How do I generate a custom menu/sub-menu system using wp_get_nav_menu_items in WordPress?
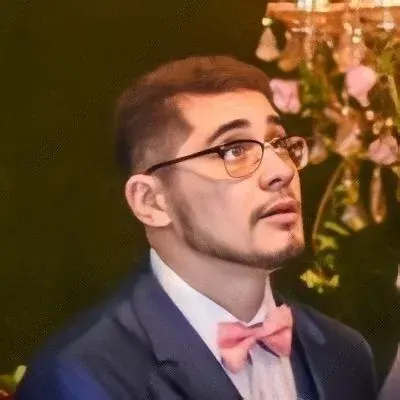
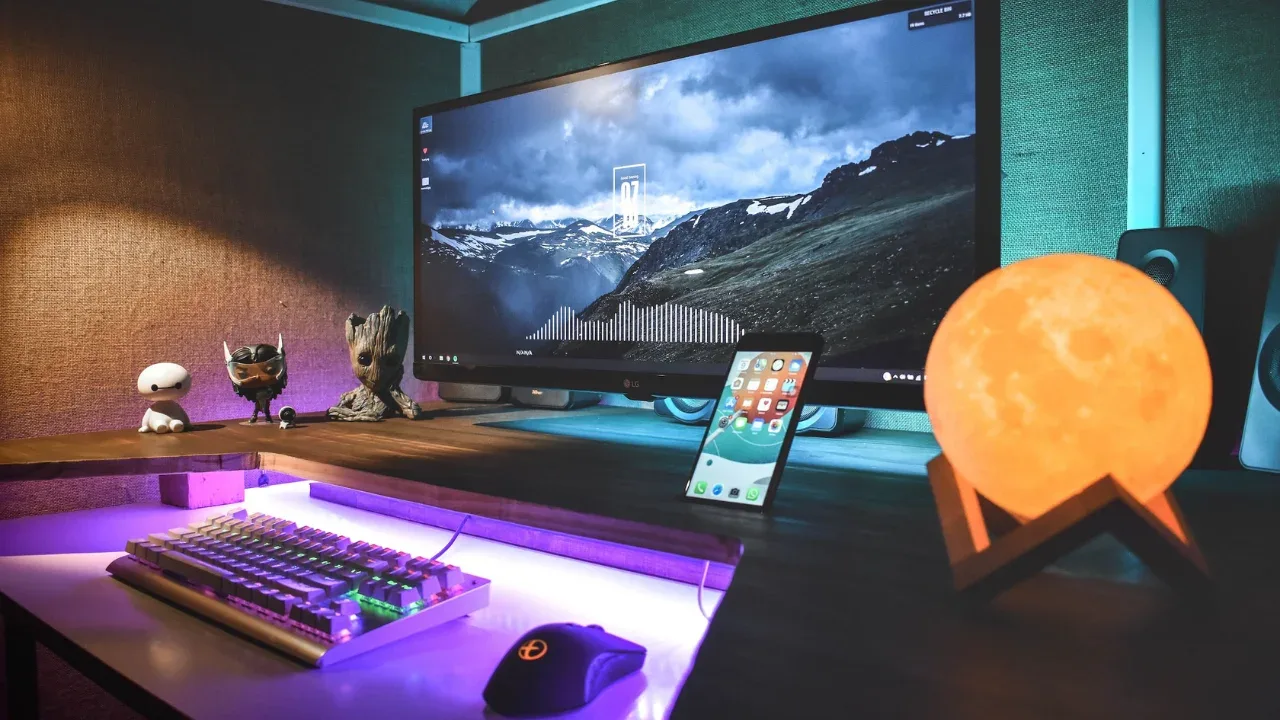
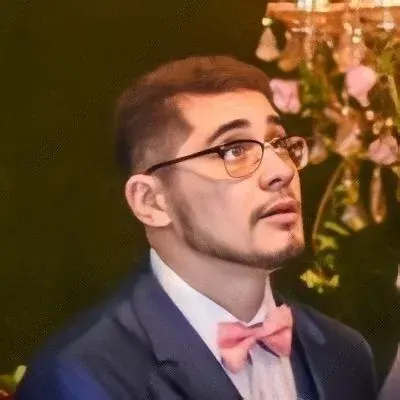
How to Generate a Custom Menu/Sub-Menu System in WordPress using wp_get_nav_menu_items 🍔
Are you looking to create a custom menu/sub-menu system in WordPress but are unsure of how to do it using wp_get_nav_menu_items? Look no further, because we have got you covered! In this guide, we will walk you through the steps to generate a custom menu and sub-menu using wp_get_nav_menu_items.
