How can I remove three characters at the end of a string in PHP?
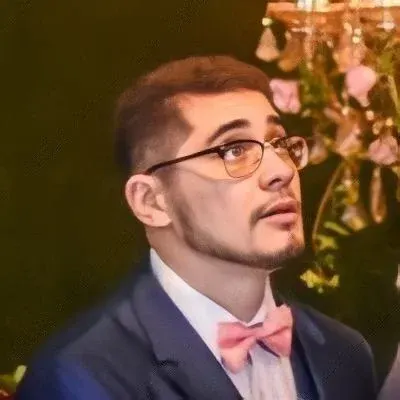
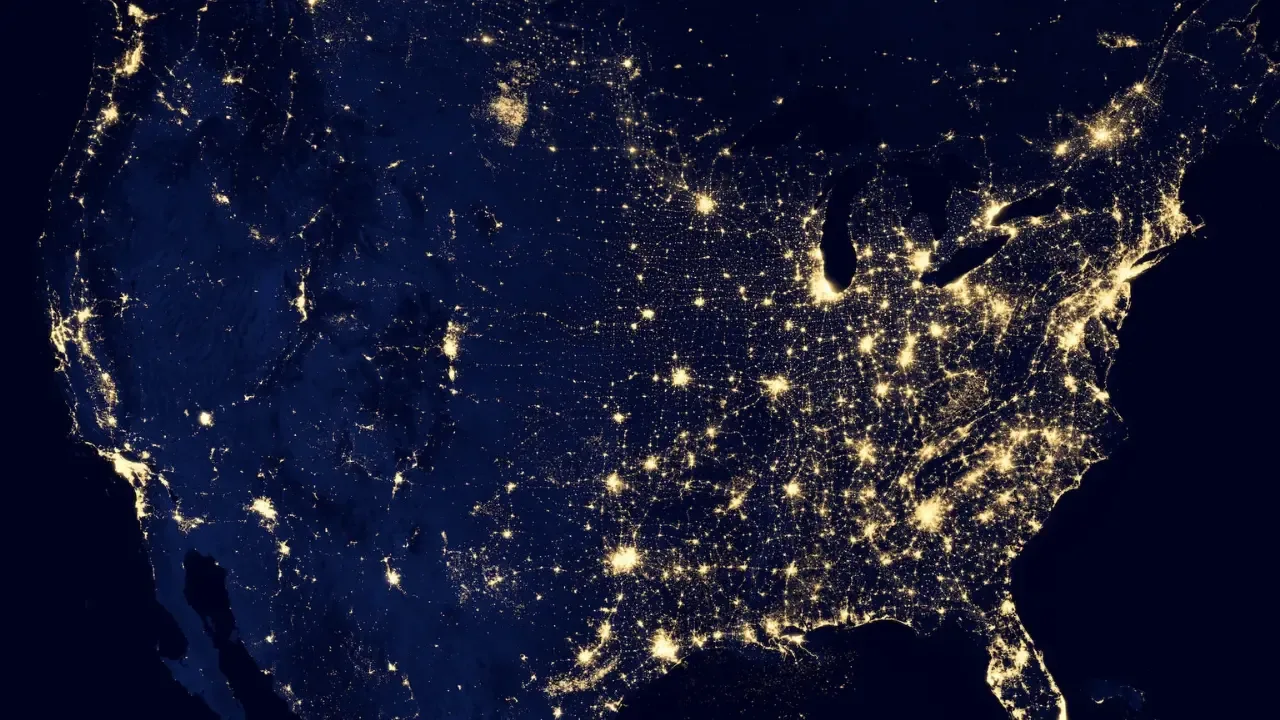
How to Remove Three Characters at the End of a String in PHP 😎💪
Picture this: you have a string that just won't cut it and you need to trim off the last three characters. 🚀 No worries! In PHP, there are several simple and efficient ways to accomplish this task. Let's dive right in! 👇
The Substr Method ✂️
One popular method for removing characters at the end of a string is using the substr
function. This function allows us to extract a portion of a string based on start and length values. In our case, we can specify a start value of 0 and a length value that is the length of the original string minus three. Here's how it looks in code:
$originalString = "abcabcabc";
$trimmedString = substr($originalString, 0, -3);
echo $trimmedString; // Output: "abcabc"
Voilà 🎉! You now have a $trimmedString
variable that contains the original string without the last three characters.
