How can I capture the result of var_dump to a string?
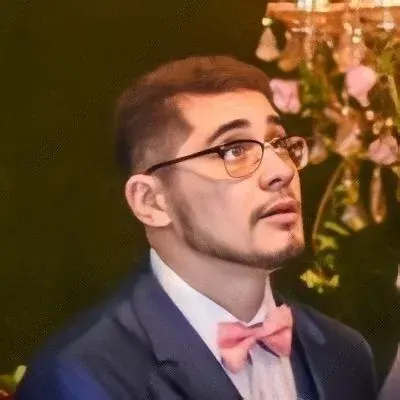
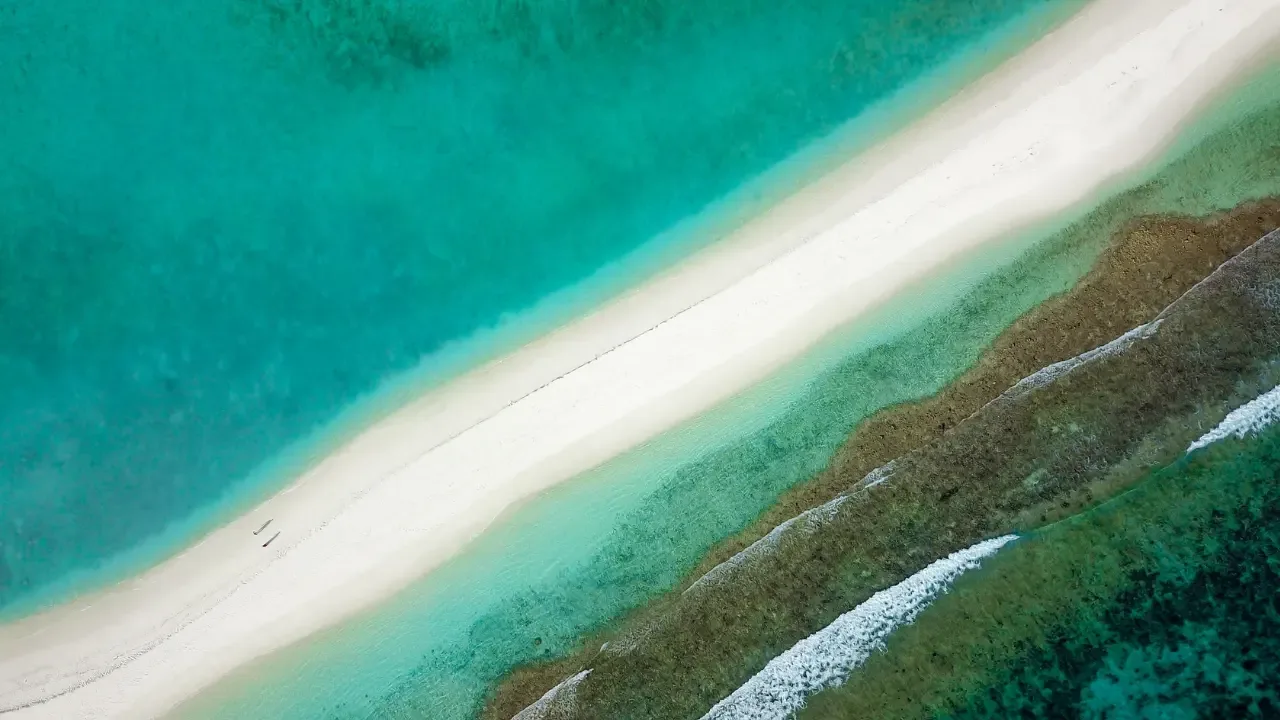
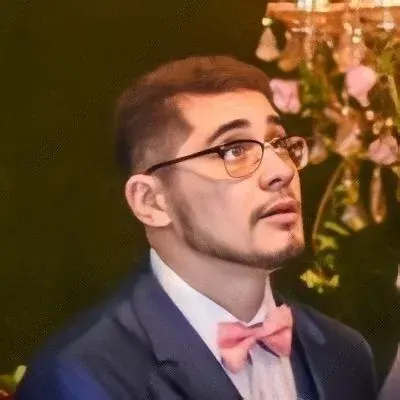
How to Capture the Result of var_dump to a String 📊📝💡
So you want to capture the output of var_dump
in PHP and save it as a string? Look no further! In this blog post, we will explore common issues you may encounter and provide easy solutions to help you achieve your goal.
The Challenge 🧩
Capturing the output of var_dump
to a string can be tricky, as the function directly outputs the data to the browser. However, the PHP documentation suggests using the output-control functions to overcome this hurdle.
Solution 1: Using Output Buffering 📦
Output buffering is a technique used to capture and manipulate the output of PHP code. It allows you to store the output in memory before sending it to the browser. Here's an example of how you can use output buffering to capture the result of var_dump
:
ob_start(); // Start output buffering
var_dump($yourVariable); // Output the variable using var_dump
$result = ob_get_clean(); // Capture the output and assign it to a variable
In the code snippet above, we start the output buffering using ob_start()
. Then, we perform var_dump
on your desired variable. Finally, we use ob_get_clean()
to capture the output and assign it to the $result
variable.
Solution 2: Using ob_get_contents() 📤
Another approach to capturing the result of var_dump
is by using the ob_get_contents()
function. This function returns the contents of the output buffer without clearing it. Here's how you can use it:
ob_start();
var_dump($yourVariable);
$result = ob_get_contents();
ob_end_clean();
In this solution, we start the output buffering, perform the var_dump
, and then use ob_get_contents()
to get the output. To ensure proper cleanup, we call ob_end_clean()
to discard the buffer.
Solution 3: Custom Function 🛠️
If you find yourself frequently needing to capture var_dump
output, you can create a custom function to simplify the process. Here's an example:
function capture_var_dump($variable) {
ob_start();
var_dump($variable);
return ob_get_clean();
}
// Usage:
$result = capture_var_dump($yourVariable);
By encapsulating the code within a function, you can easily call it whenever you need to capture the result of var_dump
without duplicating the code.
Take It Up a Notch! 🔥
Now that you know how to capture the result of var_dump
to a string, why not level up your debugging game? 🚀
Consider integrating a debugging tool like Xdebug into your development environment. Xdebug offers advanced features, such as stack traces, variable tracking, and profiling, making it easier to identify and fix issues in your code.
Conclusion 🏁
Capturing the result of var_dump
to a string may be a challenge, but with the help of output buffering or a custom function, you can accomplish it easily. Remember to choose the approach that best suits your needs.
Do you have any other PHP-related questions or suggestions? Let us know in the comments below! 👇🤔
Happy coding! 💻😄