Error “Target class controller does not exist” when using Laravel 8
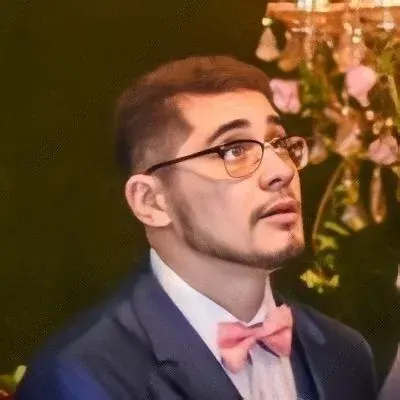
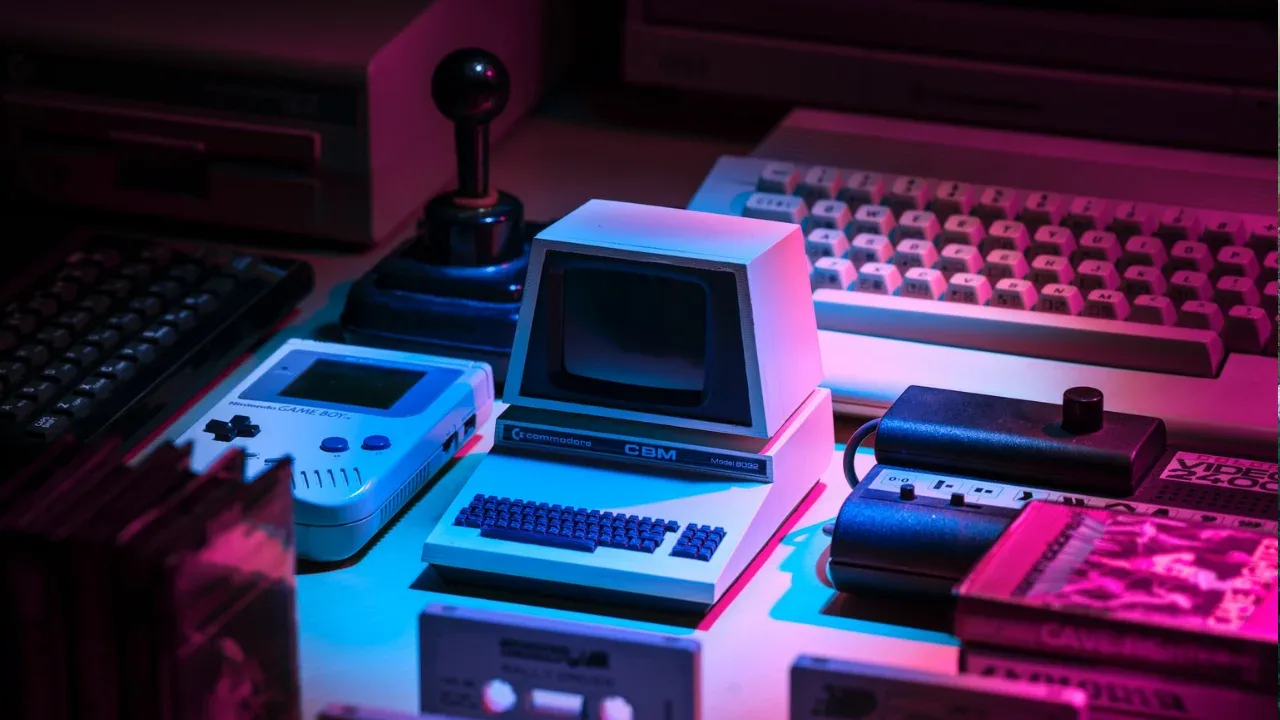
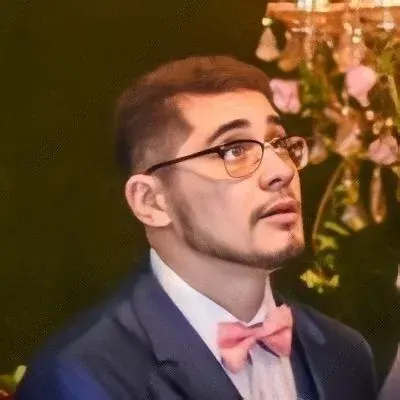
Solution to the Error "Target class controller does not exist" in Laravel 8 🚀
So, you're facing an error that says "Target class [Api\RegisterController] does not exist" when trying to access your register
route in Laravel 8. Don't worry, we've got you covered! Let's dive into the issue and find the solution. 🤔
Understanding the Problem 📚
The error is thrown because Laravel cannot find the specified class Api\RegisterController
when attempting to execute the corresponding route. This can happen due to various reasons, such as incorrect namespace, improper file placement, or incorrect routing syntax.
Identifying the Cause 🕵️♀️
Let's break down the provided code snippet and identify potential problems:
<?php
namespace App\Http\Controllers\Api;
use App\Http\Controllers\Controller;
use Illuminate\Http\Request;
class RegisterController extends Controller
{
public function register(Request $request)
{
dd('aa');
}
}
The
RegisterController
class is correctly placed inside theApp\Http\Controllers\Api
namespace.The file placement seems fine based on the provided screenshot.
The
register
method exists within theRegisterController
class.
Route::get('register', 'Api\RegisterController@register');
The route is defined correctly and tries to access the
register
method in theApi\RegisterController
.
Proposed Solutions 💡
1. Use the Fully Qualified Class Name
As mentioned in the comment from the OP in the provided context, using the fully qualified class name might resolve the issue. Update your route definition to the following:
Route::get('register', 'App\Http\Controllers\Api\RegisterController@register');
By specifying the complete namespace, Laravel will be able to locate the RegisterController
correctly.
2. Check Namespace and Filename Consistency
Ensure that the namespace declared in the RegisterController
matches the actual file path and class name. In this case, the file should be placed at app/Http/Controllers/Api/RegisterController.php
, with a class name of RegisterController
.
3. Clear Cache and Auto-load Classes
Sometimes the issue can be caused by the Laravel's cache not being refreshed or the autoloader not being up-to-date. You can try running the following artisan commands to remove cached files and reload the classes:
php artisan cache:clear
composer dump-autoload
4. Confirm Composer Autoload Configuration
Make sure the composer.json
file in your Laravel project includes the correct autoload configuration. It should look something like this:
"autoload": {
"psr-4": {
"App\\": "app/"
}
},
5. Verify Controller Name Capitalization
Ensure that the controller class name "RegisterController" is spelled correctly with the correct capitalization when used in your route definition.
Conclusion 🎉
By following one or a combination of the above solutions, you should be able to fix the "Target class [Api\RegisterController] does not exist" error in Laravel 8.
If you found this guide helpful or have any further questions, let us know in the comments section below. Happy coding! 😄✨