Removing Characters with rtrim 🔥
Another handy function we can utilize is rtrim
, which specifically removes characters from the right side of a string. By passing in the characters we want to remove as the second parameter, we can achieve the desired outcome. Here's an example:
$originalString = "abcabcabc";
$trimmedString = rtrim($originalString, "abc");
echo $trimmedString; // Output: "abcabc"
Amazing 🙌! The rtrim
function works by removing all occurrences of the specified characters from the right side of the string until it reaches a character that does not match the provided list.
Using a Regular Expression with preg_replace 🎭
For those who enjoy the flexibility of regular expressions, we can take advantage of the preg_replace
function to remove the last three characters. Here's how it works:
$originalString = "abcabcabc";
$trimmedString = preg_replace("/.{3}$/", "", $originalString);
echo $trimmedString; // Output: "abcabc"
By using the regular expression /.{3}$/
, we are capturing the last three characters of the string and replacing them with an empty string.
Time to Trim and Shine! ✨
Now that you've learned three different ways to remove three characters from the end of a string in PHP, you can confidently tackle any string-trimming challenge! Whether you prefer using substr
, rtrim
, or regular expressions with preg_replace
, you now have a range of techniques at your disposal. 🤓
If you have any questions, suggestions, or other creative approaches, feel free to share them in the comments below. Let's keep the discussion going and help one another become PHP string manipulation masters! 💪💻
Happy coding! 🎉
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
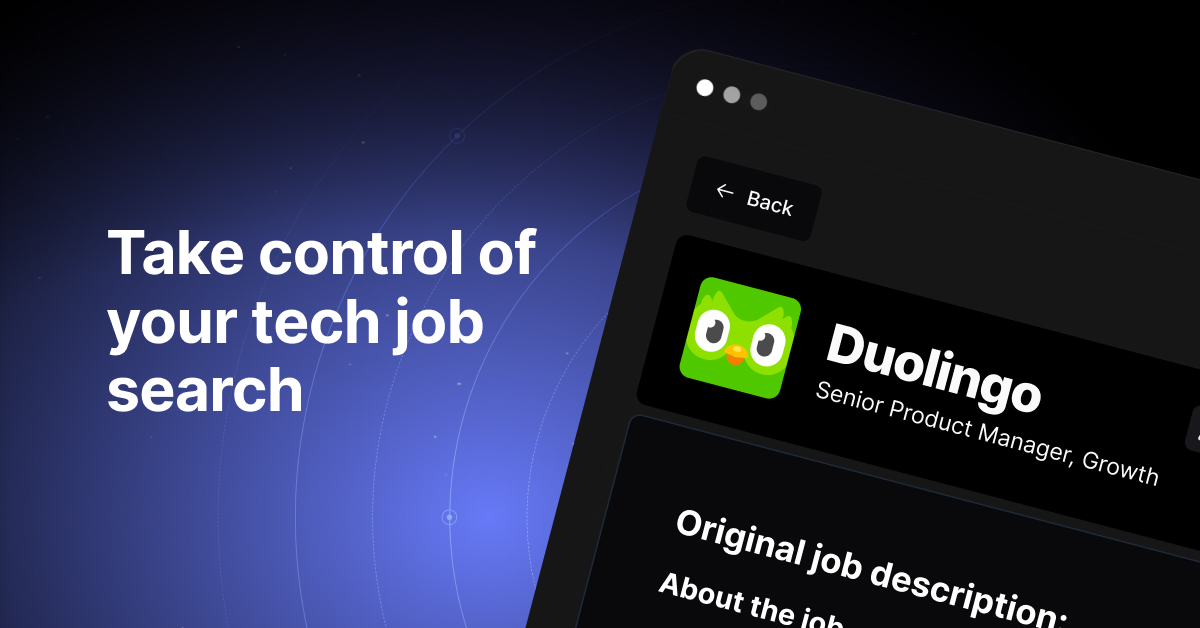