Formatting a number with leading zeros in PHP
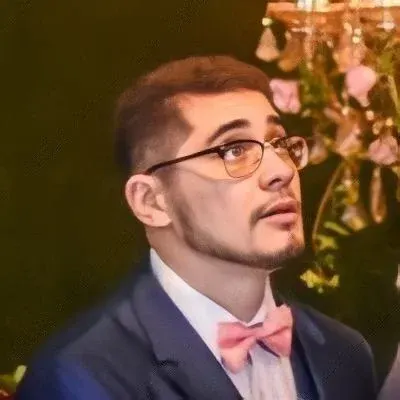

π°π» Give Your Numbers a Leading Zero in PHP π°π»
So, you've got a variable with a number that needs a leading zero? π No worries, my friend! PHP has got your back. You're just a few lines of code away from giving your numbers the desired formatting.
The situation is pretty straightforward: you have a variable containing the value 1234567
, and you want to pad it with a leading zero to make it contain exactly 8 digits, i.e., 01234567
. Sounds easy, right? It absolutely is! π
The Str_pad Function is Your Hero! π¦ΈββοΈ
To format your number with a leading zero, you can make use of the str_pad()
function, which is perfect for adding padding to strings.
Here's the basic syntax of str_pad()
:
str_pad($input_string, $desired_length, $padding_string, $padding_type);
$input_string
: The original string (or, in this case, your number).$desired_length
: The total length of the resulting string, including the padding. In our case, it's 8.$padding_string
: The string used for padding, which is a zero in this scenario.$padding_type
(optional): This specifies where the padding should be added. By default, it's added to the right. For left padding, useSTR_PAD_LEFT
.
Applying the Magic! π§ββοΈ
Now that we know how to use str_pad()
, let's apply this knowledge to our specific situation:
$original_number = "1234567";
$desired_length = 8;
$padding_string = "0";
$formatted_number = str_pad($original_number, $desired_length, $padding_string, STR_PAD_LEFT);
echo $formatted_number; // Output: 01234567
And just like that, your number has been successfully formatted! π
A Pro Tip for the π« Error-Prone π«
It's always a good idea to validate the inputs before using str_pad()
. You wouldn't want to waste precious milliseconds executing unnecessary code, would you? π
You can use a simple if
statement to check if the length of your number is less than the desired length. If it is, go ahead and apply the padding. Otherwise, leave it as is.
$original_number = "1234567";
$desired_length = 8;
$padding_string = "0";
if (strlen($original_number) < $desired_length) {
$formatted_number = str_pad($original_number, $desired_length, $padding_string, STR_PAD_LEFT);
} else {
$formatted_number = $original_number;
}
echo $formatted_number; // Output: 01234567
π¬ Let's Chat! π¬
Now that you've learned how to format a number with a leading zero in PHP, give it a try in your code and see the magic happen! If you encounter any issues or have any questions, feel free to drop a comment below. Let's geek out together! π€
π£ Stay Excited! π£
If you enjoyed this guide and found it helpful, consider sharing it with your fellow developers and tech enthusiasts. Together, we can make coding a little bit more awesome! π
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
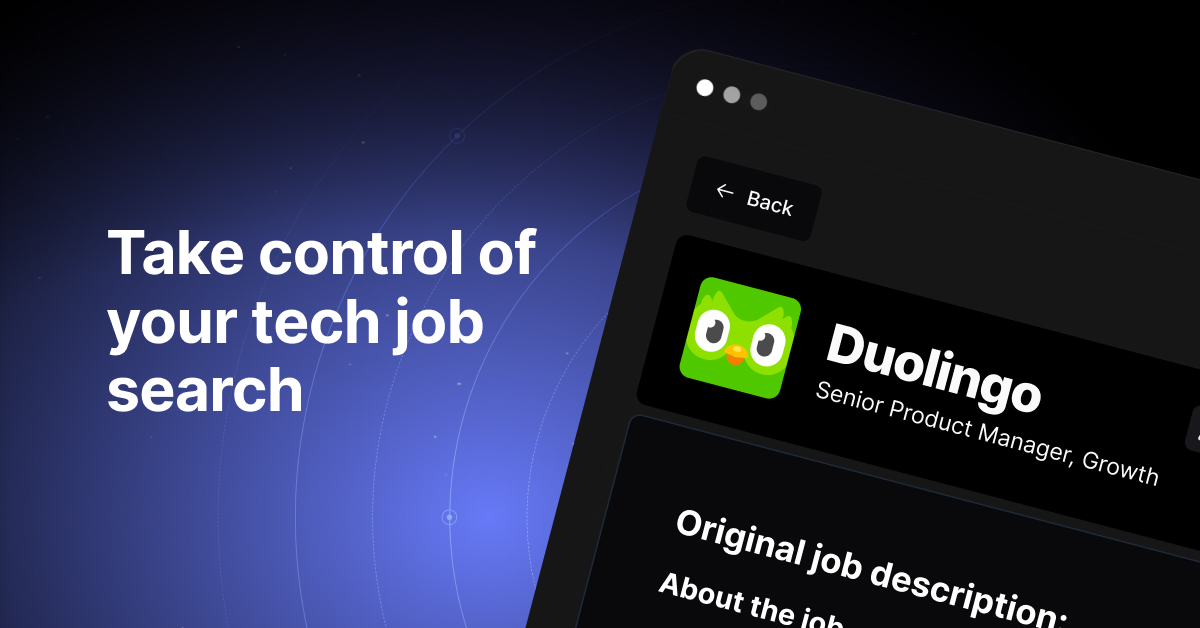