Get only specific attributes with from Laravel Collection
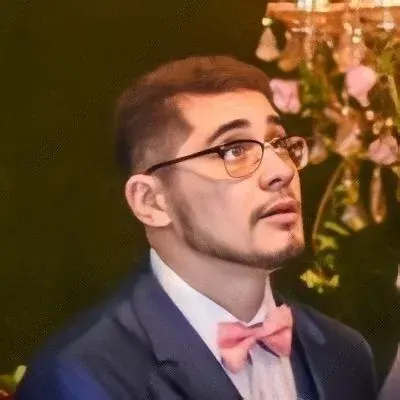
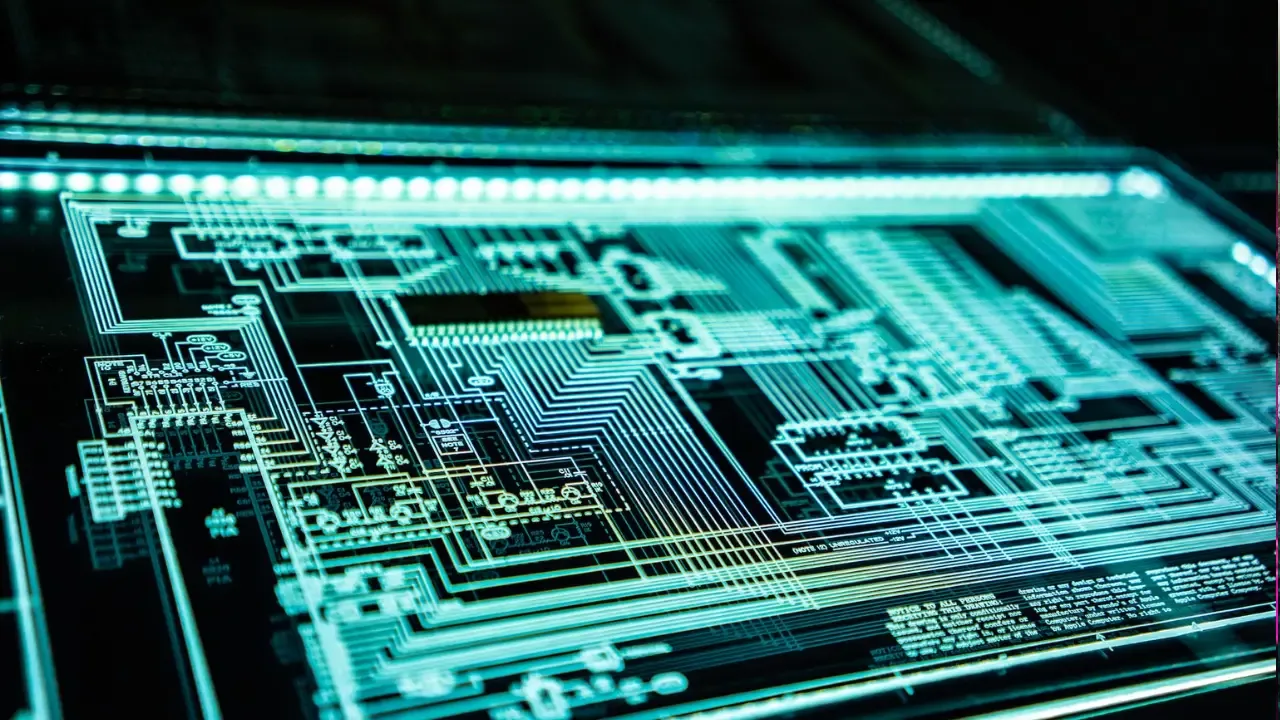
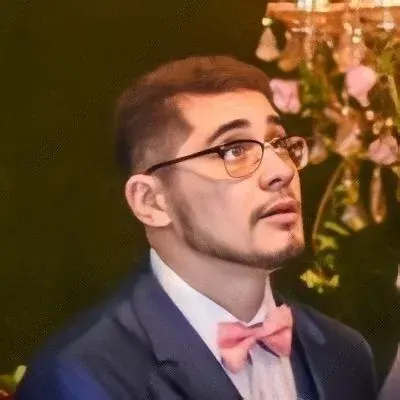
📢 Hey there, Laravel enthusiasts! Are you struggling to retrieve specific attributes from a Laravel Collection? Don't fret, I've got you covered! 🤩💪
So, you want to fetch an array with model data from a collection, but only extract the attributes that you need. It seems there's no built-in helper for this in Laravel, but fear not, I'll show you a couple of easy-peasy solutions. Let's dive right in! 🏊♀️🏊♂️
Solution 1: The pluck() Method
One elegant way to snatch those specific attributes is by using the pluck()
method. 🍴 Here's an example on how to do it:
$userData = Users::pluck('name', 'email');
In this example, pluck()
will create an array with the 'name' attribute values as keys and the 'email' attribute values as values. 🗝️ Just what you asked for!
Solution 2: The map() Method
Another approach involves using the map()
method, which allows you to manipulate each item in the collection. Here's an example of how to use it:
$userData = Users::map(function ($user) {
return [
'name' => $user->name,
'email' => $user->email,
];
});
With map()
, you can customize the specific attributes you want to include in your final array. You just need to define the attributes inside the callback function and return them accordingly. 😎✍️
Take it a Step Further ⏩
Now you know how to fetch specific attributes from a Laravel Collection, but there's so much more you can do with collections! Why not explore other fantastic methods like filter()
, sortBy()
, or transform()
? These are powerful tools that can help you manipulate your data in myriad ways. 💪🌟
🔎 If you want to learn more about Laravel Collections and how to harness their full potential, be sure to check out the official Laravel documentation. It's a treasure trove of knowledge! 📚
That's a wrap! 🎉
By utilizing either the pluck()
or map()
method, you can easily retrieve specific attributes from a Laravel Collection. 🌟 You no longer have to worry about extracting unnecessary data from your collection. 🙌
Try out these solutions and let me know how they work for you! If you have any questions or suggestions, feel free to drop a comment below. Let's create some sleek arrays together! 😍🚀
Happy Laravel coding, amigos! 💻✨