Difference between array_map, array_walk and array_filter
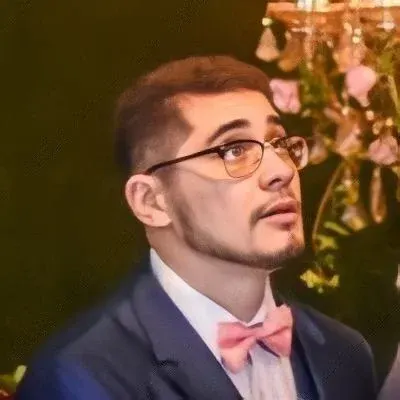
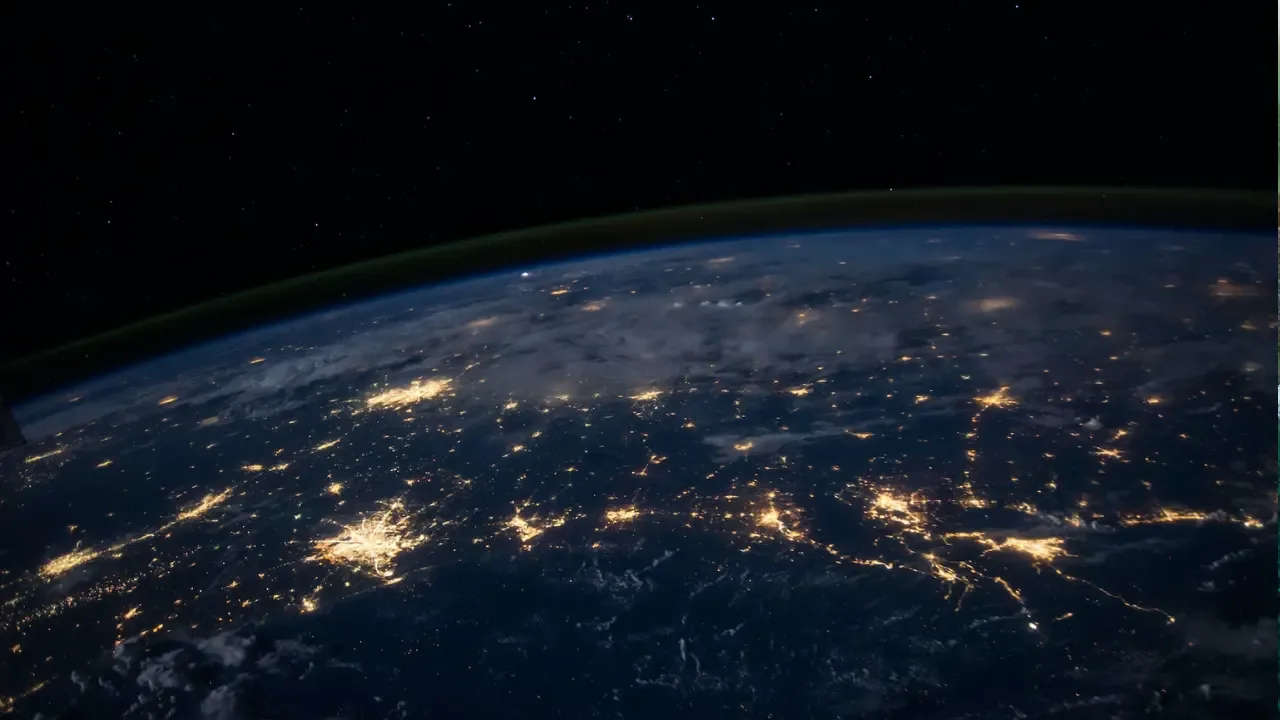
Demystifying array_map, array_walk, and array_filter in PHP
💡 Are you confused🤯 about the differences between array_map
, array_walk
, and array_filter
in PHP? 🤔 Don't worry, my friend, I've got you covered! Let's dive into the magical world of arrays and callbacks 🌟
Understanding the basics
⚡ First things first, let's understand what each function does:
1. array_map
:
array_map
is a function that applies a callback function to each element of an array and returns a new array containing the modified values⚡. It works by iterating over each element of the input array and passing the element as an argument to the callback function🔜. You can then perform any desired operation on each element and the modified value will be stored in the resulting array.
2. array_walk
:
On the other hand, array_walk
is a function that applies a callback function to each element of an array in place🔄. This means that it modifies the original array directly, without creating a separate copy. The callback function receives both the value and the key of the current array element as arguments and can modify the element or perform any desired operation on it💪.
