eloquent laravel: How to get a row count from a ->get()
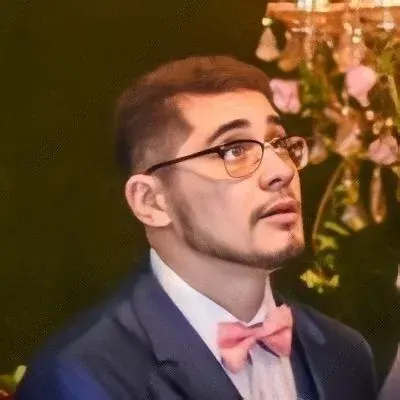
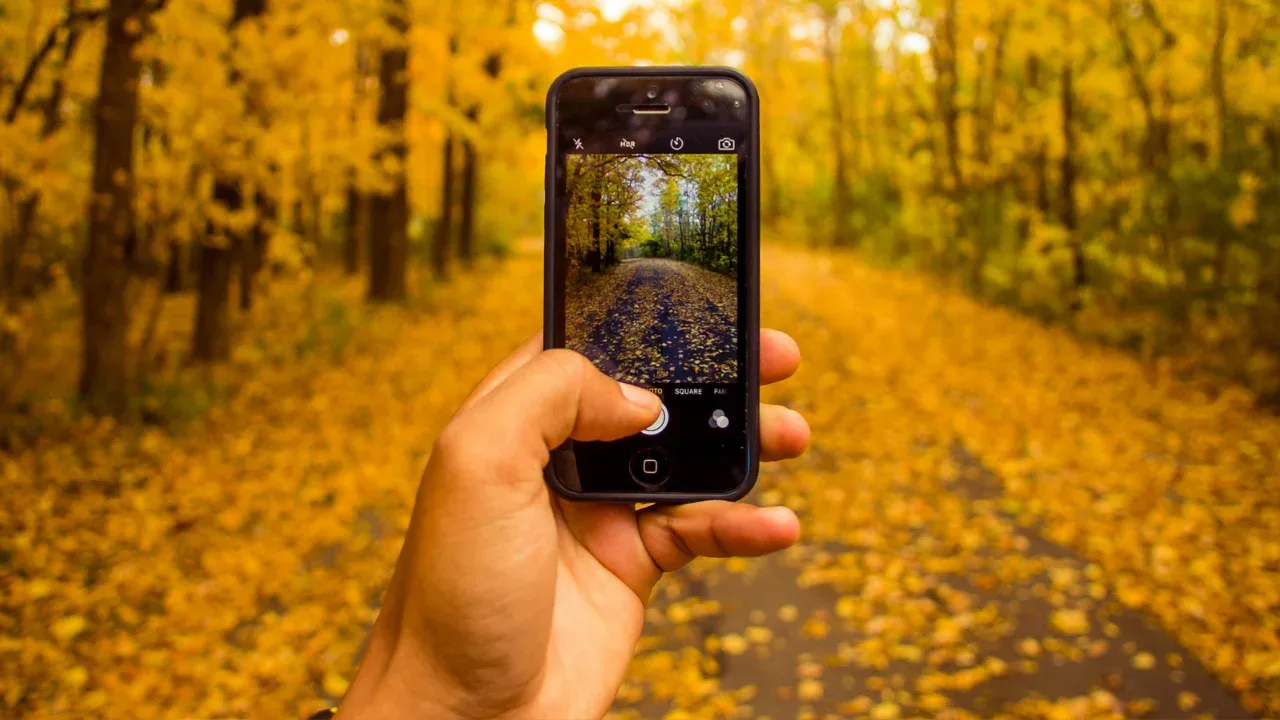
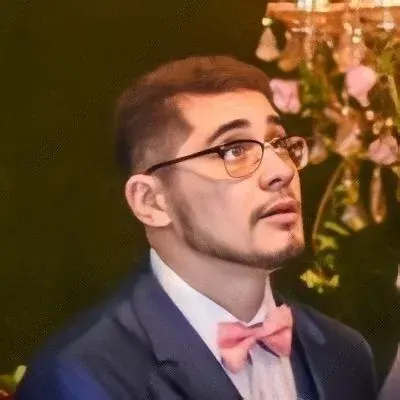
How to Get a Row Count from a ->get()
in Eloquent Laravel
So, you're using Laravel's Eloquent ORM and you're facing a problem when trying to get a row count from a ->get()
query result. Don't worry, you're not alone! Many developers struggle with this as well. But fear not, because I'm here to help you out! 😄
The Problem
Let's take a look at the code snippet that you provided:
$wordlist = \DB::table('wordlist')->where('id', '<=', $correctedComparisons)->get();
In this case, $wordlist
is a collection of results returned by the ->get()
method. Collections are awesome, but they don't come with a ->count()
method out of the box like query builders do. So, how can we get the row count from this collection?
The Solution
Luckily, Laravel provides a simple solution for this problem. You can use the ->count()
method on the collection itself. Here's how you can do it:
$count = $wordlist->count();
By calling the ->count()
method on the collection, you can obtain the row count without the need for an additional database query. 🎉
How It Works
When you use the ->get()
method, it returns a collection of results. This collection is an instance of the Illuminate\Support\Collection
class, which provides various helpful methods, including ->count()
. This method calculates and returns the number of items in the collection.
Additional Tips
If you prefer a more concise approach, you can also use the count()
function as follows:
$count = count($wordlist);
Both $wordlist->count()
and count($wordlist)
will return the same result - the row count of the collection.
Conclusion
Now that you know how to get a row count from a ->get()
query in Eloquent Laravel, you can confidently tackle this problem in your projects. 🙌
Remember, collections in Laravel are powerful and offer a wide range of methods to make your life as a developer easier. Don't hesitate to explore the Laravel documentation to learn more about what collections can do!
If you have any other questions or need further assistance, feel free to leave a comment below. Happy coding! 💻
Have you ever been stuck trying to get a row count from a ->get()
in Eloquent Laravel? Share your experience with us in the comments section below!