Get Specific Columns Using βWith()β Function in Laravel Eloquent
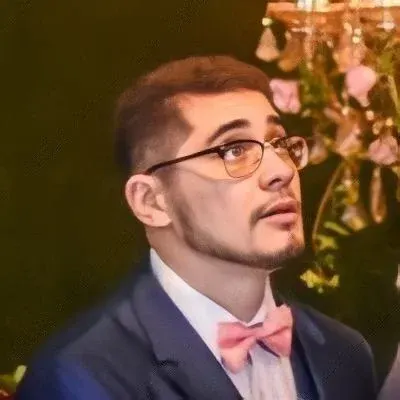
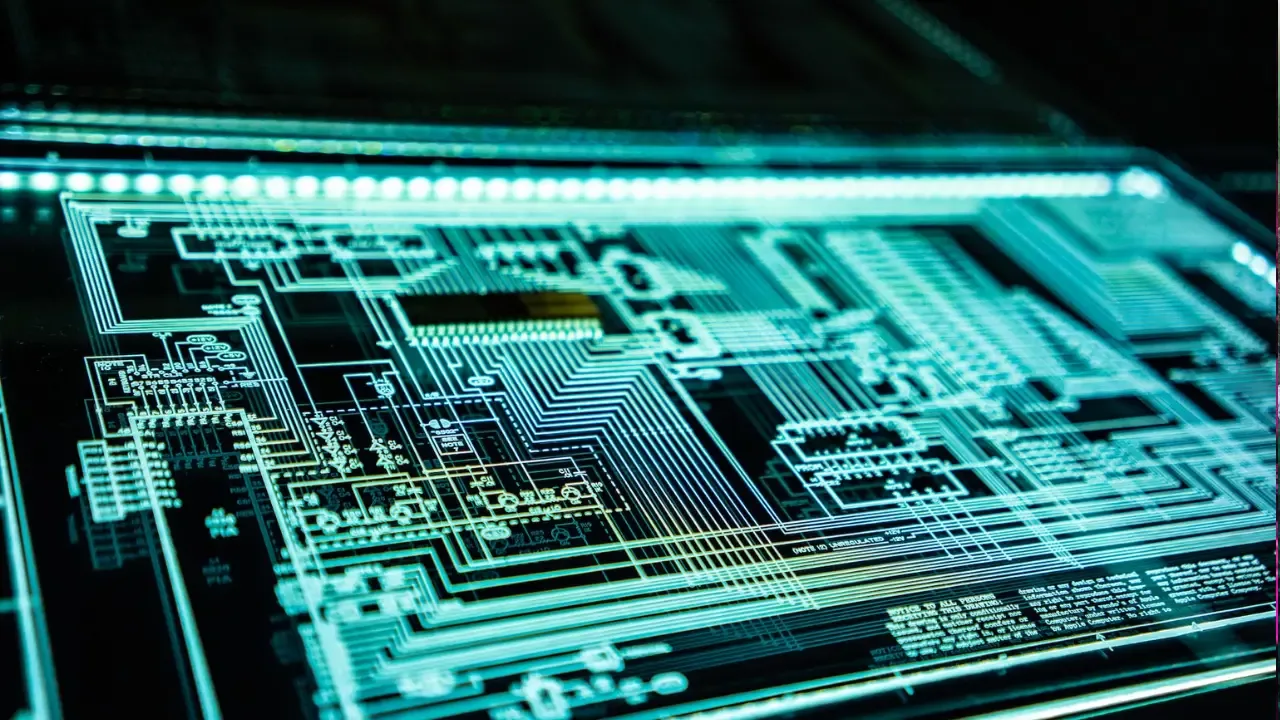
π Get Specific Columns Using "With()" Function in Laravel Eloquent π
If you're working with Laravel Eloquent and you want to join two tables using the with()
function, but only retrieve specific columns from the second table, you might be facing a common challenge. In this blog post, I'll show you an easy solution to this problem.
The Background Story
Let's set the stage and understand the scenario. You have two tables: User
and Post
. Each user can have multiple posts, and each post belongs to only one user. The relationship is defined in the models accordingly.
