Facebook SDK returned an error: Cross-site request forgery validation failed. The "state" param from the URL and session do not match
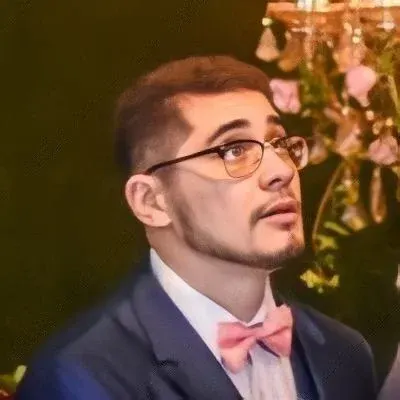
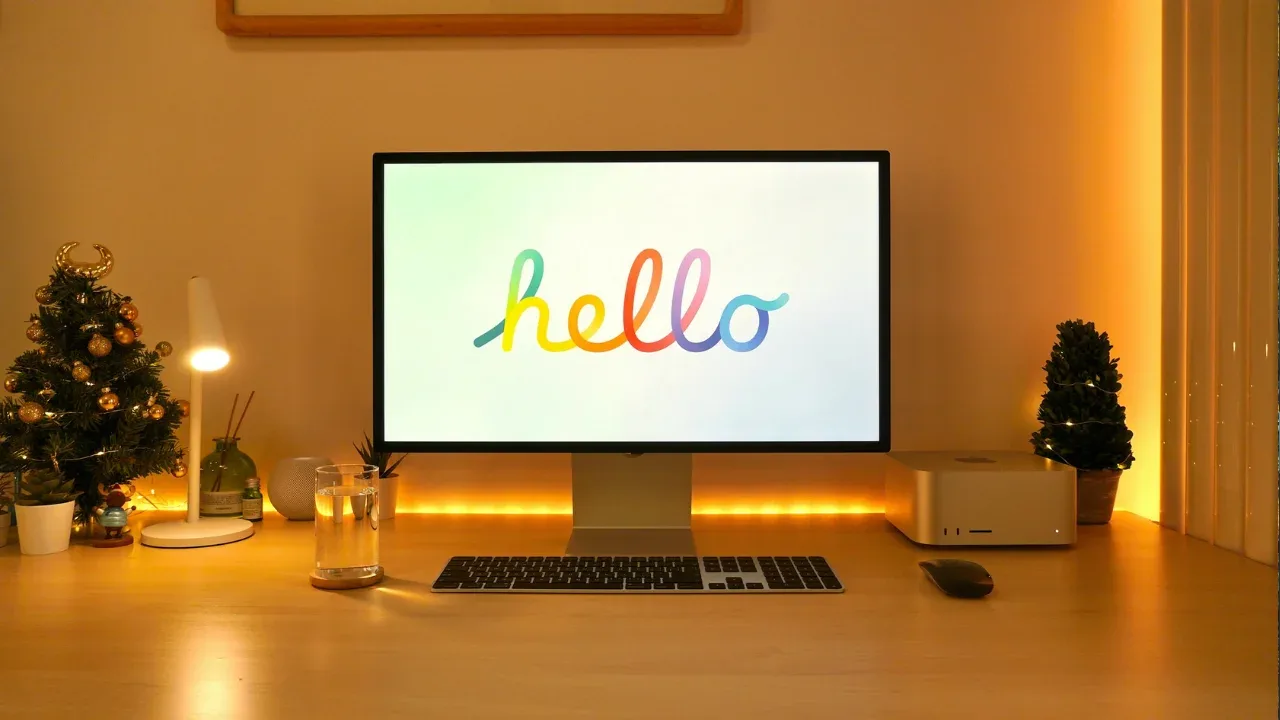
Facebook SDK: Cross-site Request Forgery Validation Failed
š Are you encountering the error message "Facebook SDK returned an error: Cross-site request forgery validation failed. The 'state' param from the URL and session do not match." when trying to get the Facebook user ID using the PHP SDK? Don't worry, you're not alone! This common issue can be easily resolved.
š Let's dive into the problem and find an easy solution.
Understanding the Error
The error message you're facing usually occurs due to a mismatch between the 'state' parameter residing in the URL and the session. This 'state' parameter is a security feature implemented by Facebook to prevent Cross-Site Request Forgery attacks.
The Solution
To resolve this issue, you need to make sure the 'state' parameter in the URL and your session match correctly. Fortunately, the solution is relatively simple.
