Best way to do multiple constructors in PHP
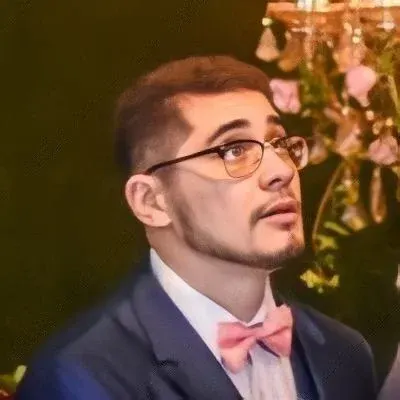
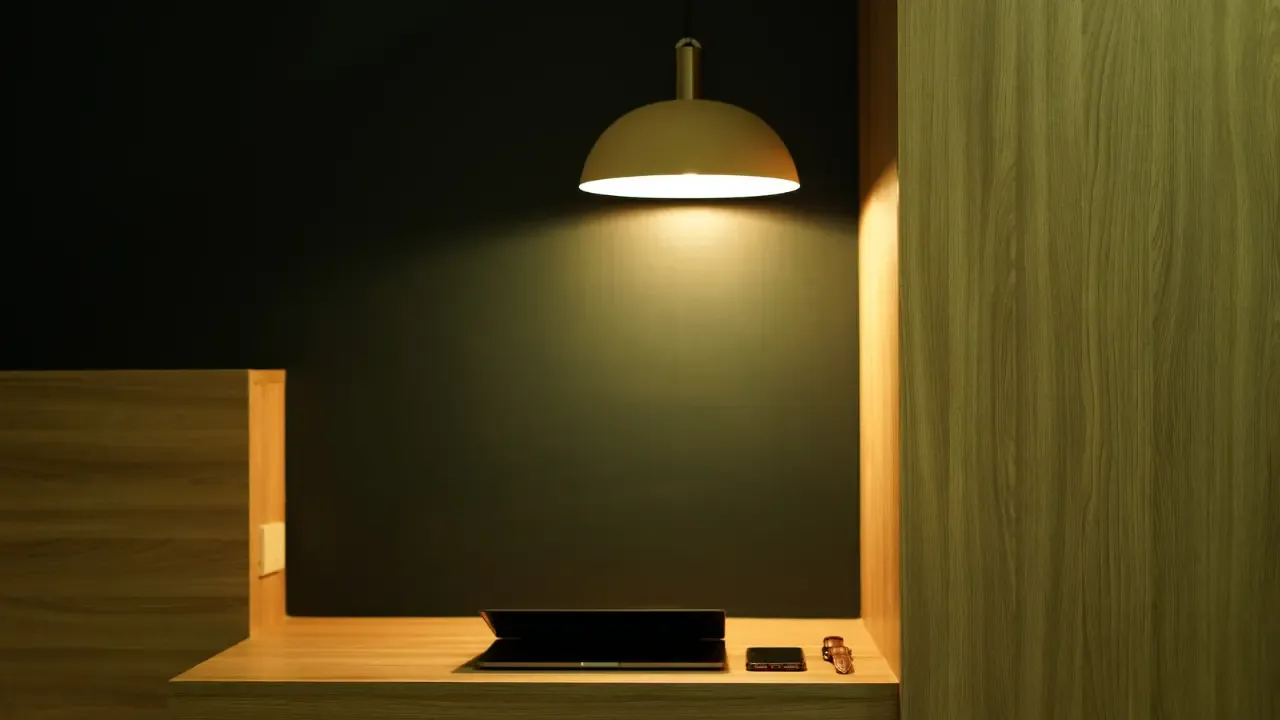
💡 Easy Solutions for Multiple Constructors in PHP
Have you ever found yourself in a situation where you need to create multiple constructors in a PHP class with different argument signatures? 🤔
In PHP, you can't define multiple __construct
functions with unique argument signatures. This limitation can be frustrating, especially when you have different ways of initializing your object. But fear not! We've got you covered with some easy solutions. 🚀
1️⃣ Solution: Method Overloading Using Default Parameters
One way to mimic multiple constructors is by using method overloading with default parameters. Here's how you can do it:
class Student
{
protected $id;
protected $name;
// etc.
public function __construct($id = null, $row_from_database = null)
{
if (!is_null($id)) {
$this->id = $id;
// other members are still uninitialized
} elseif (!is_null($row_from_database)) {
$this->id = $row_from_database->id;
$this->name = $row_from_database->name;
// etc.
