How can I get the last 7 characters of a PHP string?
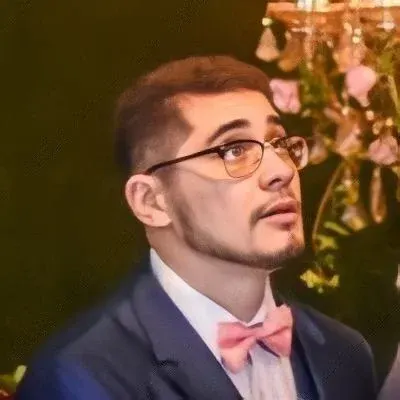
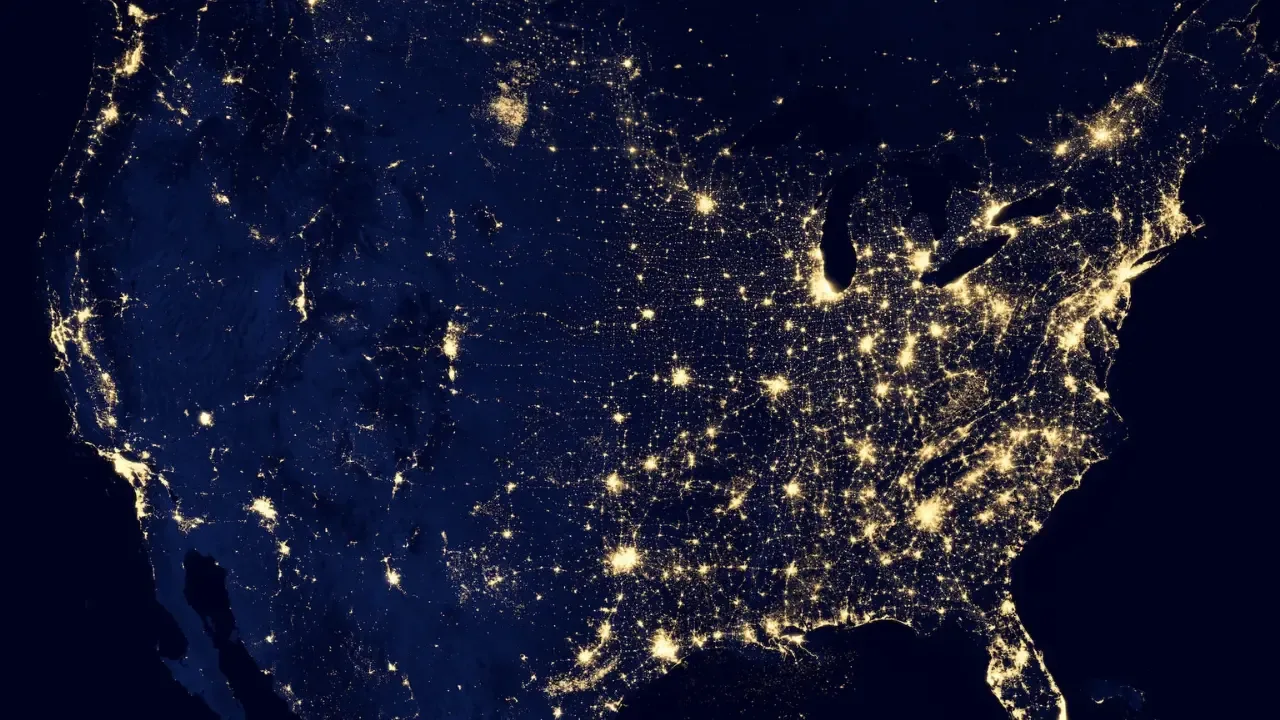
Grabbing the Last 7 Characters of a PHP String: A Simple Guide 🎯
Are you struggling to get the last 7 characters of a PHP string? Don't worry, we've got you covered! In this blog post, we'll walk you through common issues and provide easy solutions using PHP functions. By the end, you'll be a pro at extracting the last seven characters! Let's dive in! 🚀
The Problem: Grabbing the Last 7 Characters
So, you have a dynamic string, and you want to extract only the last 7 characters. In the example provided, $dynamicstring
holds the original string 2490slkj409slk5409els
, and you're aiming to get 5409els
. Sounds simple enough, right? 😄
The Solution: Using PHP String Functions
Fortunately, PHP offers a set of powerful built-in string functions that make our lives easier. Let's look at two popular methods to extract the last 7 characters from a string.
