Creating and Update Laravel Eloquent
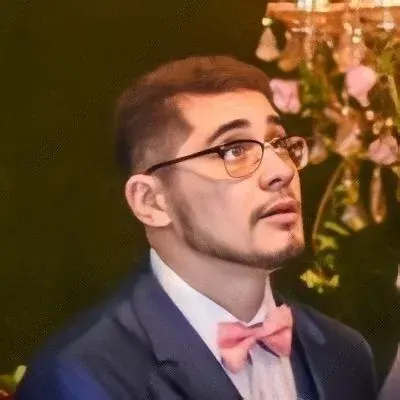
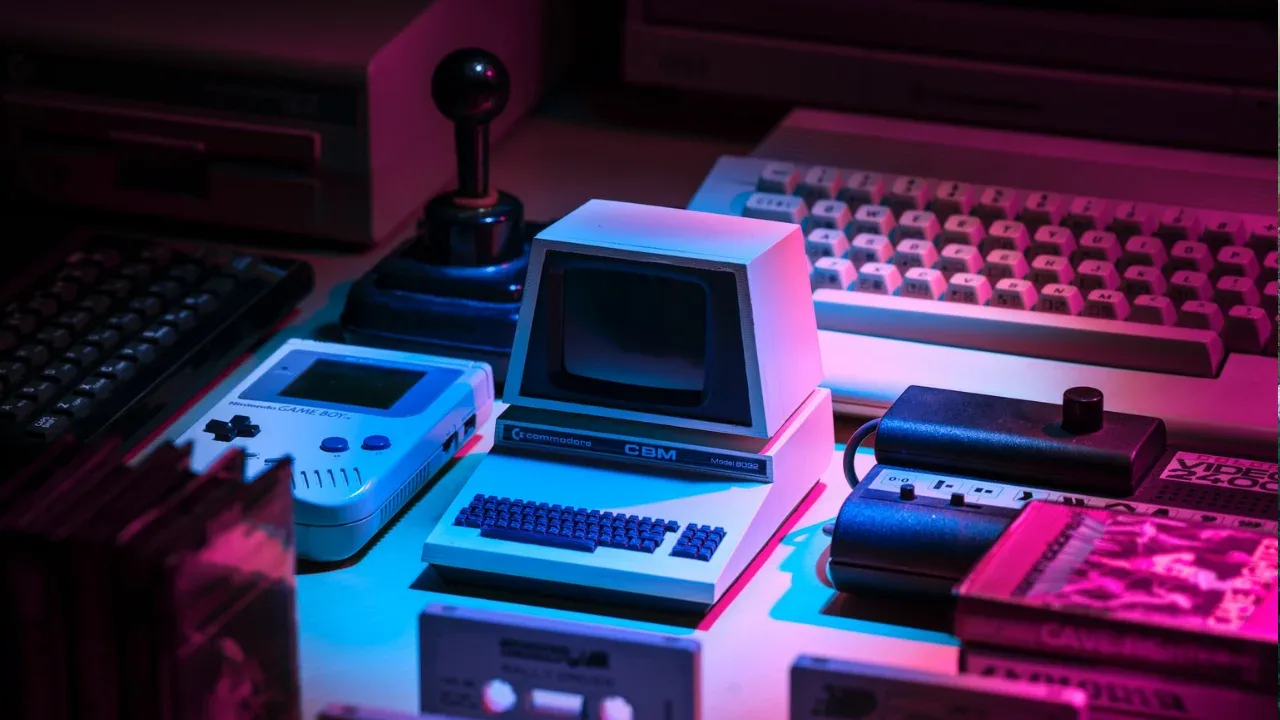
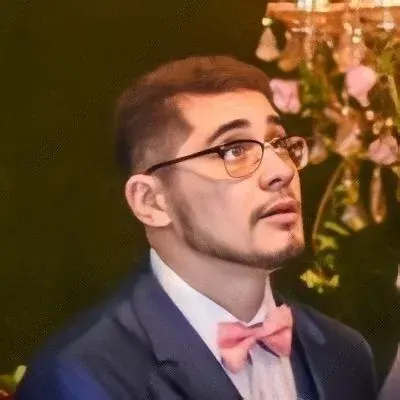
Creating and Updating Records in Laravel Eloquent: A Quick Guide 💻🔥
So, you want to know the shorthand for inserting a new record or updating if it already exists in Laravel Eloquent? You've come to the right place! In this blog post, we'll dive into this common issue and provide you with easy solutions that will save you time and headaches. Let's get started! 🚀
The Problem 😕
In the code snippet you provided, the goal is to check if a record exists in the database based on certain conditions. If it does, you want to update the existing record. Otherwise, you want to insert a new record. While the code works, it can be optimized and made more concise. We'll walk you through the solution step by step. 💪
The Solution 💡
Laravel Eloquent provides a powerful method called updateOrCreate
that simplifies the process of creating or updating records. Let's see how it looks in action:
$shopOwner = ShopMeta::updateOrCreate(
['shopId' => $theID, 'metadataKey' => 2001],
['additionalData' => 'example']
);
In this example, we use the updateOrCreate
method on the ShopMeta
model. The first argument is an array of conditions to locate the record. If a record matching these conditions is found, it will be updated with the new data provided in the second argument. If no matching record is found, a new record will be created with both the conditions and additional data.
Understanding the Code 🤓
Let's break down the code snippet to fully understand what's happening here.
We call the
ShopMeta
model and use theupdateOrCreate
method on it.The first argument is an array of conditions. In this case, we're looking for a record where the
shopId
column is equal to$theID
and themetadataKey
column is equal to2001
.The second argument is an array of data we want to update or insert. In this example, we're setting the
additionalData
column to'example'
.The
updateOrCreate
method returns the updated or newly created instance of theShopMeta
model, which we can assign to the$shopOwner
variable.
That's it! 😄
Why Should You Use updateOrCreate
? 🤔
Using the updateOrCreate
method has several advantages:
Simplicity: The method provides a cleaner and more concise syntax, eliminating the need for manual checks and unnecessary if-else statements.
Efficiency: By utilizing a single method, you reduce the number of database queries required, resulting in improved performance.
Clarity: The code becomes more readable and easier to understand for other developers working on the project.
Your Turn! ✍️
Now that you've learned the shorthand for creating and updating records in Laravel Eloquent using the updateOrCreate
method, it's time to put your knowledge into action! Try implementing this approach in your own project and see how it simplifies your code. Don't forget to share your experiences in the comments below. We'd love to hear from you! 💬
Happy coding! 💻😊