}
}
}
By specifying default parameters for $id
and $row_from_database
, you can create an instance of the Student
class using either $id
or $row_from_database
. It also allows you to use the constructor without any arguments.
2️⃣ Solution: Factory Method
Another approach is to use a factory method to create instances of the class based on the input provided. Here's an example:
class Student
{
protected $id;
protected $name;
// etc.
public static function createFromId($id)
{
$student = new self();
$student->id = $id;
// other members are still uninitialized
return $student;
}
public static function createFromRow($row_from_database)
{
$student = new self();
$student->id = $row_from_database->id;
$student->name = $row_from_database->name;
// etc.
return $student;
}
}
Using factory methods, you can create instances of the Student
class with specific initialization parameters. This approach provides flexibility and allows you to handle different initialization scenarios easily.
3️⃣ Solution: Method Chaining
Method chaining can be a handy technique when you want to initialize multiple properties of an object. Here's an example of how you can implement it:
class Student
{
protected $id;
protected $name;
// etc.
public function setId($id)
{
$this->id = $id;
return $this;
}
public function setName($name)
{
$this->name = $name;
return $this;
}
// other setter methods
public static function create()
{
return new self();
}
}
With method chaining, you can create multiple setters in your class, allowing you to set individual properties in a fluent and expressive way:
$student = Student::create()->setId($id)->setName($name);
The create()
method returns a new instance of the class, and subsequent method calls set properties and return the object itself, enabling chaining.
📣 Call-to-Action: Share Your Thoughts and Ideas!
Now that you have learned a few solutions to handle multiple constructors in PHP, it's time to put them to use! Which approach resonates with you the most? Do you have any other cool techniques to share? Let us know in the comments below! 👇
Let's keep the conversation going and help each other become better PHP developers! 🌟
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
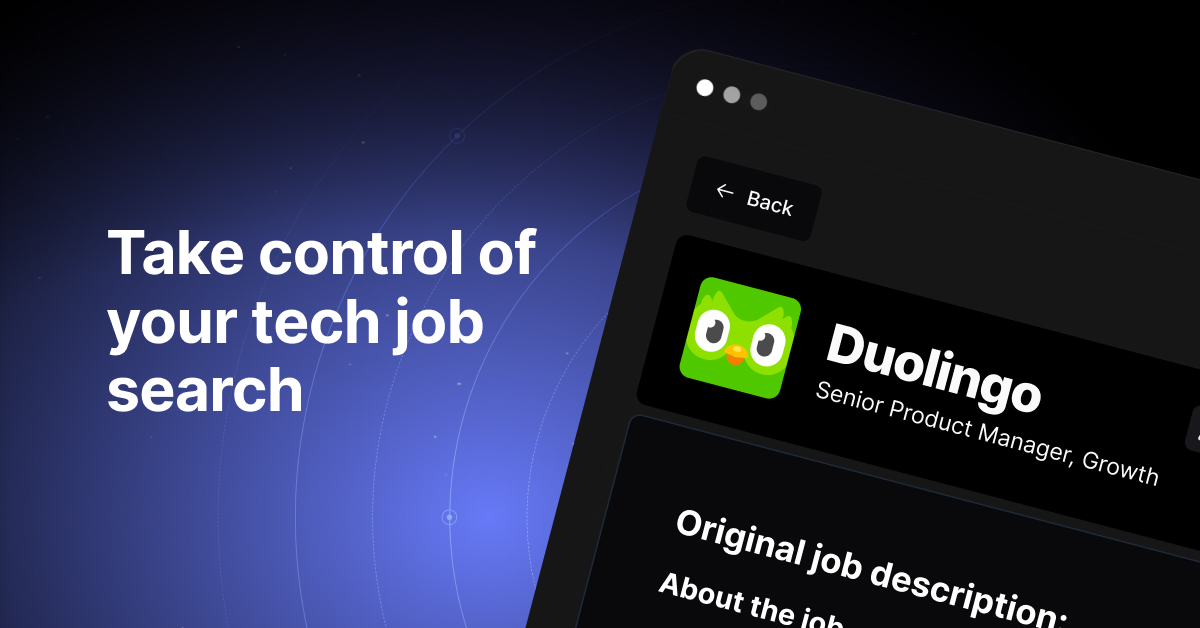