How can I manually return or throw a validation error/exception in Laravel?
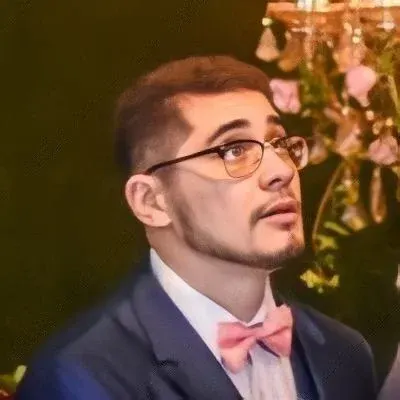
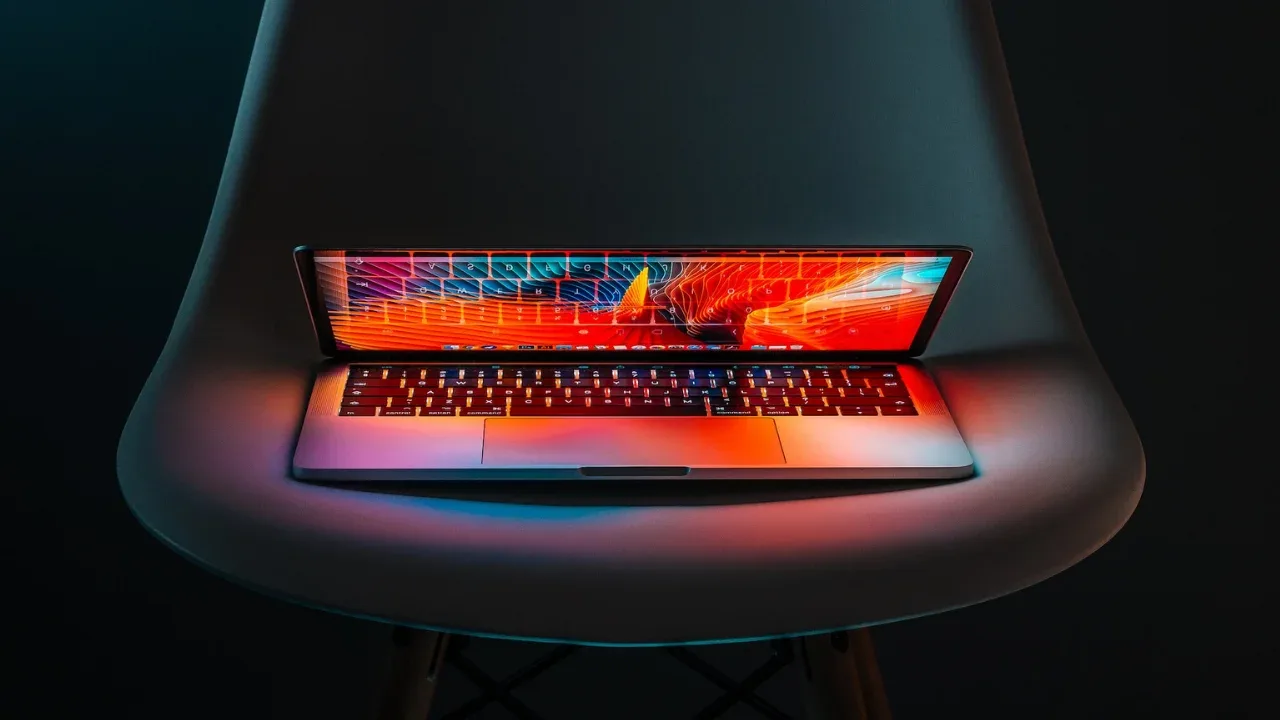
How to Manually Return or Throw a Validation Error/Exception in Laravel 📝🔥
So, you're working on a Laravel project and you have this cool method that imports CSV data into your database 📊. You've got some basic validation in place using the validate
method provided by Laravel, and it works like a charm 👌. But then, as you go deeper into the rabbit hole of complexity, you encounter situations when exceptions are thrown for more intricate reasons 😱.
You might be thinking, "Gosh, I really love how Laravel handles validation errors and easily embeds them into blade views 🎨, but I can't use the validate
method here in this complex scenario. Is there a way to manually tell Laravel that I messed up validation and I want it to handle the error gracefully just like it does with the validate
method? 🤔"
Fear not, my friend! Laravel provides a solution to this dilemma 🙌. You can manually return or throw a validation error/exception and make Laravel work its magic as if you had used the validate
method 💫. Let's explore a clean way to achieve this.
