Disable Laravel"s Eloquent timestamps
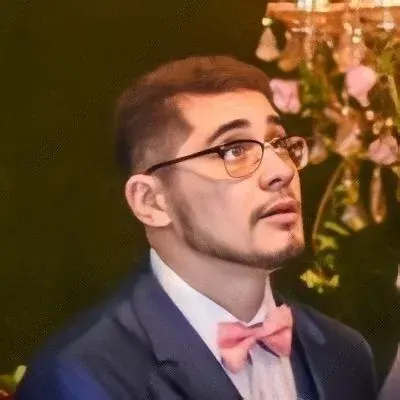
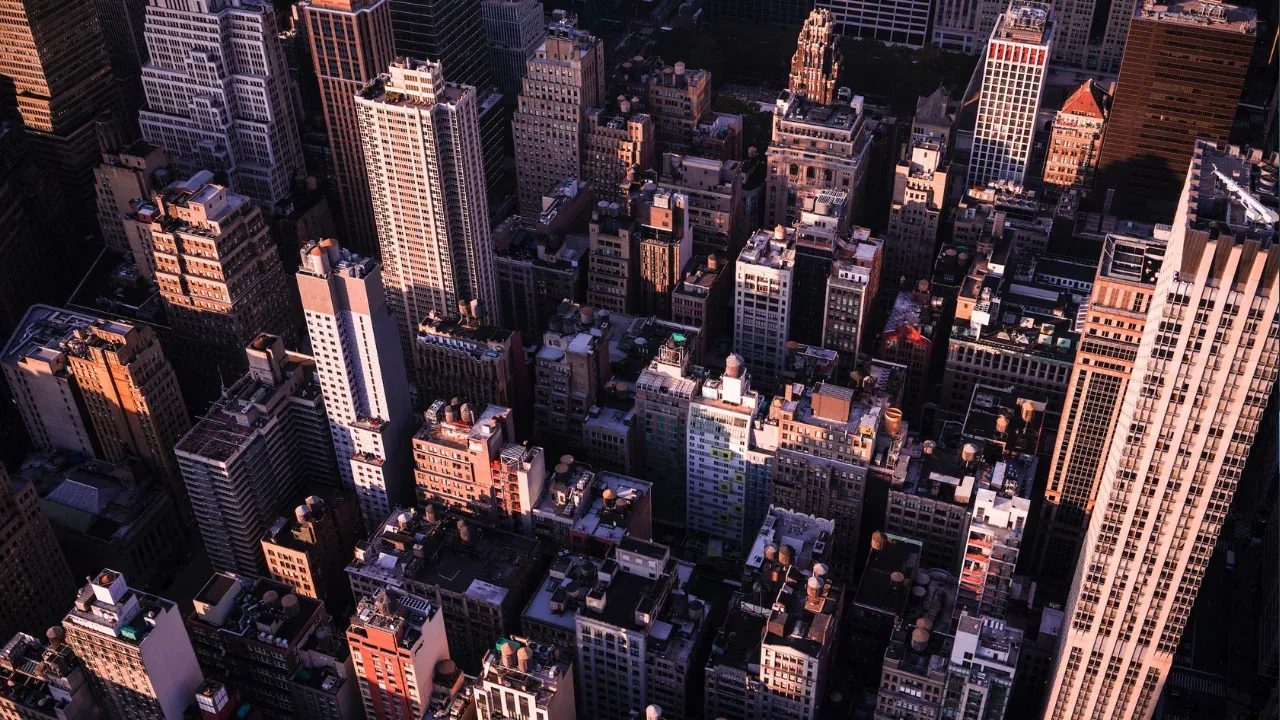
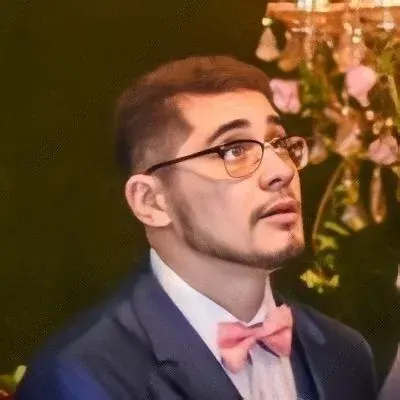
How to Disable Laravel's Eloquent Timestamps
So, you're converting your web application from CodeIgniter to Laravel, but you're facing an issue with Laravel's Eloquent timestamps. You don't want to add the updated_at
and created_at
fields to all of your tables because you already have a logging class that handles this for you. Don't worry, there's a solution!
The Problem: Unwanted Eloquent Timestamps
Laravel's Eloquent ORM automatically manages timestamps for your database records. By default, every Eloquent model has created_at
and updated_at
fields, which get automatically updated whenever records are created or updated.
But in your case, you have a dedicated logging class that handles timestamps and you don't want to clutter your tables with additional timestamp fields.
The Solution: Disabling Timestamps
Thankfully, Laravel provides an easy way to disable Eloquent timestamps without the need to modify core files or add code to every model class. Here's how you can do it:
Step 1: Create a Base Model Class
Create a base model class that extends Laravel's Eloquent Model
class. This base model will serve as a parent class for all your models, allowing you to customize Eloquent's behavior.
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class BaseModel extends Model
{
public $timestamps = false;
}
Step 2: Extend the Base Model in Your Models
Now, instead of extending Laravel's Model
class directly, extend your newly created BaseModel
class in all your models. This way, the public $timestamps = false;
property will be inherited, disabling timestamps for all your models.
<?php
namespace App\Models;
class User extends BaseModel
{
// Your model code here
}
Step 3: Update the Namespace
Make sure to update the namespace of your models to reflect the new location of your BaseModel
. For example, if you placed your BaseModel
class in the App\Models
namespace, update your models like this:
<?php
namespace App\Models;
class User extends BaseModel
{
// Your model code here
}
That's it! By extending the BaseModel
class in your models, all of them will now have timestamps disabled. You won't need to modify the core files or add code to every model individually.
Call-to-Action: Share Your Experience and Insights
Have you faced any issues with Laravel's Eloquent timestamps? How did you tackle them? Share your experience and insights with the community in the comments below. Let's help each other optimize our Laravel development workflows! 💪💻
Remember, if you found this guide helpful, don't forget to share it with your developer friends who might be struggling with Eloquent timestamps. Together, we can make Laravel development even smoother! 🚀🌟