Add a custom attribute to a Laravel / Eloquent model on load?
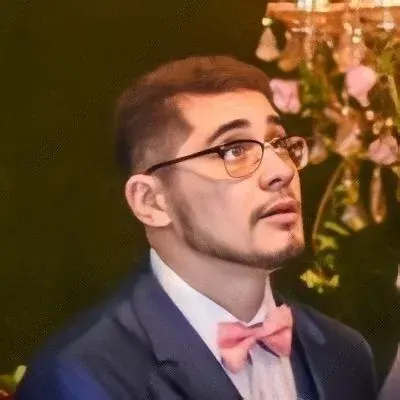
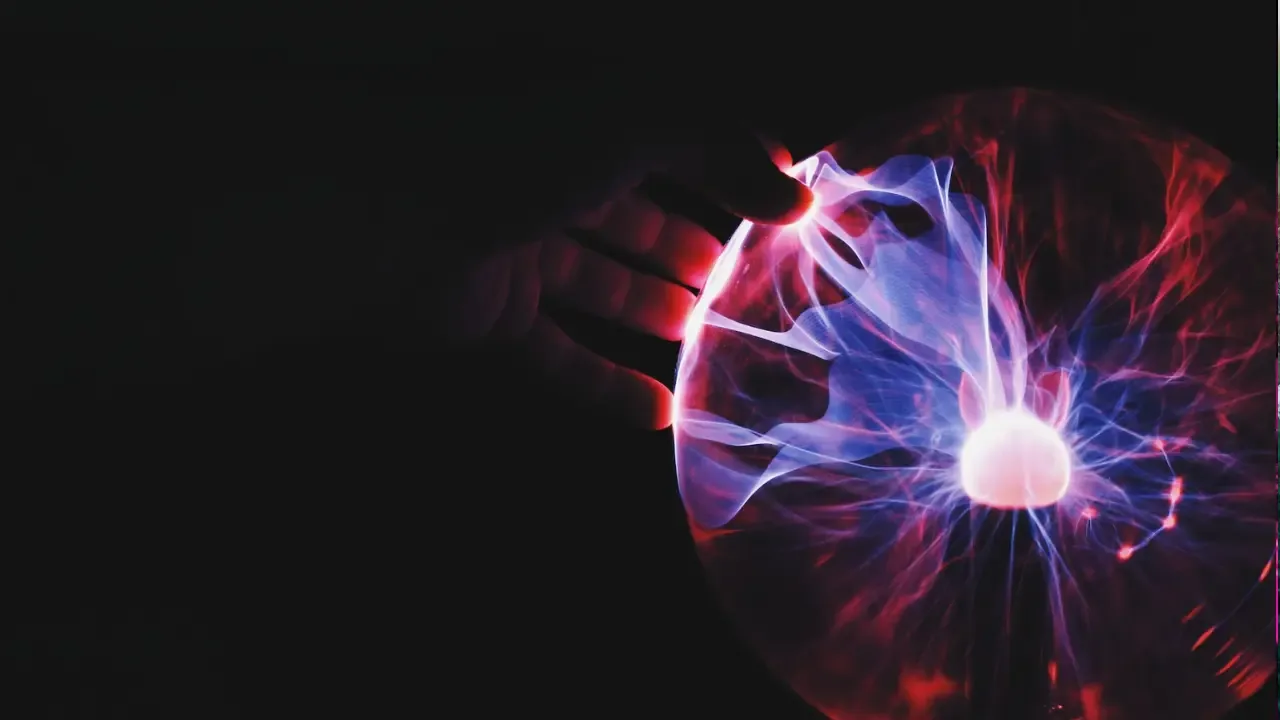
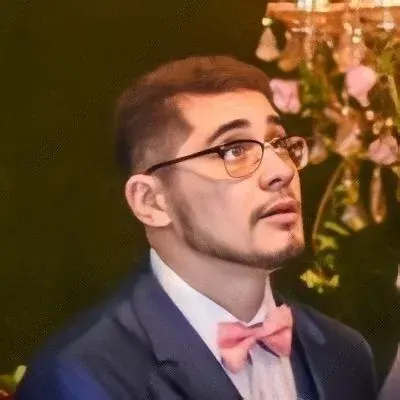
š Blog Post: Adding a Custom Attribute to a Laravel/Eloquent Model on Load
š Hey there, fellow Laravel developer! Have you ever wanted to add a custom attribute to a Laravel/Eloquent model when it is loaded? Like, without having to use a loop?
We've got your back! In this blog post, we'll address this common issue and provide you with an easy solution. Let's dive right in! šŖ
š” Understanding the Problem
So, you want to add a custom attribute to your Laravel/Eloquent model without the need for a loop. We totally get the frustration! Currently, you might have something like this in your controller:
public function index()
{
$sessions = EventSession::all();
foreach ($sessions as $i => $session) {
$sessions[$i]->available = $session->getAvailability();
}
return $sessions;
}
You wish there was a way to omit the loop and have the 'available' attribute already set and populated. You've tried using some of the model events described in the documentation, but no luck so far. We're here to help you out! šŖ
š The Solution: Accessors and Mutators
To achieve your desired result, you can make use of Laravel's accessors and mutators. Accessors allow you to get the value of an attribute dynamically, while mutators allow you to set the value of an attribute dynamically. Here's how you can implement them in your model:
Open your Laravel/Eloquent model file (e.g.,
EventSession.php
).Add a new method, let's call it
getAvailableAttribute
, inside your model class:
public function getAvailableAttribute()
{
return $this->getAvailability();
}
Now, whenever you access the
available
attribute on your model instance, Laravel will automatically call this method and retrieve the result. Cool, right? šThat's it! You can now omit the loop in your controller and simply return the
$sessions
.
public function index()
{
return EventSession::all();
}
š And there you have it! Your available
attribute will be included in the JSON response. No loops, no worries! š
š£ Call-to-Action: Share Your Experience!
We hope this blog post has helped you add a custom attribute to your Laravel/Eloquent model without the need for a loop. If you found this solution useful or have any other cool Laravel tips and tricks, we'd love to hear from you! Share your thoughts in the comments section below, and let's empower the Laravel community together! š¬š
So go ahead, implement this solution, and let us know how it worked for you! Happy coding! š