Return a Validation Error Response
Laravel allows you to conveniently return a JSON response containing validation errors. Here's how you can accomplish this:
use Illuminate\Support\Facades\Validator; use Illuminate\Http\JsonResponse; try { // Your complex import logic goes here } catch (\Exception $e) { $validator = Validator::make([], []); $validator->getMessageBag()->add('fieldname', 'Your detailed error message'); // Return the validation error response throw new \Illuminate\Validation\ValidationException($validator); }
In this example, we create an empty
Validator
instance and add our custom error message to the validation error bag. Then, we throw aValidationException
with theValidator
instance. Laravel will take care of transforming this into a proper JSON response 📤, so you can handle it gracefully in your front-end application.Render a Blade View with Validation Errors
If you prefer to render a Blade view with validation errors, Laravel can handle that too! Let's see how to achieve it:
use Illuminate\Support\Facades\Validator; use Illuminate\Support\Facades\View; try { // Your complex import logic goes here } catch (\Exception $e) { $validator = Validator::make([], []); $validator->getMessageBag()->add('fieldname', 'Your detailed error message'); // Render the view with the validation errors return View::make('error.view')->withErrors($validator); }
Here, we create a new
Validator
instance and add our custom error message to the validation error bag. We then pass theValidator
to thewithErrors
method of theView
class. This will make Laravel bind the errors to the view, allowing you to display them using Laravel's awesome blade templating engine 🎭.Handle the Validation Error Response/View
Now that you've mastered the art of returning or throwing a validation error/exception, how do you handle it on the front end? Here's where your creativity comes in! You can use JavaScript to handle the JSON response and display it to the user in a graceful manner. Or, if you're using the Blade view approach, just make sure to include the necessary error display logic in your template.
So go on, brave Laravel developer! 🔥 Utilize these techniques to manually return or throw a validation error/exception. Embrace the power of Laravel's error handling capabilities, even in those complex scenarios where the validate
method can't be used.
Remember to always let your code shine and your users stay happy 😄💻.
Got any questions or cool approaches to share? Drop a comment below and let's keep the conversation going! 🗣️💬
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
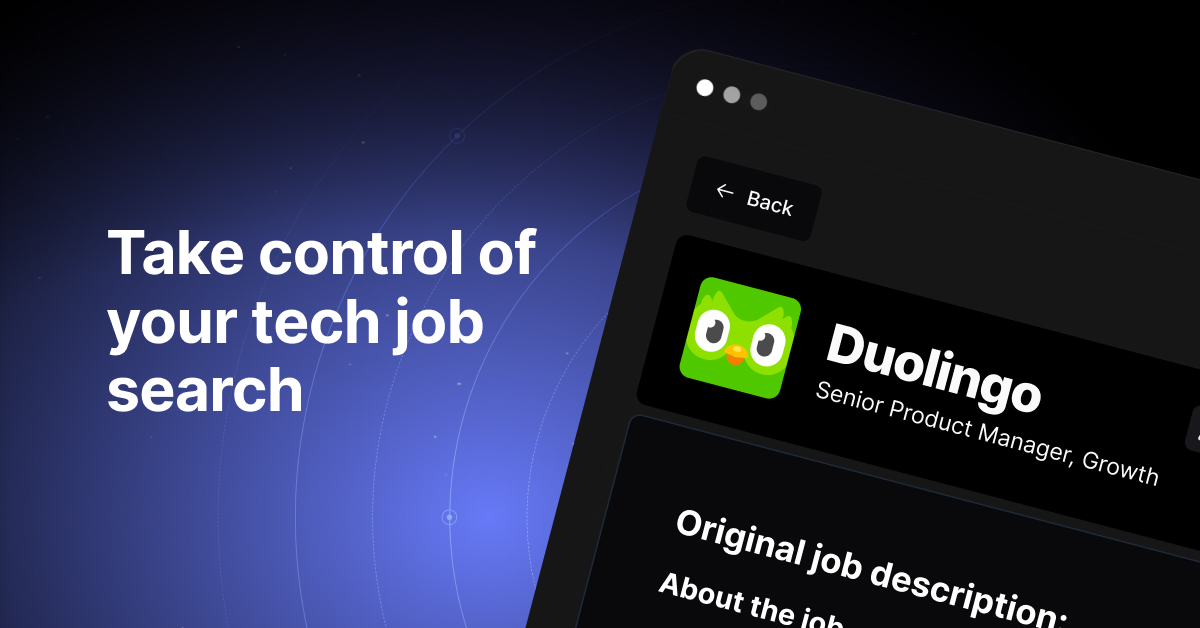