Get the Query Executed in Laravel 3/4
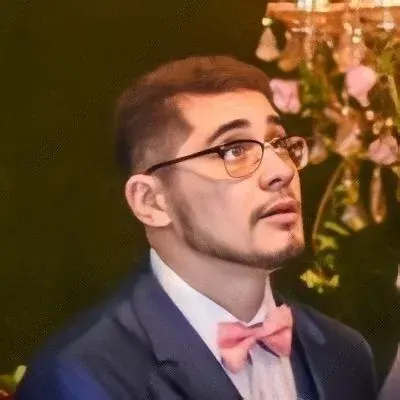
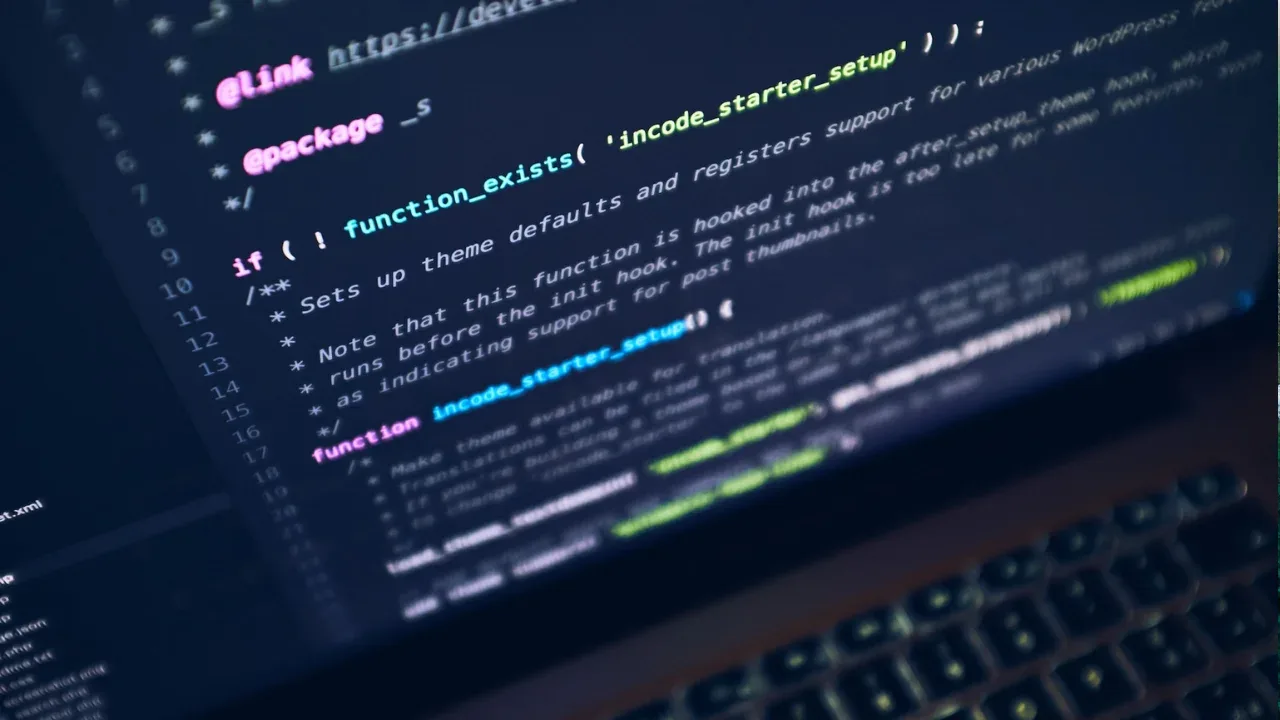
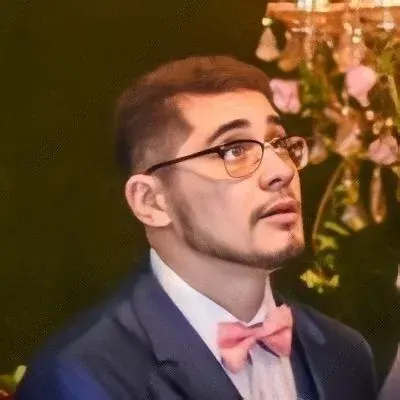
🌟 Get the Query Executed in Laravel 3/4 🌟
Are you working with Laravel 3/4 and finding it challenging to retrieve the executed SQL query? Don't worry, my friend! I've got your back. In this blog post, we'll tackle this common issue and provide you with easy solutions. Let's dive in! 💪
⚡️ Solution 1: Using Laravel Query Builder ⚡️
To retrieve the raw executed SQL query using Laravel Query Builder in Laravel 3/4, follow these steps:
Step 1️⃣: Open your code editor and locate the part where you're executing the query. It might look something like this:
$query = DB::table('users')->where_status(1)->get();
Step 2️⃣: To get the raw executed SQL query, add the to_sql()
method before executing the query. Your code will look like this:
$query = DB::table('users')->where_status(1)->to_sql();
$results = $query->get();
Step 3️⃣: By calling the to_sql()
method, you'll retrieve the raw SQL query. You can now use $query
to access the query itself, or $results
to access the query results.
⚡️ Solution 2: Using Eloquent ORM ⚡️
If you're using the Eloquent ORM in Laravel 3/4, retrieving the executed SQL query is just as simple. Follow these steps:
Step 1️⃣: Locate the part of your code where you're using Eloquent to execute the query. It might look like this:
$posts = User::find(1)->posts->get();
Step 2️⃣: To get the raw executed SQL query, you can use the toSql()
method. Modify your code like this:
$posts = User::find(1)->posts->toSql();
$results = User::find(1)->posts->get();
Step 3️⃣: By using the toSql()
method, you can access the raw SQL query through $posts
. Similarly, you can use $results
to access the query results.
📝 Bonus Tip: Save queries to laravel.log 📝
If all you need is to save all executed queries to laravel.log
, Laravel provides an in-built solution for that. Open your .env
file and make sure the following line is set to true
:
DB_LOG_QUERIES=true
🔍 This will automatically log all queries executed in your Laravel 3/4 application.
Now you know how to retrieve the executed SQL query in Laravel 3/4 using Laravel Query Builder or Eloquent ORM. Give these solutions a try and simplify your debugging process! 💡
If you found this blog post helpful, don't forget to share it with your developer friends. And if you have any questions or want to share your experiences, leave a comment below. Happy coding! 🚀