Get Object From Collection By Attribute
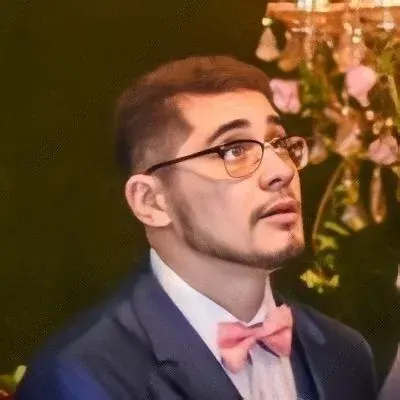
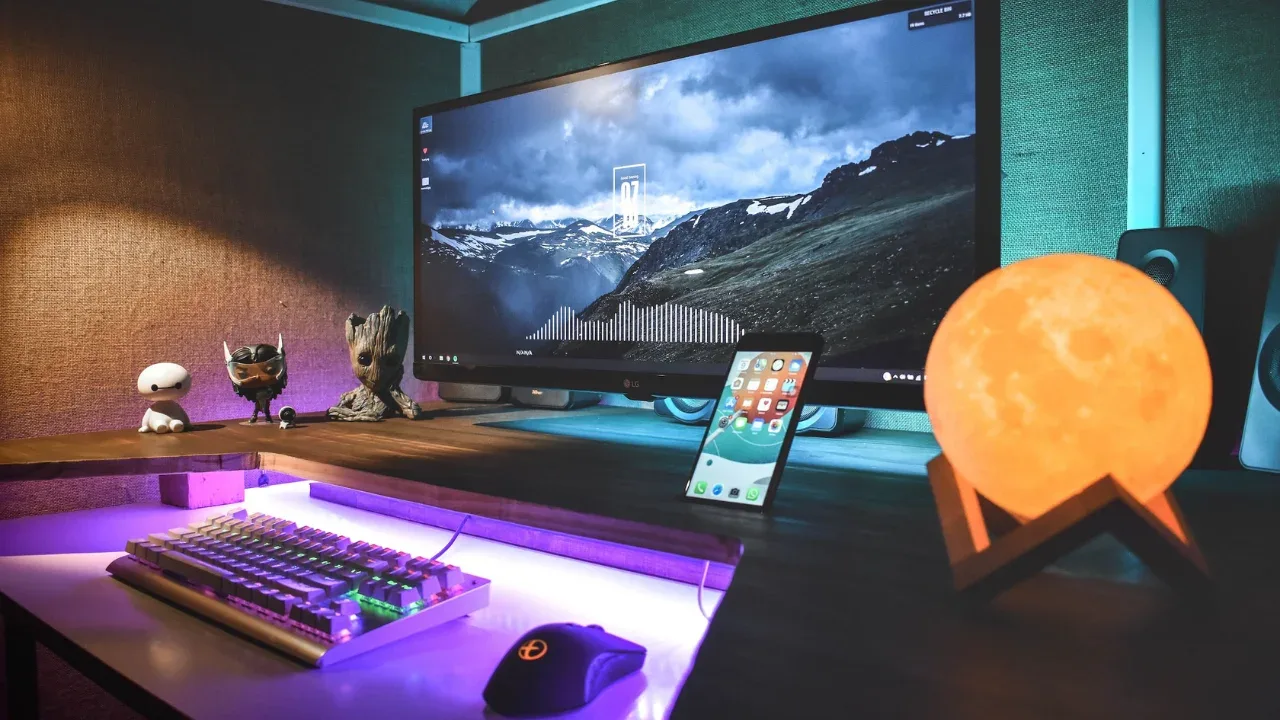
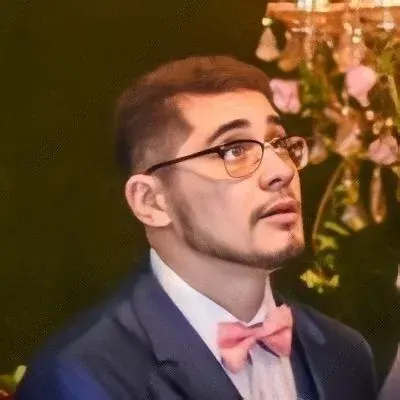
How to Get Object from Collection by Attribute in Laravel?
Are you tired of dealing with arrays of models in Laravel, where you can't directly access the object you want to modify? 🙄 Don't worry, we've got you covered! In this post, we'll discuss a common issue faced when working with collections in Laravel and provide easy solutions to get objects by attribute. Let's dive in! 💪
The Problem 😫
When you perform a query in Laravel and retrieve a collection of objects, the keys of the array are just numeric indices. So, if you want to modify a specific object by its attribute (e.g., id, color, age, etc.), you can't do it directly. Instead, you have to loop through the collection and check each object's attribute. 😓
However, there are cleaner and more efficient ways to tackle this issue. Let's explore some solutions! 🚀
Solution 1: Using the firstWhere
Method 👍
The firstWhere
method provided by the Illuminate Collection allows you to retrieve the first object that matches a given attribute and value. Here's an example:
$desired_object = $foods->firstWhere('id', 24);
$desired_object->color = 'Green';
$desired_object->save();
By using the firstWhere
method, you can directly access the object you want to modify without the need for looping. 🎉
Solution 2: Using the filter
Method 🙌
Another handy method provided by the Illuminate Collection is filter
. It allows you to filter the collection based on a condition and return a new collection with the matching objects. Here's an example:
$desired_objects = $foods->filter(function ($food) {
return $food->color == 'Green';
});
// Modifying the filtered objects
foreach ($desired_objects as $desired_object) {
$desired_object->color = 'Green';
$desired_object->save();
}
With the filter
method, you can retrieve all objects that match a specific attribute and condition, giving you more flexibility in modifying multiple objects. 😎
Solution 3: Using Eloquent Queries on Collection 🤩
If you have already performed the query and retrieved the collection, you can still use Eloquent queries on the collection itself. For example:
$green_foods = $foods->where('color', 'green');
// Modifying the green foods
foreach ($green_foods as $green_food) {
$green_food->color = 'Green';
$green_food->save();
}
This way, you can directly filter the collection without the need for an additional database query. 🎊
Conclusion and Next Steps 🏁
Now you know how to get objects from a collection by attribute in Laravel without the need for messy loops or unnecessary queries. 😄
Remember to choose the solution that best fits your needs: firstWhere
for retrieving a single object, filter
for retrieving multiple objects, and Eloquent queries on the collection if you have already performed the query.
If you found this post helpful, don't forget to share it with your fellow Laravel developers! Also, feel free to leave your thoughts in the comments below or reach out to us on social media.
Happy coding! ✨👩💻👨💻✨