Check if PHP session has already started
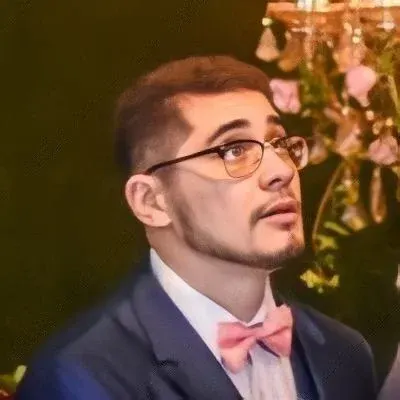
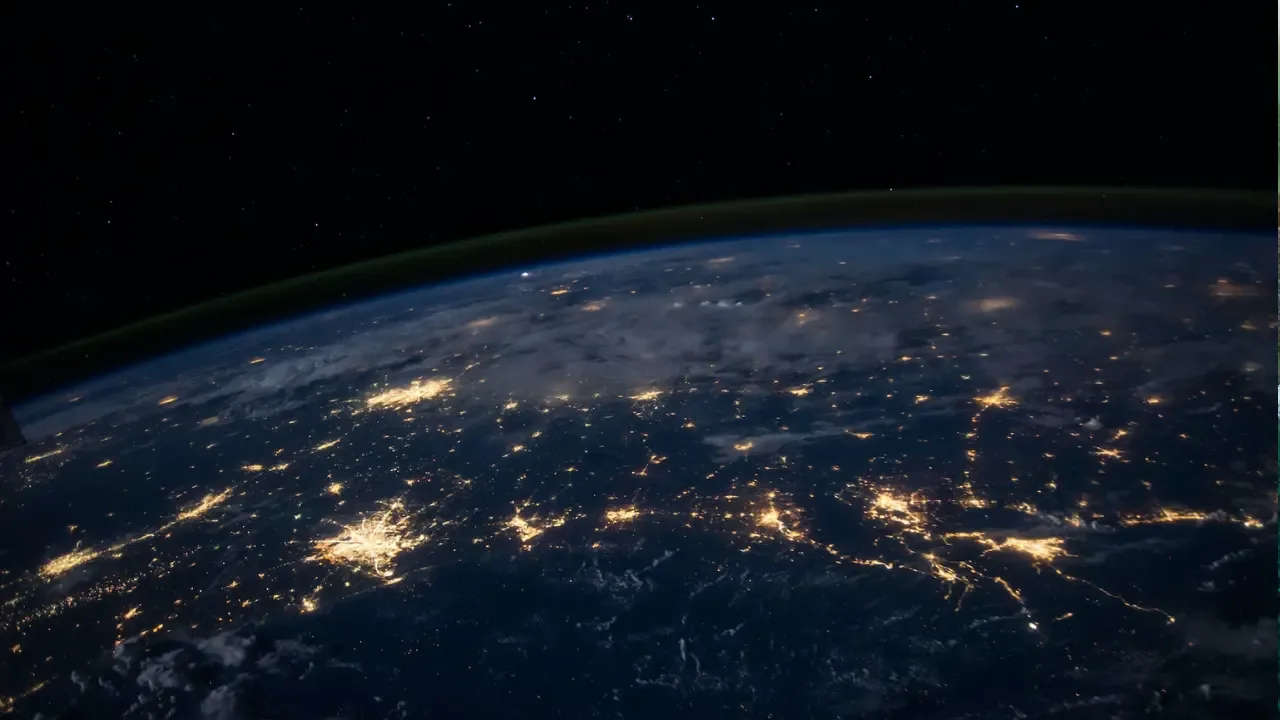
🤔 How to Check If PHP Session Has Already Started?
Picture this scenario: You have a PHP file that can be called from either a page with an active session or a page without an active session. When you include session_start()
in this file, you sometimes encounter an error message stating that the session has already started. 😱 To tackle this issue, you implemented the following code snippet:
if (!isset($_COOKIE["PHPSESSID"])) {
session_start();
}
However, this solution ended up throwing the warning message: "Notice: Undefined variable: _SESSION". 😫 Now, you're left wondering if there's a better way to check if a session has started and whether using @session_start
will silence the warnings. Let me guide you through this conundrum! 🚀
🛑 The Problem with Using isset($_COOKIE["PHPSESSID"])
The code snippet you used initially tries to check if the cookie key "PHPSESSID"
is set. If the cookie is not set, it proceeds with starting the session using session_start()
. Seems reasonable, right? But here's the catch: 🎣
If the cookie is not set, it DOES NOT guarantee that the session has not been started. 🤯 It only indicates whether the session ID has been received via a cookie or not. Remember, the session ID is not solely dependent on cookies; it can also be passed through URLs or forms. So, relying solely on this check is unreliable. 😵
✔️ A Reliable Solution: session_status()
To overcome this issue and accurately establish whether a session has started, PHP provides the session_status()
function. This function returns an integer representing the current session status. Let's break down the possible return values:
PHP_SESSION_DISABLED
: Indicates that sessions are disabled on the server.PHP_SESSION_NONE
: Indicates that sessions are enabled but no session exists.PHP_SESSION_ACTIVE
: Indicates that sessions are enabled and a session exists.