// User model
public function posts()
{
return $this->hasMany(Post::class);
}
// Post model
public function user()
{
return $this->belongsTo(User::class);
}
The Challenge
You want to retrieve all posts and also include the user information associated with each post. However, you only need specific columns from the users
table, not all of them. Using the with()
function in Laravel Eloquent is a great approach for achieving this, but it's not immediately obvious how to specify the desired columns from the second table.
The Solution
To get specific columns from the second table while using the with()
function, you'll need to use the select()
method on the eager loaded relation. This allows you to define the columns you want to retrieve from the associated table.
Here's an example that demonstrates how to achieve this:
public function getAllPosts()
{
return Post::with(['user' => function ($query) {
$query->select('id', 'username');
}])->get();
}
By using a closure in the with()
method and calling the select()
method within it, you can specify the desired columns (id
and username
) from the users
table.
Let's Break It Down
In the with()
method, we pass an array where the key represents the relationship name (user
in our case), and the value is a closure that allows us to customize the query for the relation. This closure receives a $query
parameter, which is an instance of the underlying query builder.
Inside the closure, we call the select()
method on the $query
instance and pass the columns we want to retrieve (id
and username
in our example).
When you execute getAllPosts()
, Laravel will perform the following queries:
select * from `posts`
select `id`, `username` from `users` where `users`.`id` in (...user ids...)
This way, you'll get the desired posts and only the specified columns from the users
table associated with each post.
Conclusion
Using the with()
function in Laravel Eloquent allows you to efficiently retrieve related data, and now you know how to specify specific columns from the associated table. By using the select()
method within a closure, you can customize the columns retrieved from the second table with ease.
So go ahead, utilize this technique in your Laravel projects and level up your querying skills! If you have any further questions or need assistance, drop a comment below, and let's keep the conversation going.
Happy coding! π»β¨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
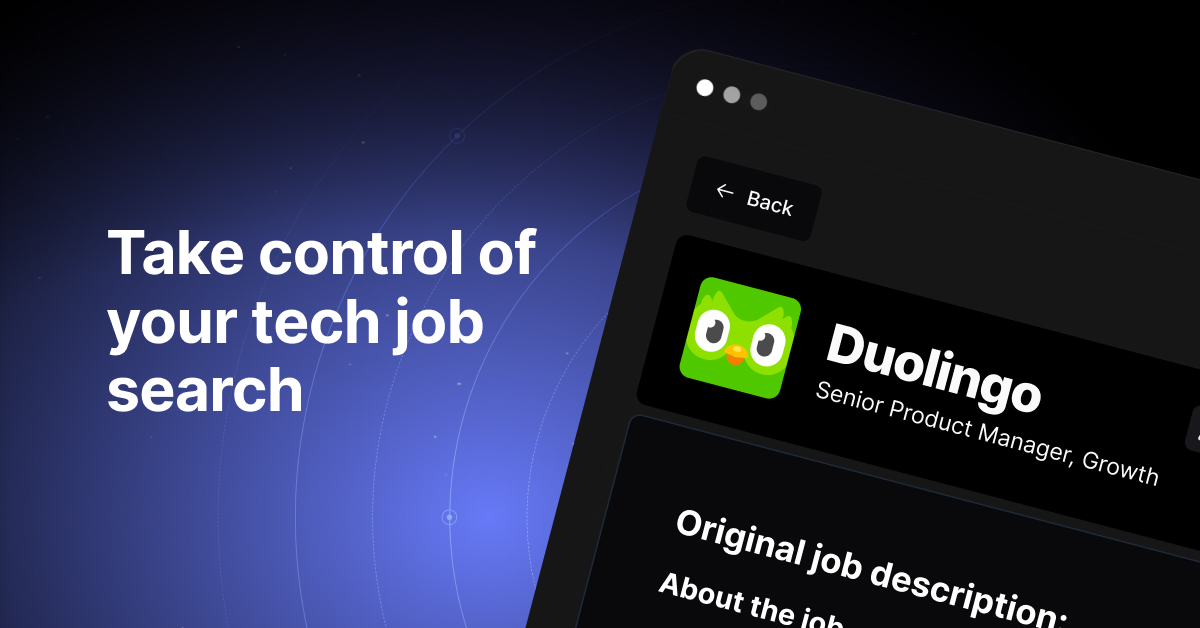