The Challenge 🎯
You have an HTML structure that requires customization of the wp_nav_menu code. You need to generate a specific HTML structure that includes a main navigation menu and sub-menus. Your current code is successfully generating the main menu items, but you need to integrate the sub-menus as well.
The Solution ✨
To generate the sub-menus in your foreach loop, you can leverage the $item->menu_item_parent property, which gives you the ID of the parent menu item. Here's how you can achieve it:
<?php
$menu_name = 'main-nav';
$locations = get_nav_menu_locations();
$menu = wp_get_nav_menu_object($locations[$menu_name]);
$menuitems = wp_get_nav_menu_items($menu->term_id, array('order' => 'DESC'));
foreach ($menuitems as $item):
$id = get_post_meta($item->ID, '_menu_item_object_id', true);
$page = get_page($id);
$link = get_page_link($id);
?>
<li class="item">
<a href="<?php echo $link; ?>" class="title">
<?php echo $page->post_title; ?>
</a>
<a href="<?php echo $link; ?>" class="desc">
<?php echo $page->post_excerpt; ?>
</a>
<?php
// Check if the current item has sub-menus
if ($item->menu_item_parent != '0'):
// Get the sub-menu items for the current parent item
$sub_menu_items = array_filter($menuitems, function ($submenu_item) use ($item) {
return $submenu_item->menu_item_parent == $item->ID;
});
if (!empty($sub_menu_items)):
?>
<ul class="sub-menu">
<?php
// Loop through the sub-menu items and generate the HTML structure
foreach ($sub_menu_items as $sub_menu_item):
$sub_menu_id = get_post_meta($sub_menu_item->ID, '_menu_item_object_id', true);
$sub_menu_page = get_page($sub_menu_id);
$sub_menu_link = get_page_link($sub_menu_id);
?>
<li class="item">
<a href="<?php echo $sub_menu_link; ?>" class="title">
<?php echo $sub_menu_page->post_title; ?>
</a>
<a href="<?php echo $sub_menu_link; ?>" class="desc">
<?php echo $sub_menu_page->post_excerpt; ?>
</a>
</li>
<?php endforeach; ?>
</ul>
<?php endif; ?>
<?php endif; ?>
</li>
<?php endforeach; ?>
In this code snippet, we have added an if condition inside the foreach loop to check if the current item has sub-menus. If it does, we retrieve the sub-menu items by filtering the $menuitems array based on the $item->ID.
Finally, we loop through the sub-menu items and generate the HTML structure required.
Simplicity with wp_nav_menu? 🤔
While using wp_get_nav_menu_items with customized code provides the flexibility you need, you might be wondering if there is a simpler way using wp_nav_menu. Unfortunately, wp_nav_menu does not directly provide the ability to customize the sub-menu HTML structure. Therefore, using wp_get_nav_menu_items is the recommended approach for generating a custom menu/sub-menu system.
Conclusion 🌟
Generating a custom menu/sub-menu system using wp_get_nav_menu_items in WordPress can seem daunting at first, but with the right approach, it becomes a breeze. By leveraging the $item->menu_item_parent property and a foreach loop, you can easily generate the desired HTML structure for your menu and sub-menu.
Remember to customize the code according to your specific requirements and HTML structure. Enjoy creating awesome custom menus in WordPress!
Got any questions or facing any issues? We're here to help! Drop a comment below and let's get the conversation started. 💬