1. Using substr() Function
The substr($string, $start, $length)
function is your go-to for extracting substrings in PHP. To get the last 7 characters of a string, you need to specify the starting position and the length of the substring desired.
$dynamicstring = "2490slkj409slk5409els";
$newstring = substr($dynamicstring, -7); // starting from the 7th character from the end
echo "The new string is: " . $newstring;
The output will be:
The new string is: 5409els
By passing a negative value as the starting position, substr()
cleverly counts from the end of the string. You don't need to calculate the starting position manually!
2. Using mb_substr() Function
Sometimes, our strings might contain multi-byte characters such as emojis or non-ASCII characters. In such cases, we need to rely on the mb_substr($string, $start, $length, 'utf-8')
function. This function works similarly to substr()
, but with added support for multi-byte characters.
$dynamicstring = "2490slkj409slk5409els";
$newstring = mb_substr($dynamicstring, -7, null, 'utf-8'); // null length parameter to extract till the end
echo "The new string is: " . $newstring;
The output will be the same:
The new string is: 5409els
By passing null
as the length parameter, mb_substr()
extracts the substring from the starting position until the end of the string, regardless of its length.
Tested & Verified!
Now you know how to grab the last 7 characters of a PHP string using either substr()
or mb_substr()
functions. Feel free to try both methods and use the one that suits your needs best. 💪
Call to Action: Share Your Experience!
We hope this guide helped you effortlessly solve the problem of extracting the last 7 characters from a PHP string. 💡
But wait! We'd love to hear from you too! Have you encountered any other string-related challenges in PHP? Share your experience and let's discuss them in the comments section below. 🗣️💬
Happy coding! 🎉
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
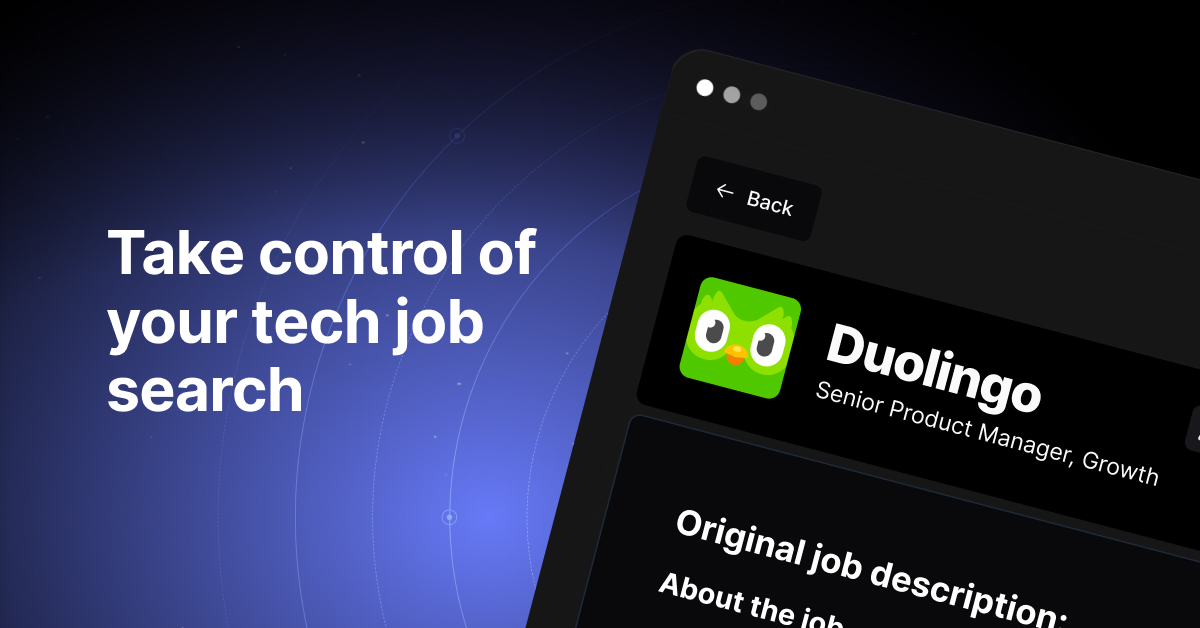