Eloquent get only one column as an array
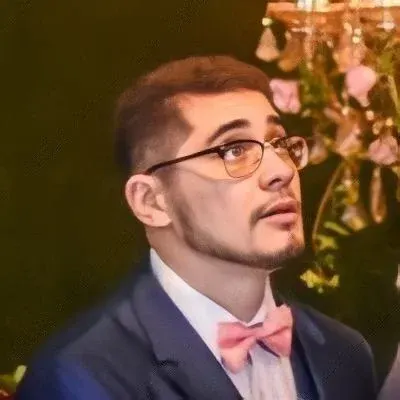

Getting Only One Column as a One-Dimensional Array in Laravel 5.2 Using Eloquent
Are you struggling to get only one column as a one-dimensional array in Laravel 5.2 using Eloquent? Don't worry, you're not alone! Many developers face this issue when working with Eloquent.
The Problem
Let's take a look at the code snippet you provided:
$array = Word_relation::select('word_two')->where('word_one', $word_id)->get()->toArray();
This code seems logical at first, as it selects only the "word_two" column from the "Word_relation" table where "word_one" matches a given $word_id
. However, the result is not what we expect. Instead of a one-dimensional array, we end up with a two-dimensional array like this:
array(2) {
[0]=>
array(1) {
["word_one"]=>
int(2)
}
[1]=>
array(1) {
["word_one"]=>
int(3)
}
}
The Solution
To get only one column as a one-dimensional array, you can make use of the pluck()
method provided by Laravel's Eloquent. Here's how you can modify your code:
$array = Word_relation::where('word_one', $word_id)->pluck('word_two')->toArray();
Using the pluck()
method, we first filter the records where "word_one" matches the given $word_id
. Then, we specify the specific column we want to retrieve, which is "word_two" in this case.
Now, let's see the result you're looking for:
array(2) {
[0]=> 2
[1]=> 3
}
Voila! You successfully obtained a one-dimensional array with only the "word_two" column values.
Wrapping Up
Getting only one column as a one-dimensional array in Laravel 5.2 is a common issue, but thankfully there's a simple solution. By using the pluck()
method, you can easily retrieve the desired column values.
Next time you find yourself facing this problem, remember to modify your Eloquent query using the pluck()
method. It will save you time and headaches!
Do you have any other Laravel-related questions or issues? Let us know in the comments below and we'll be happy to help!
🙌 Let's Connect!
We love engaging with our readers and community. Feel free to follow us on social media and subscribe to our newsletter for more Laravel tips, tricks, and tutorials.
Facebook: [link]
Twitter: [link]
Instagram: [link]
Newsletter: [link]
📚 Further Reading
If you enjoyed this article and want to dive deeper into Laravel, check out these related articles:
"Mastering Eloquent Relationships in Laravel" [link]
"Getting Started with Laravel Query Builder" [link]
"10 Tips and Tricks for Laravel Developers" [link]
Thanks for reading! Stay tuned for more exciting Laravel content. Let's keep coding! 💻💪
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
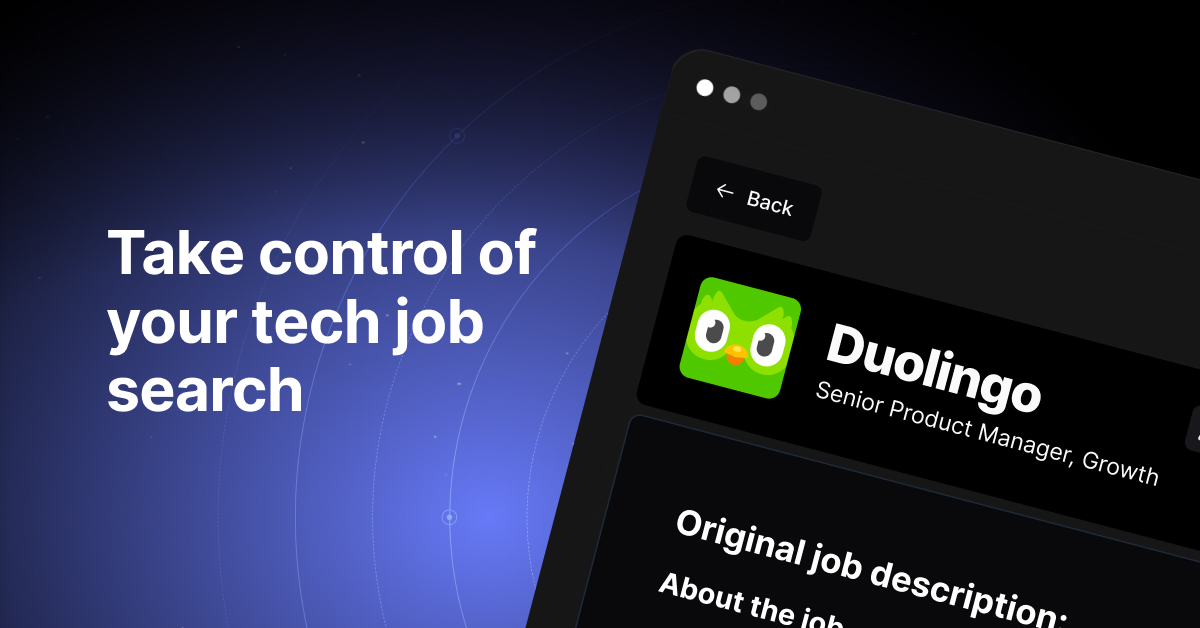