How do I write to the console from a Laravel Controller?
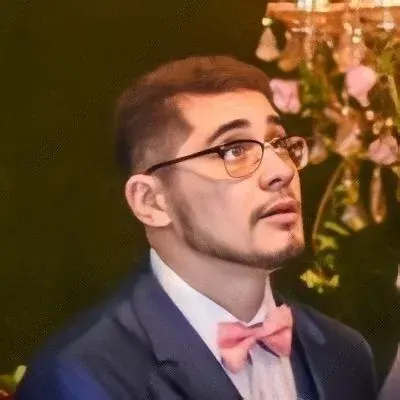
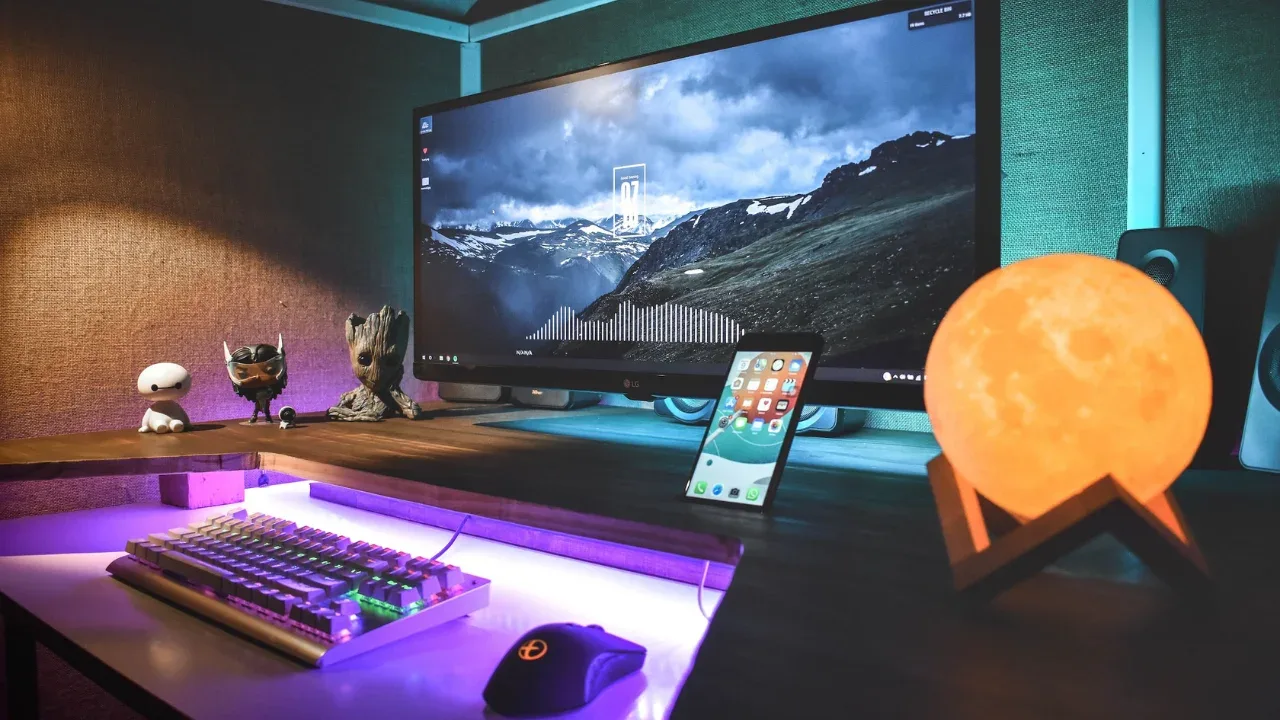
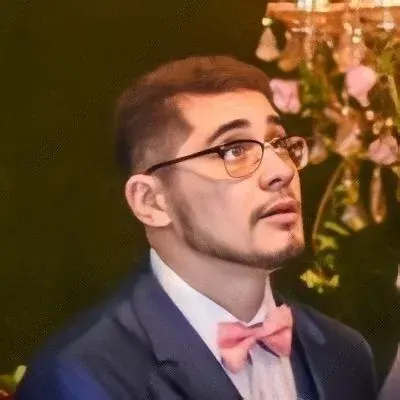
How to Write to the Console from a Laravel Controller
Are you having trouble figuring out how to log console messages from a Laravel controller? Don't worry, we've got you covered! In this guide, we'll walk you through the steps to write to the console with ease. Let's dive right in!
The Problem
So you have a Laravel controller and you want to log messages to the console using the Console
class. However, you're unsure about what to put in the parameter of the Console::info()
method. Let's take a closer look at the code snippet you provided:
class YeahMyController extends BaseController {
public function getSomething() {
Console::info('mymessage'); // <-- what do I put here?
return 'yeahoutputthistotheresponse';
}
}
The Solution
To log console messages from your Laravel controller, you'll need to make use of the stdout
pipe. Don't worry if you're not familiar with this term – we'll explain it in simple terms.
First, make sure you're running your Laravel application using artisan, which runs PHP's built-in development web server under the hood. You can start the server by running the following command in your terminal:
php artisan serve
Once your application is up and running, you can log console messages to the
STDOUT
pipe. This pipe allows you to send output directly to the console. In your controller method, you can use thefwrite()
function to write to theSTDOUT
pipe. Here's an example:public function getSomething() { fwrite(STDOUT, 'mymessage'); return 'yeahoutputthistotheresponse'; }
In the example above, we've replaced the
Console::info()
method with thefwrite()
function and passedSTDOUT
as the first parameter. This will log the message'mymessage'
to the console.
And that's it! You've successfully written to the console from your Laravel controller. Easy, right?
Further Enhancements
If you want to take your console logging to the next level, you can consider the following enhancements:
Logging Levels: Laravel provides various logging levels such as
info
,warning
, anderror
. You can use these levels to differentiate your log messages and provide better insights into your application's behavior.Logging Facade: Instead of directly using the
fwrite()
function, you can make use of Laravel's logging facade –Log
. This facade provides a more expressive and convenient way to log messages. Here's an example:use Illuminate\Support\Facades\Log; public function getSomething() { Log::info('mymessage'); return 'yeahoutputthistotheresponse'; }
By using the
Log
facade, you can take advantage of additional logging features provided by Laravel, such as log channels and log formatting.
Take Action!
Now that you've learned how to write to the console from a Laravel controller, put your knowledge into practice! Try implementing console logging in your own application and see how it can help you debug and monitor your code.
Don't forget to share your experience and any cool tricks you discover along the way. Drop a comment below and let us know how console logging has improved your Laravel development workflow. Happy coding! 🚀🔥💻