Dropping column with foreign key Laravel error: General error: 1025 Error on rename
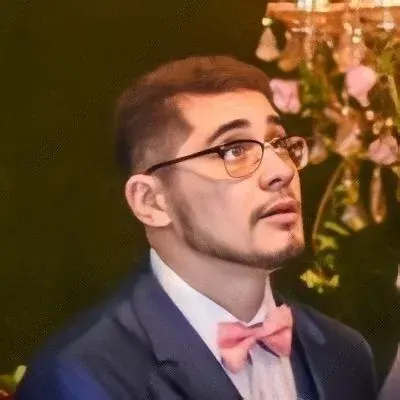
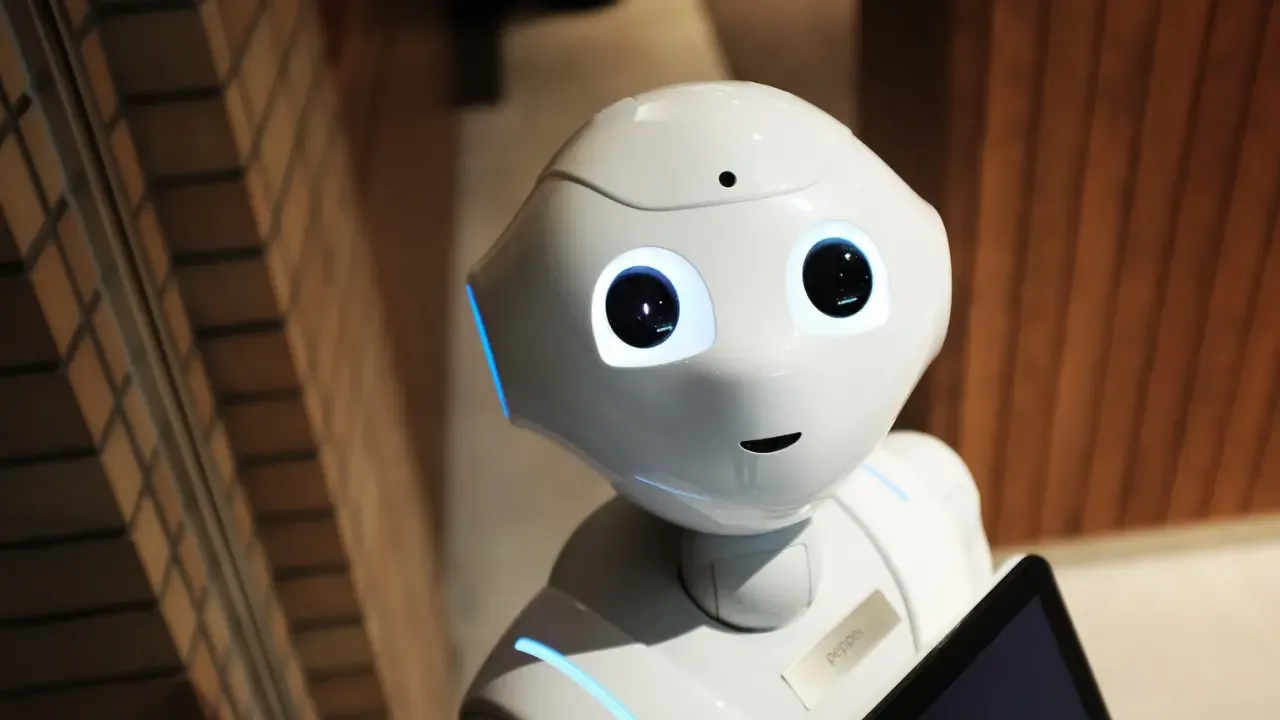
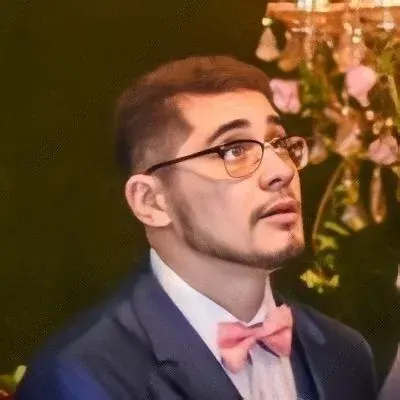
📝 Blog Post: Dropping Column with Foreign Key in Laravel: Troubleshooting General Error 1025
Hey there, Laravel developers! 👋 Have you ever encountered the dreaded "General error: 1025 Error on rename" when trying to drop a column with a foreign key reference in your migration? 😫 Don't worry, you're not alone! In this blog post, we'll explore the common issues that cause this error and provide you with easy solutions to resolve it. So, grab your ☕️ and let's dive in!