First, ensure that your PHP script uses sessions correctly. Add the following line of code at the very beginning of your script, before any other code:
session_start();
This will initialize a session and enable you to store data between requests.
Next, make sure to pass the session variable as a second parameter to the getRedirectLoginHelper()
method, like this:
$helper = $fb->getRedirectLoginHelper($_SESSION);
This will allow the Facebook SDK to use the session data to validate the 'state' parameter properly.
Putting It All Together
Your updated code should now look like this:
$fb = new Facebook\Facebook([
'app_id' => '11111111111',
'app_secret' => '1111222211111112222',
'default_graph_version' => 'v2.4',
]);
session_start();
$helper = $fb->getRedirectLoginHelper($_SESSION);
$permissions = ['public_profile','email']; // Optional permissions
$loginUrl = $helper->getLoginUrl('http://MyWebSite', $permissions);
echo '<a href="' . $loginUrl . '">Log in with Facebook!</a>';
// The rest of your code...
š” Remember to replace 'http://MyWebSite'
with your actual website URL.
Still Having Trouble?
If you're still facing issues after implementing the above solution, try the following troubleshooting steps:
Ensure that the session is enabled in your PHP configuration.
Make sure the
session.save_path
is properly set in your php.ini file.Double-check that you're using the correct Facebook app ID and app secret.
Verify that the 'state' parameter in the URL is not being modified or tampered with.
Clear your browser cookies and try again.
If the problem persists, consider checking the Facebook SDK documentation or reaching out to the Facebook developer community for assistance.
Conclusion
š Congratulations on resolving the "Cross-site request forgery validation failed" error when using the Facebook PHP SDK! With the updated code and a correct session setup, you should now be able to obtain the Facebook user ID without any issues.
š If you found this guide helpful or have any further questions, let us know in the comments below! Happy coding! š»
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
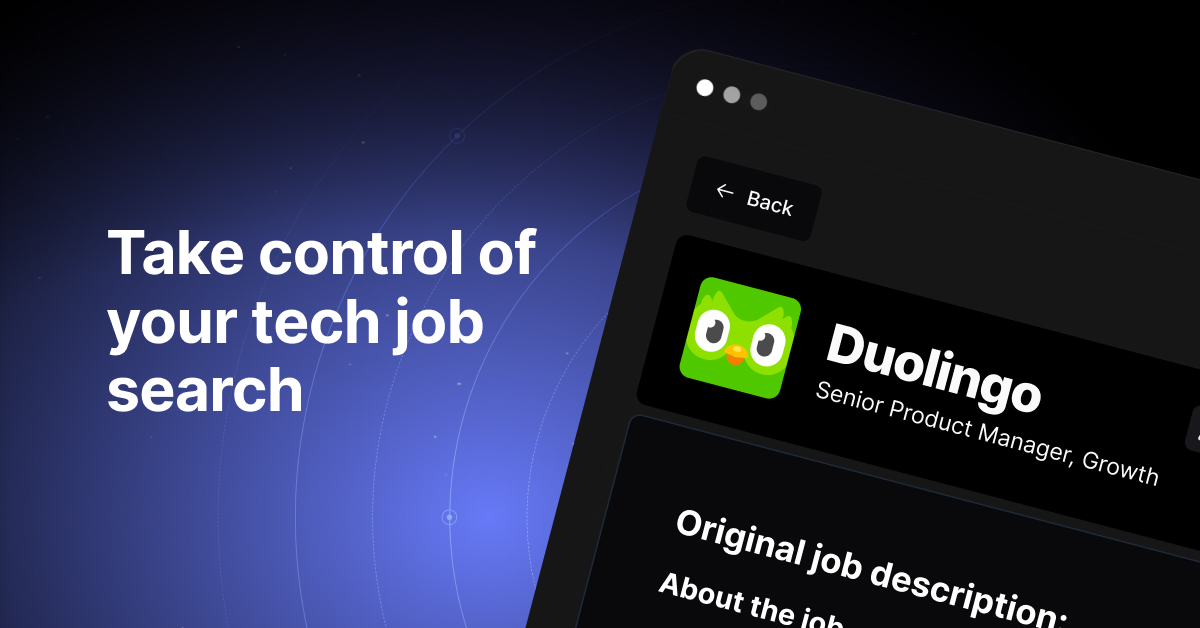