Convert a date format in PHP
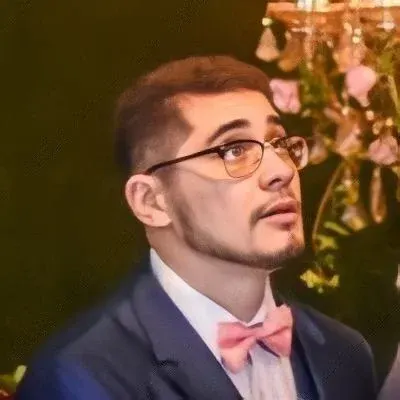
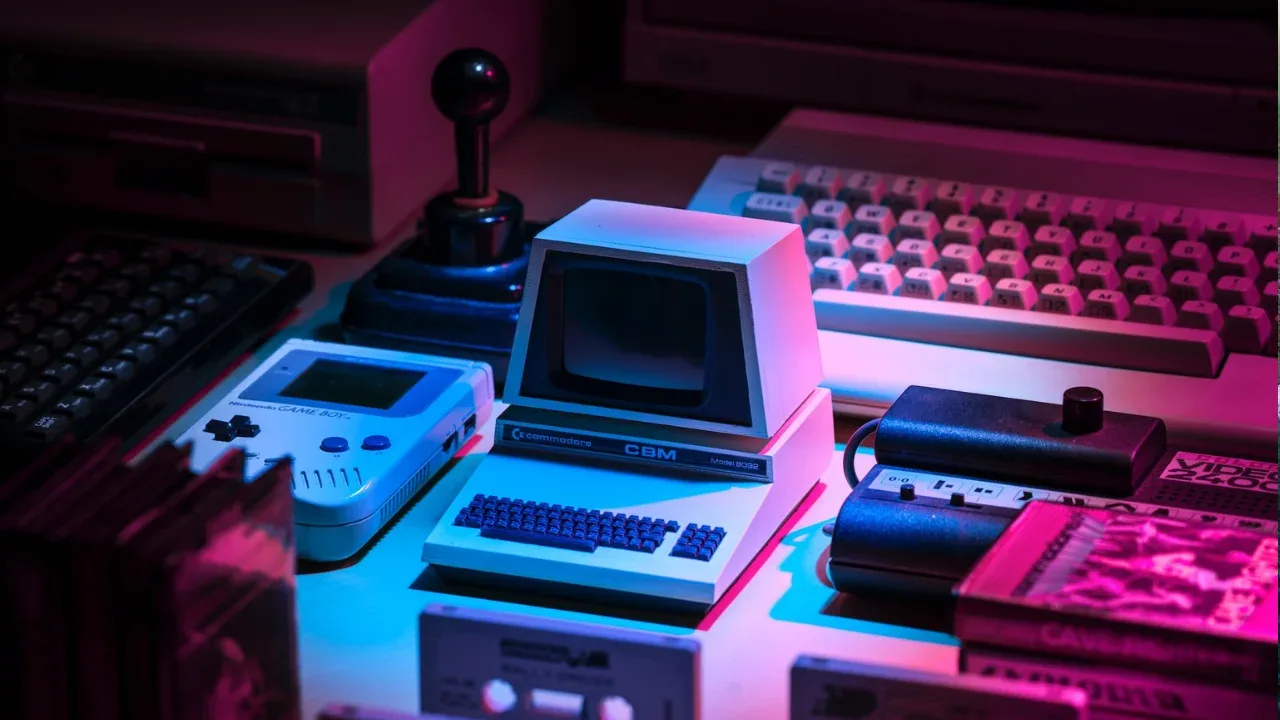
Converting a Date Format in PHP: Unlock the Code! 📅🔓
So you've stumbled upon the classic date format conversion dilemma in PHP. You need to convert a date from yyyy-mm-dd
format to dd-mm-yyyy
, but you're facing a roadblock. Fear not, intrepid coder! In this blog post, we'll walk you through the common issues, provide easy solutions, and empower you to conquer this date conversion quest. Let's dive in! 💻🚀
The Challenge 💥
The issue our fellow developer is encountering is that the PHP date function requires a timestamp, but they are unable to obtain a timestamp from the yyyy-mm-dd
date string. This is a common obstacle that often leaves developers scratching their heads. But worry not, we have some tricks up our sleeves to make this seemingly elusive conversion a piece of 🍰!