Understanding the Problem
The "General error: 1025 Error on rename" occurs when attempting to drop a column that has a foreign key constraint in Laravel. In the provided context, our goal is to drop the pick_detail_id
column and add a new sku
column in the despatch_discrepancies
table.
Solution 1: Correct Migration Order
One possible cause of this error is the migration order. Since the foreign key constraint depends on the pick_detail_id
column, we need to drop the constraint first before removing the column itself. Let's update our migration:
public function up()
{
Schema::table('despatch_discrepancies', function ($table) {
$table->dropForeign('despatch_discrepancies_pick_detail_id_foreign');
$table->dropColumn('pick_detail_id');
});
Schema::table('despatch_discrepancies', function ($table) {
$table->string('sku', 20)->after('pick_id');
});
}
public function down()
{
Schema::table('despatch_discrepancies', function ($table) {
$table->integer('pick_detail_id')->unsigned();
$table->foreign('pick_detail_id')->references('id')->on('pick_details');
});
Schema::table('despatch_discrepancies', function ($table) {
$table->dropColumn('sku');
});
}
By dropping the foreign key constraint before the column, we ensure a smooth alteration. 🚀
Solution 2: Proper Foreign Key Naming
Another possible cause is a mismatch between the foreign key name used for dropping the constraint and the actual foreign key name in the database. To avoid this, it's best to explicitly name your foreign keys during creation. Let's update our initial migration:
public function up()
{
Schema::create('despatch_discrepancies', function($table) {
$table->increments('id')->unsigned();
$table->integer('pick_id')->unsigned();
$table->foreign('pick_id')->references('id')->on('picks');
$table->integer('pick_detail_id')->unsigned()->index('fk_despatch_discrepancies_pick_detail_id');
$table->foreign('pick_detail_id', 'fk_despatch_discrepancies_pick_detail_id')
->references('id')->on('pick_details');
$table->integer('original_qty')->unsigned();
$table->integer('shipped_qty')->unsigned();
});
}
// Rest of the migration remains the same...
By explicitly naming the foreign key constraint, Laravel can easily drop the correct constraint without any conflicts. 💪
Solution 3: Manual Dropping of Constraint
If the provided solutions didn't work for you, we can try manually dropping the constraint using raw SQL statements. Here's an alternative approach:
public function up()
{
DB::statement('ALTER TABLE despatch_discrepancies DROP FOREIGN KEY despatch_discrepancies_pick_detail_id_foreign');
Schema::table('despatch_discrepancies', function ($table) {
$table->dropColumn('pick_detail_id');
$table->string('sku', 20)->after('pick_id');
});
}
public function down()
{
Schema::table('despatch_discrepancies', function ($table) {
$table->integer('pick_detail_id')->unsigned();
$table->foreign('pick_detail_id')->references('id')->on('pick_details');
$table->dropColumn('sku');
});
}
Here, we are using the DB::statement
method to execute a raw SQL statement and drop the foreign key constraint explicitly.
Still Stuck? Seek Community Help!
If none of the above solutions worked for you, fear not! Laravel has a vibrant and supportive community. Share your problem on forums, reach out on social media, or ask for help on platforms like Stack Overflow. 🙌
That's it, folks! We hope this guide helped you understand and resolve the "General error: 1025 Error on rename" issue when dropping a column with a foreign key constraint in Laravel. Remember to backup your database before performing migrations, just in case! 😉
If you found this blog post helpful, don't forget to share it with your fellow Laravel enthusiasts. Plus, we'd love to hear your thoughts and experiences in the comments below. Let's build amazing Laravel applications together! 👨💻🚀