in this case, PASSWORD_BCRYPT
). It then returns the hashed password, ready to be stored in your database. Easy, right? 🙌
Comparing Hashed Passwords 🤝✅
When it comes to verifying passwords, bcrypt has got you covered. To check if a provided password matches the hashed password stored in your database, you can use the password_verify()
function. Here's how:
$password = "supersecret";
$hashedPassword = password_hash($password, PASSWORD_BCRYPT);
// Assume $storedPassword is the hashed password retrieved from your database
if (password_verify($password, $storedPassword)) {
echo "Passwords match!";
} else {
echo "Passwords don't match!";
}
The password_verify()
function takes two parameters: the password you want to check and the hashed password retrieved from your database. It then compares the two and returns true
if they match, or false
if they don't. Simple and secure! 🔒🔑
Take Your Password Security to the Next Level 🚀🔒
By now, you should have a clear understanding of how to use bcrypt for hashing passwords in PHP. It's a powerful and secure solution that you can easily implement in your applications. Remember, password security is crucial, so don't settle for less! 😉
If you found this guide helpful, be sure to share it with your fellow developers. And feel free to leave a comment below if you have any questions or additional insights. Happy coding! 👩💻👨💻💬
📢 Join the discussion: How do you ensure password security in your PHP applications? 🤔🔒
📌🗣️ Let us know in the comments below!
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
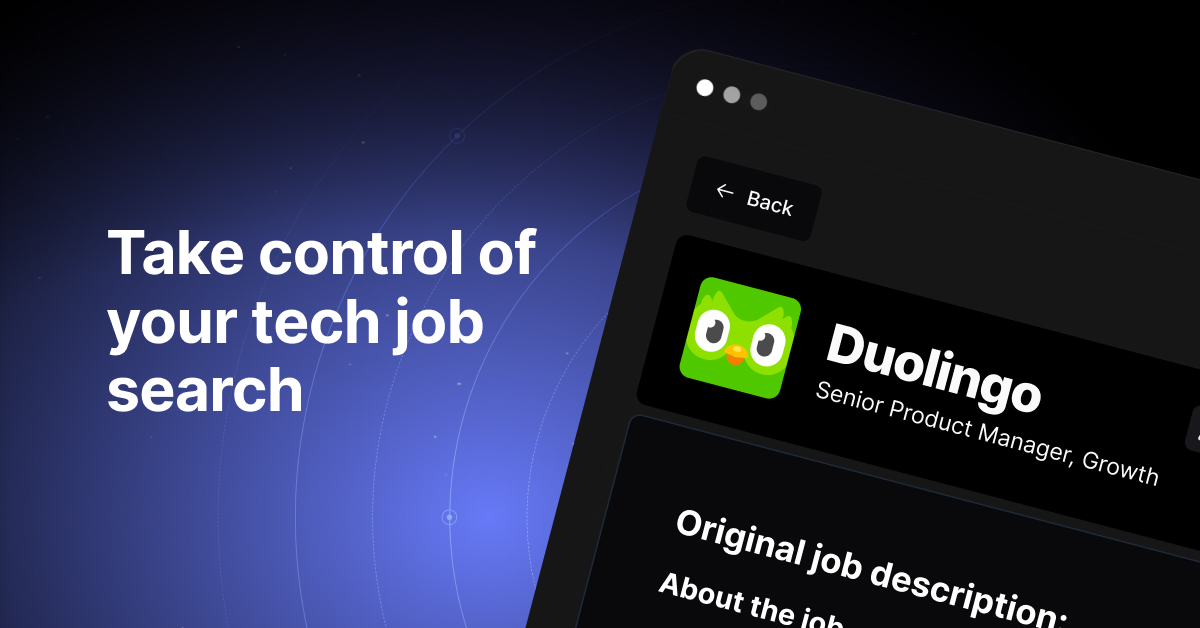