3. array_filter
:
Meanwhile, array_filter
is a function that filters an array by applying a callback function to each element. It returns a new array containing only the elements for which the callback function returns true
🔍. The callback function should return either true
or false
based on a condition that you define. This is useful for selectively extracting specific elements from an array based on your criteria.
How are they different?
🔁 To put it simply:
array_map
modifies and returns a new array.array_walk
modifies the original array directly.array_filter
returns a new array containing only the filtered elements.
✨ Let's illustrate these differences with some examples:
$numbers = [1, 2, 3, 4, 5];
// Example 1: array_map
$squaredNumbers = array_map(function($num) {
return $num * $num;
}, $numbers);
print_r($squaredNumbers);
// Output: [1, 4, 9, 16, 25]
// Example 2: array_walk
$sum = 0;
array_walk($numbers, function($num) use (&$sum) {
$sum += $num;
});
echo $sum;
// Output: 15
// Example 3: array_filter
$evenNumbers = array_filter($numbers, function($num) {
return $num % 2 === 0;
});
print_r($evenNumbers);
// Output: [2, 4]
🎉 Cool, right? By understanding the differences between these functions, you can now use them effectively in your code and save yourself from potential confusion.
⚡ So, to answer your questions:
💡 No, array_map
, array_walk
, and array_filter
do not perform the same thing. Each function has its unique purpose, which we just explored.
💡 While there might be some scenarios where you could use them interchangeably, it's essential to understand the differences and choose the one that best fits your specific use case.
🚀 Now it's your turn! Go ahead and experiment with these functions and have fun unleashing their full potential in your PHP code!
🎁 If you found this guide helpful, please consider sharing it with your fellow coders and spread the knowledge! 💙
✉️ I would love to hear your thoughts and answer any further questions you might have. Drop a comment below or reach out to me on Twitter. Let's code together and conquer the PHP universe! 🌌
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
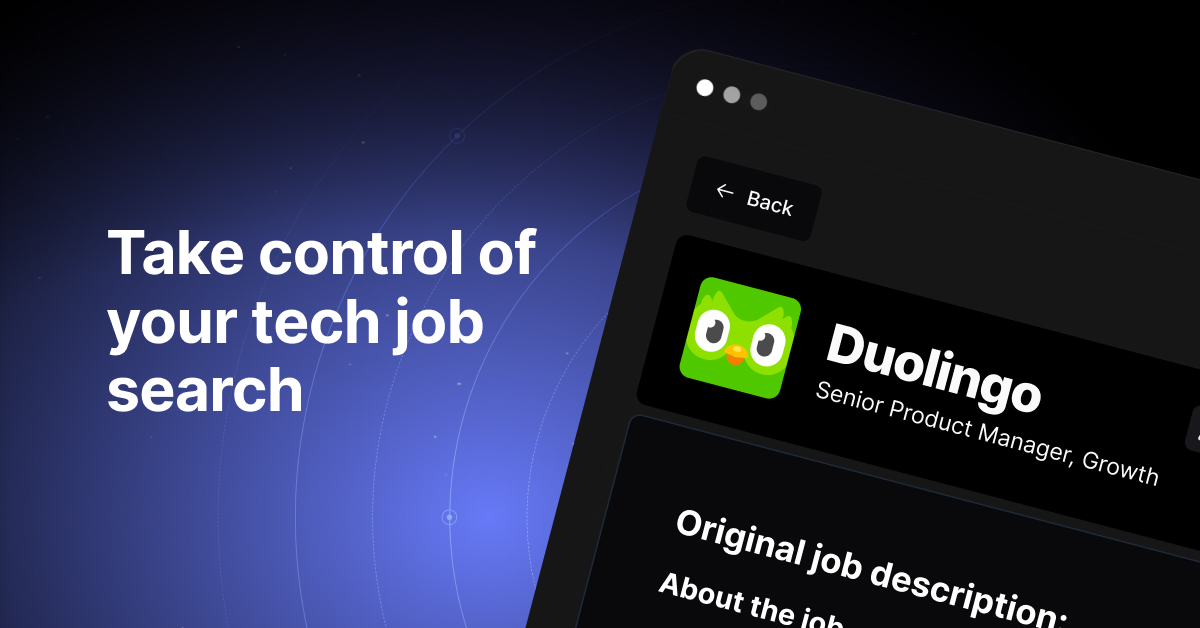