How do I check if a string contains a specific word?
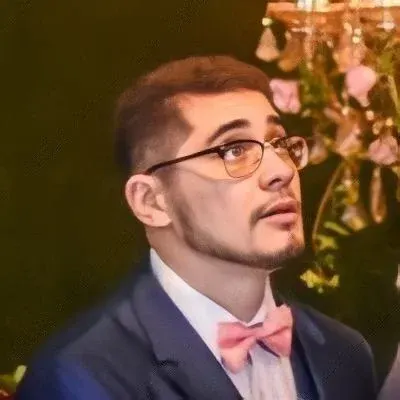

📝 The String Contain Dilemma: How to Check if a String Contains a Specific Word?
Ever found yourself pondering over the perfect syntax for checking if a string contains a specific word? 🤔 Don't worry; you're not alone! Whether you're a seasoned programmer or just starting out, handling strings can be a tricky task. But fear not! In this guide, we'll dive into common issues, provide easy solutions, and empower you to conquer this string conundrum. Let's get started! 💪
💡 Understanding the Problem
Before we delve into solutions, let's break down the problem at hand. In the given context, the code attempts to determine if the string $a
contains the word "are". However, searching for substrings within a string isn't as straightforward as it may seem. So how do we tackle this challenge? Let's explore some possible solutions.
🛠️ Solution 1: Using strpos()
Function
A popular approach to this problem involves using the strpos()
function, which stands for "string position". This function helps us find the first occurrence of a substring within a string. To check if a string contains a specific word, you can use code similar to this:
$a = 'How are you?';
if (strpos($a, 'are') !== false) {
echo 'true';
}
In the above code snippet, strpos($a, 'are')
returns the index of the first occurrence of 'are' within the string $a
. If the substring is found, strpos()
provides a non-negative integer value. On the other hand, if the substring is not found, it returns false
.
Note: We use
!== false
instead of== true
in the condition becausestrpos()
can return0
if the substring is found at the start of the string. In PHP,0
is considered as falsy, so checking with== true
could lead to incorrect results.
🛠️ Solution 2: Utilizing Regular Expressions
Another powerful technique for checking if a string contains a specific word involves utilizing regular expressions. Regular expressions (or regex) are powerful tools that allow for pattern matching within strings.
To employ regex in PHP, we can use the preg_match()
function. This function searches a string for a pattern and returns true if a match is found. Here's an example of using preg_match()
to check for the word "are" in the string variable $a
:
$a = 'How are you?';
if (preg_match('/are/', $a)) {
echo 'true';
}
In the code snippet above, the regular expression /are/
is passed as the pattern to preg_match()
. The function searches for this pattern in the string $a
. If a match is found, it returns true
. Remember, regex offers powerful capabilities for more complex matching patterns beyond simple words.
❗ Common Pitfalls and Tips
While we've discussed the two primary solutions, let's highlight some common pitfalls and tips to keep in mind when checking if a string contains a specific word:
Case Sensitivity: Both
strpos()
andpreg_match()
are case-sensitive by default. If you need a case-insensitive search, you can modify the regular expression or convert the string and substring to lowercase before comparison.Whitespace Concerns: Both functions are whitespace-sensitive. So, if a string contains leading or trailing whitespaces, the function may not detect a match. Consider using
trim()
to remove surrounding whitespaces before performing the search.Multiple Occurrences: If you're interested in finding multiple occurrences of a word within a string, you can iterate over the string or utilize appropriate functions like
preg_match_all()
.
📣 A Call to Action: Let's Level Up Your String Game!
Congratulations, string searcher! You've now mastered the art of checking if a string contains a specific word. 🎉 But why stop here? Strings are powerful tools, and understanding how to manipulate them opens up a world of possibilities in your coding journey. So go ahead, experiment with different scenarios, and level up your string game!
Share your thoughts and experiences in the comments below. How did you overcome the string searching challenge? Have any additional tips or tricks to offer? Let's continue this string seeking adventure together! 🔎💬
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
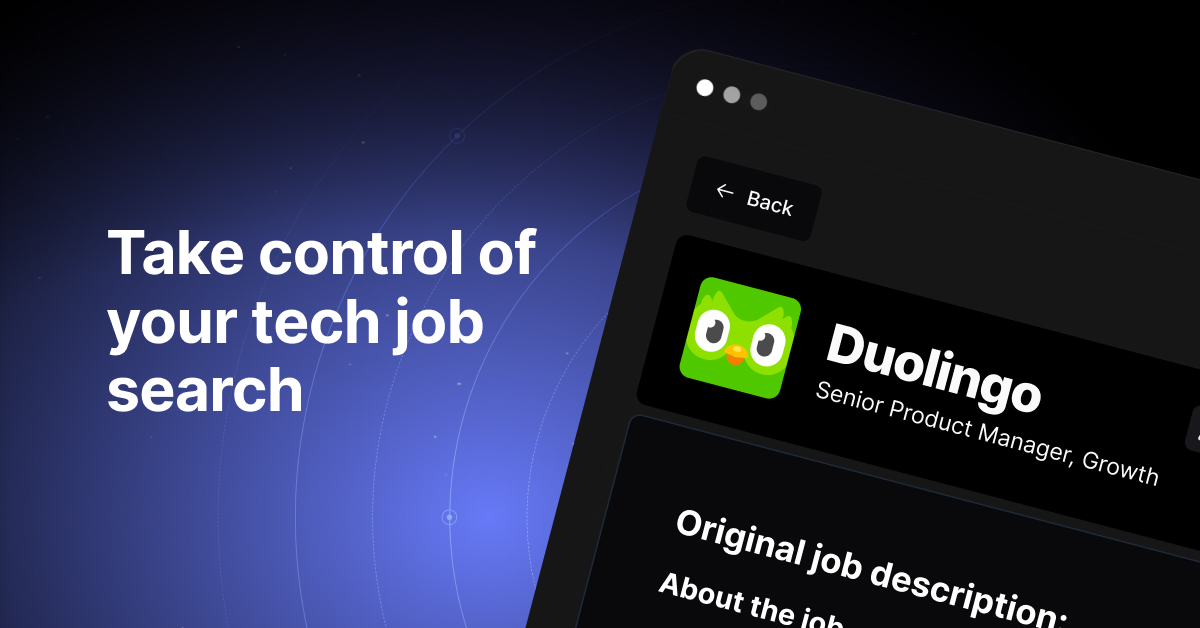