Call a REST API in PHP
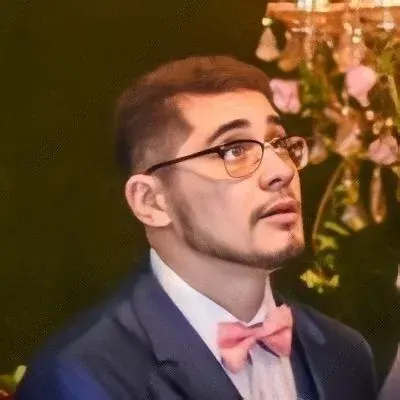
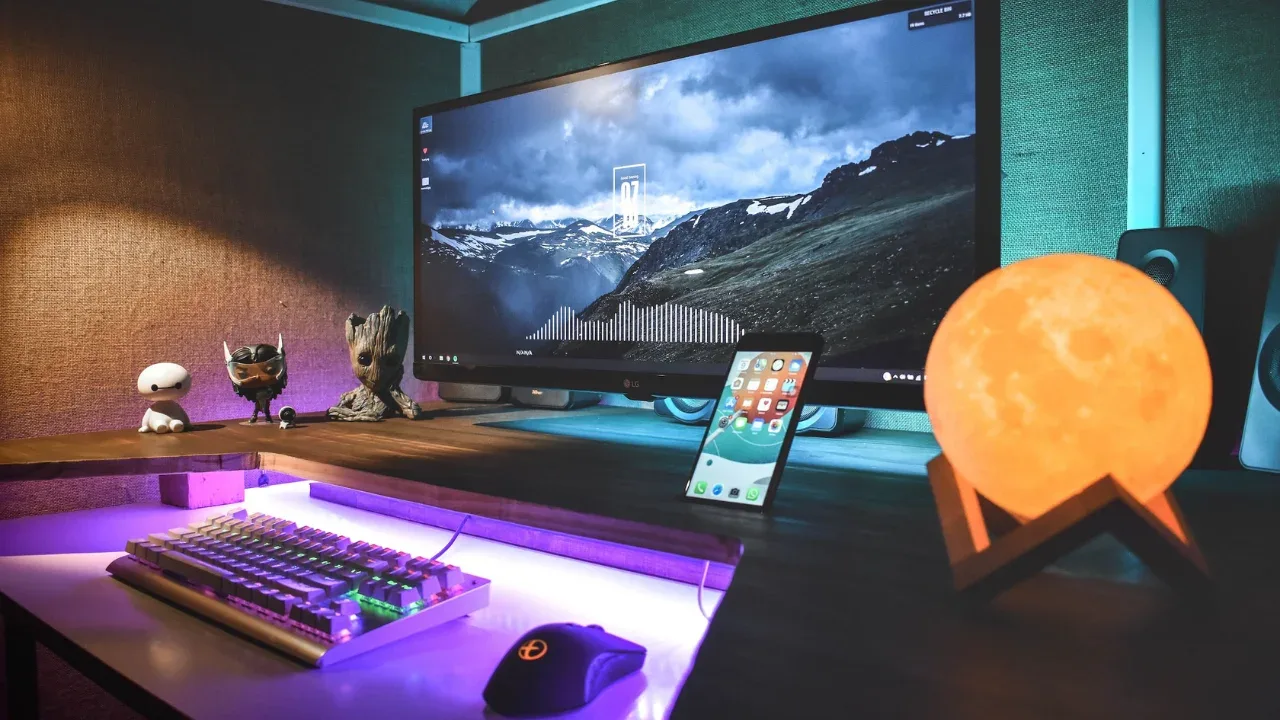
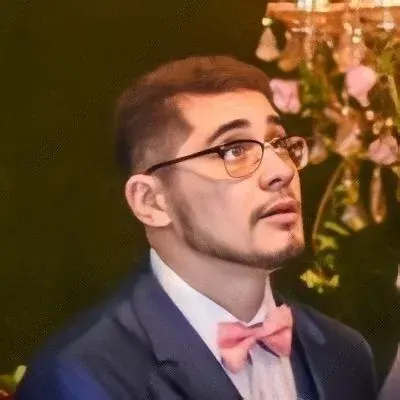
📝 Title: Calling a REST API in PHP: Your Ultimate Guide
So, you've been given a shiny new REST API to work with, but you're feeling a bit lost on how to make it work in PHP. You've searched high and low for proper documentation, only to be met with expired tutorials and incomplete guides. Fear not! In this blog post, we'll explore common issues when calling a REST API, provide easy solutions, and equip you with the knowledge to master the art of API calling in PHP. Let's dive in! 💪
Understanding REST APIs
Before we jump into the nitty-gritty of calling a REST API in PHP, let's quickly cover the basics. REST (Representational State Transfer) is an architectural style for building web services, allowing communication between different systems over the web.
A REST API typically communicates using HTTP methods such as GET, POST, PUT, DELETE, etc., and responses are generally in JSON or XML format. The API endpoints correspond to specific resources like users, products, or orders.
Common Issues and Solutions
Issue 1: Lack of Documentation
You're not alone in facing this challenge. Many APIs come with limited or outdated documentation, making it frustrating to figure out how to call them. But worry not! There are a few steps you can take:
Start with the basics: Take a look at the API endpoints and their parameters. Note down any required headers or authentication methods.
Explore the API: Try using tools like Postman or cURL to interact with the API endpoints and observe the responses.
Reach out for help: If you're still struggling, search for developer communities or discussion forums related to the API. Often, other developers have faced similar problems and might offer solutions or workarounds.
Issue 2: Choosing the Right PHP Library
When it comes to calling REST APIs in PHP, you have several options to choose from. Let's explore some popular libraries:
cURL: This is a widely-used PHP library for making HTTP requests. It provides a lot of flexibility and control over the API calls. Here's a basic example:
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $apiUrl);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
Guzzle: Guzzle is a powerful HTTP client library built specifically for PHP. It offers a more convenient and object-oriented approach to make API calls. Here's an example using Guzzle:
$client = new GuzzleHttp\Client();
$response = $client->request('GET', $apiUrl);
HTTP Plug: HTTP Plug is a lightweight and extensible HTTP client interface for PHP. It allows you to use various HTTP client libraries interchangeably. Here's a basic example:
$httpClient = new Http\Client\HttpClient();
$request = new Http\Message\Request('GET', $apiUrl);
$response = $httpClient->sendRequest($request);
Choose the library that best suits your project's requirements and coding style.
Issue 3: Handling Authentication
Authentication is crucial when making API calls. Depending on the API, you might need to include authentication credentials like API keys, tokens, or OAuth. Here's an example using cURL and an API key:
$headers = [
'Authorization: Bearer YOUR_API_KEY',
];
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $apiUrl);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
$response = curl_exec($ch);
curl_close($ch);
Make sure to refer to the API documentation for the specific authentication method required.
🙌 Call-to-Action: Share Your API Calling Experience
Now that you've learned the essentials of calling a REST API in PHP, it's time to put your knowledge into action. Share your experiences in the comments below! Did you face any challenges? How did you overcome them? Help the community grow stronger by sharing your tips and tricks.
Remember, mastering API calling takes time and practice. Code, experiment, and explore different APIs to become a pro in no time!
Happy coding! 💻🚀