Access Controller method from another controller in Laravel 5
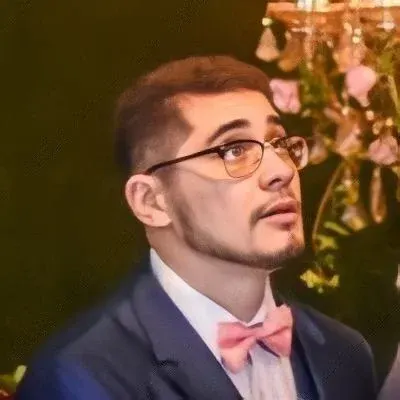
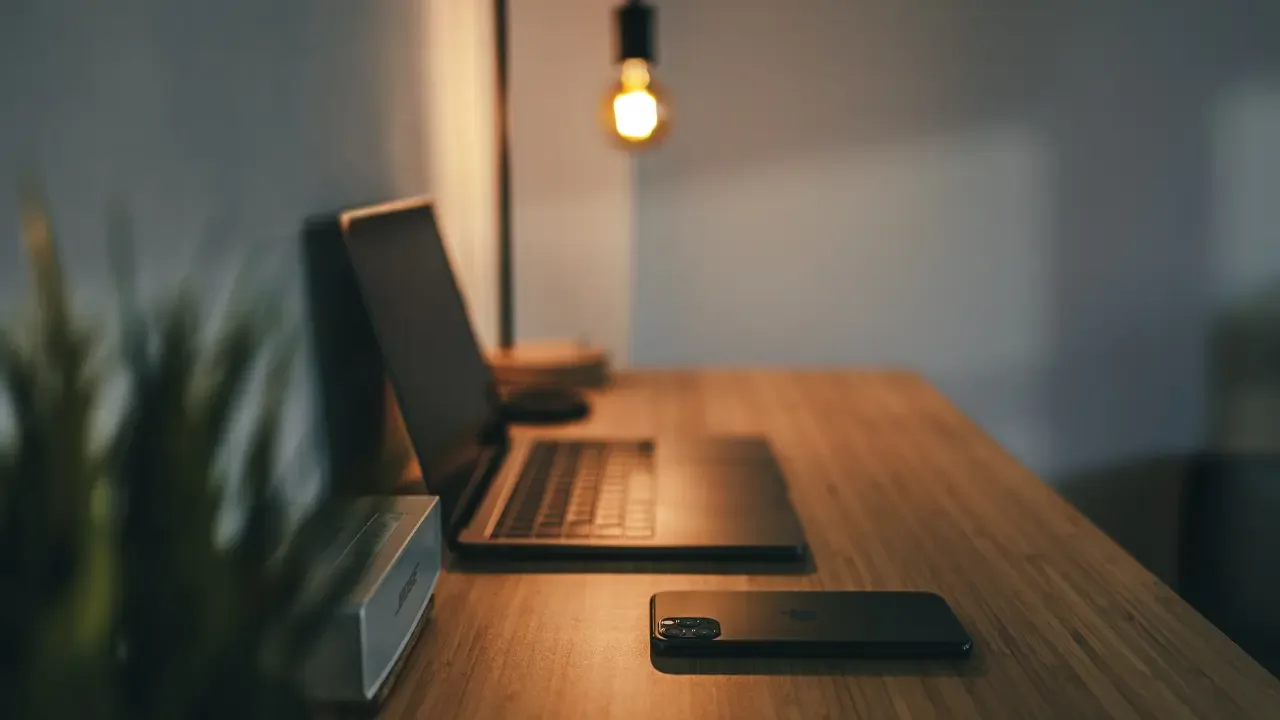
š Title: How to Access Controller Method from Another Controller in Laravel 5
š Hey there, tech enthusiasts! Today, we're diving into a common issue that developers face: accessing a controller method from another controller in Laravel. š
šÆ Problem: The Context
In our scenario, we have two controllers: SubmitPerformanceController
and PrintReportController
. The latter has a handy method called getPrintReport
. The challenge is how to access this method from SubmitPerformanceController
. Let's find a solution together! š
š” Solution: Bridging the Gap
Fortunately, Laravel provides an elegant way to access methods within a controller using dependency injection. By leveraging this feature, we can seamlessly call the getPrintReport
method from SubmitPerformanceController
. š
Here's an example of how you can achieve this:
Import the
PrintReportController
at the top ofSubmitPerformanceController
:
use App\Http\Controllers\PrintReportController;
Create a constructor method in
SubmitPerformanceController
:
public function __construct(PrintReportController $printReportController)
{
$this->printReportController = $printReportController;
}
Access the desired method using the controller instance:
public function someMethod()
{
// Call the getPrintReport method
$printReport = $this->printReportController->getPrintReport();
// Do whatever you need with the result
return view('some.view', ['printReport' => $printReport]);
}
š¦ Final Touches: A Compelling Call-to-Action
This handy method of accessing controller methods from another controller can save you from unnecessary headaches in your Laravel development journey. So go ahead, give it a try, and boost your productivity levels! šŖ
š” Don't hesitate to explore Laravel's extensive documentation for more incredible features and solutions to your coding woes!
⨠Now, it's your turn! Have you ever faced a similar challenge in Laravel or any other framework? Share your experiences, tips, or questions in the comments below. Let's learn and grow together! š±
P.S. Don't forget to share this blog post with your fellow developers who might find it helpful. Happy coding! š
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
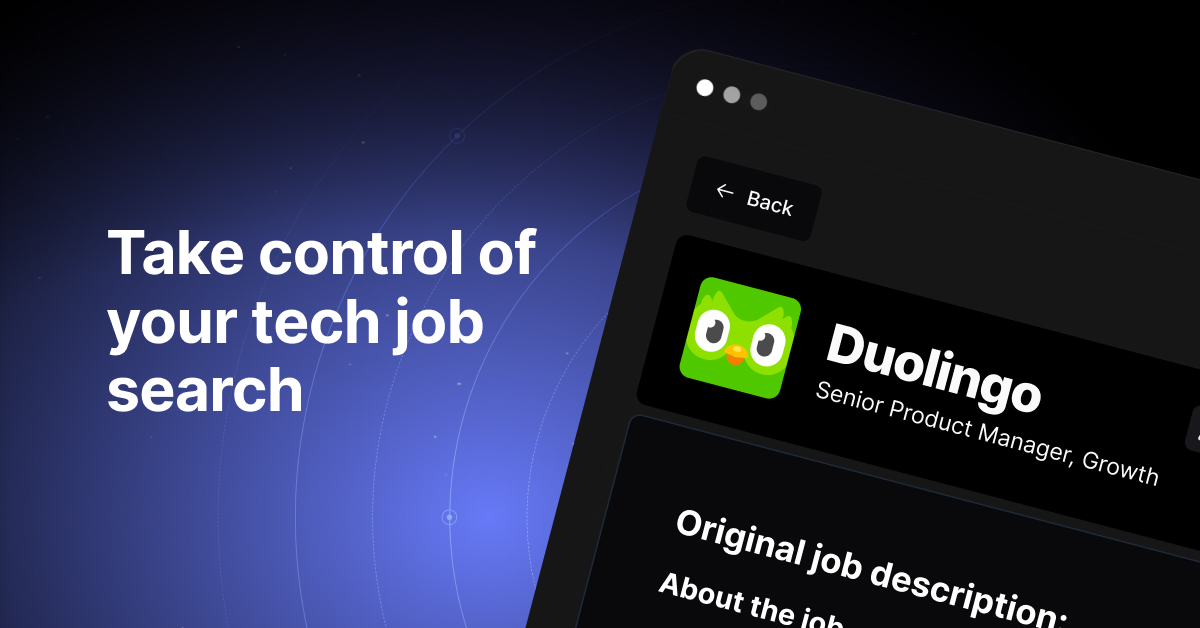