By leveraging this function, you can effectively check if a session has already started, regardless of the method used (cookie, URL, form, or others). 🙌
To implement this solution, modify your code snippet as follows:
if (session_status() === PHP_SESSION_NONE) {
session_start();
}
Now, let's dive into the advantages of using this approach!
💪 Advantages of Using session_status()
By utilizing session_status()
, you can reap several benefits:
Reliability: Unlike checking a specific cookie,
session_status()
provides an accurate representation of the session's current state, regardless of the method used to pass the session ID.Compatibility: This method is compatible with various PHP versions, including versions that do not support the
$_COOKIE
superglobal (such as CLI scripts).Ease of Use: The
session_status()
function is extremely easy to implement. It returns a simple integer value, enabling straightforward comparisons and checks.Future-proofing: As PHP evolves, so does the method of session management. Relying on a function dedicated to determining session status ensures your code's longevity and adaptability. 🚀
📣 Call-to-Action: Level Up Your Session Handling!
Congratulations, you're now armed with the knowledge of effectively checking if a PHP session has already started! 🎉 By using session_status()
, you're ensuring reliable session management, compatibility, ease of use, and future-proofing. 💪
So, why stop here? Put your newfound skills to the test by implementing this approach in your own projects. Share your experience with us in the comments section below! 📝 Let's engage in a discussion and learn together. Don't forget to hit the share button to spread the session-handling wisdom with your fellow developers! 🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
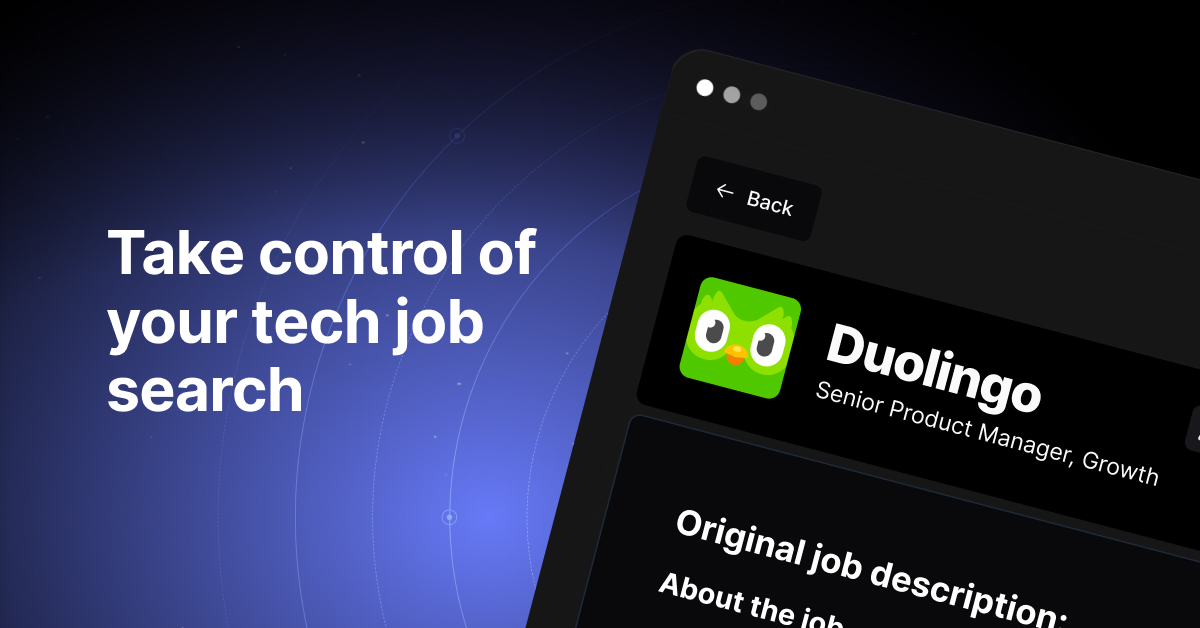