The Solution 🎯
To convert the date format selectively, we'll follow a three-step process:
Parsing the Original Date: We'll utilize PHP's
strtotime()
function to convert theyyyy-mm-dd
string into a Unix timestamp. This function is perfect for extracting a timestamp from a given date string.Formatting the Date: After obtaining the timestamp, we'll leverage PHP's
date()
function to format it into the desireddd-mm-yyyy
format.Putting It All Together: Finally, we'll combine both steps to create a streamlined function that you can reuse with ease.
Let's take a closer look at the code snippets to accomplish each step.
1. Parsing the Original Date
$originalDate = "2022-10-15";
$timestamp = strtotime($originalDate);
In the snippet above, we declare a variable $originalDate
to hold our date string. Replace it with the actual date string you want to convert. The strtotime()
function takes care of converting the string into a Unix timestamp, which we store in the variable $timestamp
.
2. Formatting the Date
$formattedDate = date("d-m-Y", $timestamp);
Here, we use the date()
function with a format string "d-m-Y"
. Adjust the format accordingly if you desire a different representation. The second argument, $timestamp
, is where we bring in our converted timestamp to produce the desired format.
3. Putting It All Together
function convertDateFormat($originalDate) {
$timestamp = strtotime($originalDate);
return date("d-m-Y", $timestamp);
}
// Call the function with your original date to get the formatted date
$formattedDate = convertDateFormat("2022-10-15");
With this final step, we encapsulate the conversion logic within a function called convertDateFormat()
. By passing your original date as a parameter, you'll receive the formatted date as a return value. Simply call the function whenever you need to perform the conversion, and voilà!
Time to Shine ✨
Congratulations, brave coder! You've now equipped yourself with the knowledge and code snippets necessary to convert a date format in PHP. Go ahead and implement this solution in your projects, impressing your colleagues with your newfound date conversion prowess. 😎💪
If you found this guide helpful or have any questions, feel free to share your thoughts in the comments below. Let's keep the conversation going! And don't forget to share this blog post with fellow developers who might find it beneficial. Happy coding! 🖥️📝🤝
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
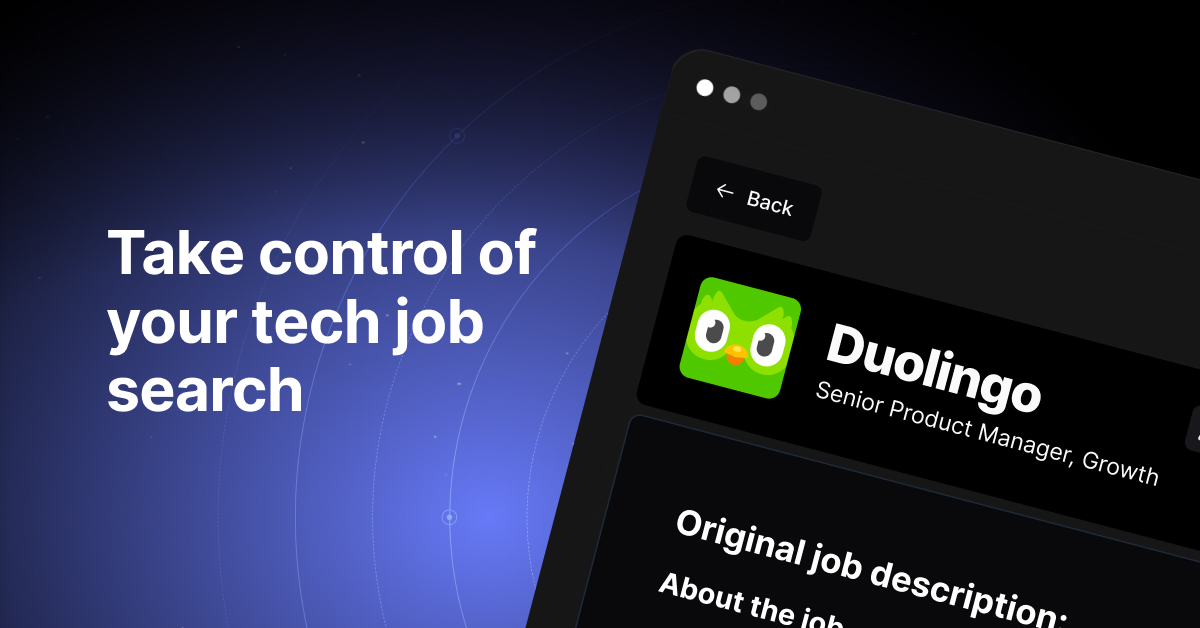