Automatically deleting related rows in Laravel (Eloquent ORM)
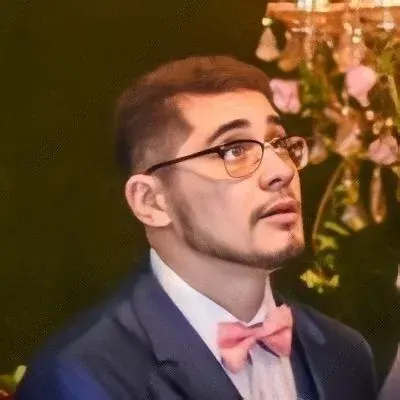
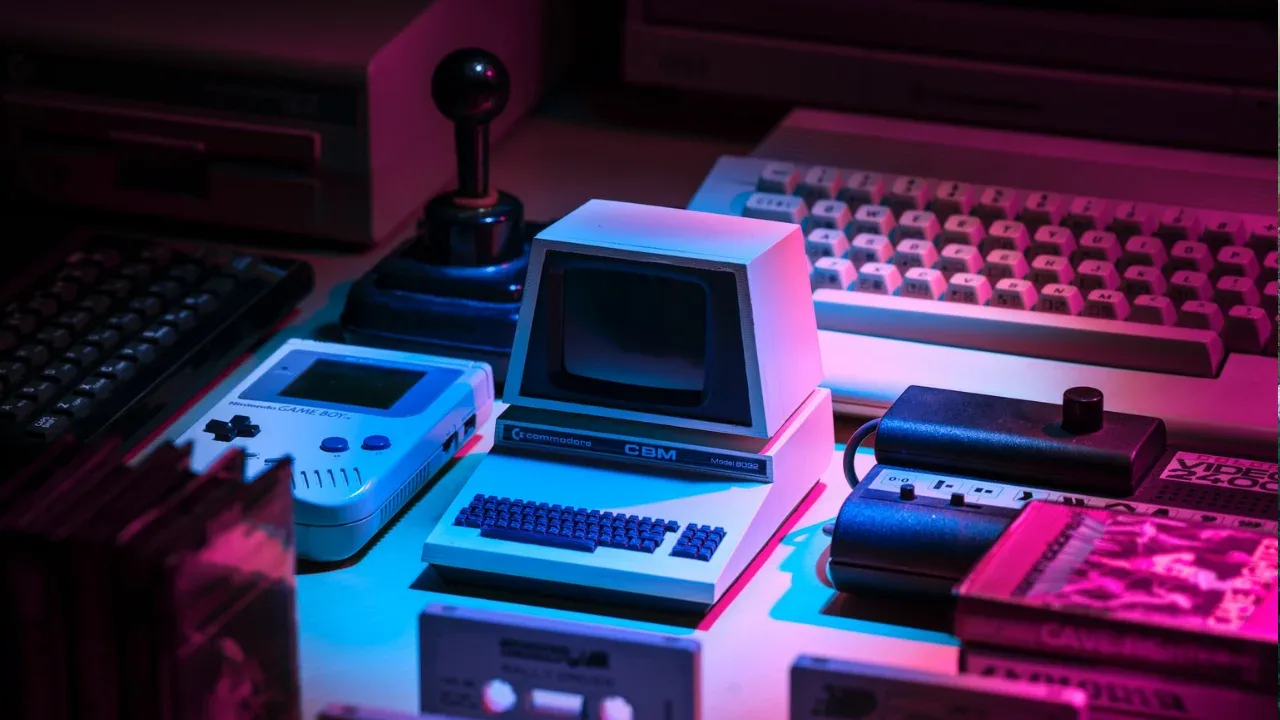
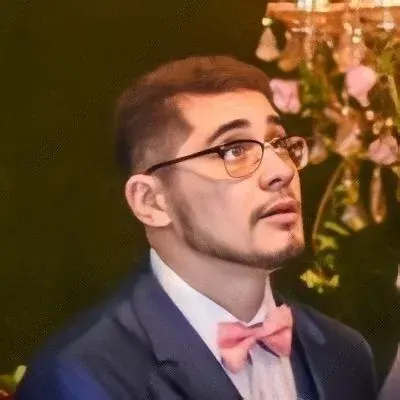
📝🧐💡🚀💥
Title: Say Goodbye to Related Rows Automatically in Laravel: Eloquent ORM Guide
Intro: Are you tired of manually deleting related rows in Laravel every time you delete a parent row? 😫🔌 Well, fret no more! In this guide, we'll explore how to automatically delete related rows using Laravel's Eloquent ORM.💥🎉
Problem Statement:
When deleting a row using the basic syntax $user->delete();
, you might be wondering if there's a way to attach a callback or method so that it automatically deletes related rows too. For instance, can we make it delete related photos using $this->photo()->delete();
automatically, preferably within the model class? 🤔📉
Solution Approach: Fortunately, Laravel provides a simple solution through its Eloquent ORM. Let's dive into three steps to automatically delete related rows. 📚🔍
1️⃣ Defining Relationship in the Model: First, make sure you have defined the relationship between the parent and related rows in your model class. For example, let's assume a one-to-many relationship where a user has many photos.
class User extends Model
{
public function photos()
{
return $this->hasMany(Photo::class);
}
}
2️⃣ Setting Up Cascade Deletion:
Next, leverage Laravel's onDelete
method to set up cascade deletion. Open the model representing the parent row (in this case, the User
model) and define the relationship using the onDelete
method.
class User extends Model
{
public function photos()
{
return $this->hasMany(Photo::class)
->onDelete('cascade');
}
}
3️⃣ Triggering Automatic Deletion:
Now, whenever you delete a user, Laravel's Eloquent ORM automatically deletes all related photos, thanks to the cascade deletion setup. You can use the basic syntax $user->delete();
to delete the parent row, and it will delete all related photos without the need for additional code. ✂️📸
$user->delete(); // Related photos will be automatically deleted 🔄
What if you want to delete the related rows independently? Well, you can still do that. Just call the delete
method on the relationship itself.
$user->photos()->delete(); // Deletes only related photos 🔒📸
Conclusion: Gone are the days of manually deleting related rows in Laravel. With Laravel's Eloquent ORM, you can effortlessly set up cascade deletion, ensuring that associated rows get deleted automatically. 🕒🔥😌
Now it's your turn to simplify your codebase and say farewell to those rows you don't need anymore! Start using automatic deletion in Laravel today! 🥳💻
Have you encountered any other challenges or questions related to Laravel or web development in general? Share them in the comments below, and let's discuss! 💬👇
#laravel #eloquentORM #automatedDeletion #webdevelopment #php #laraveltips