How do you test running time of VBA code?
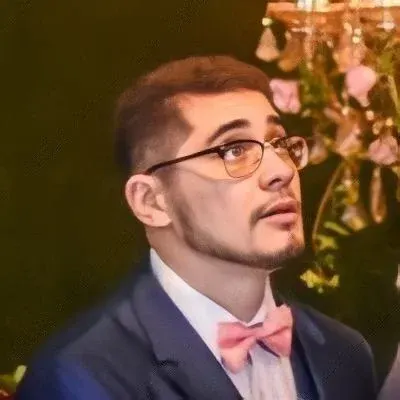
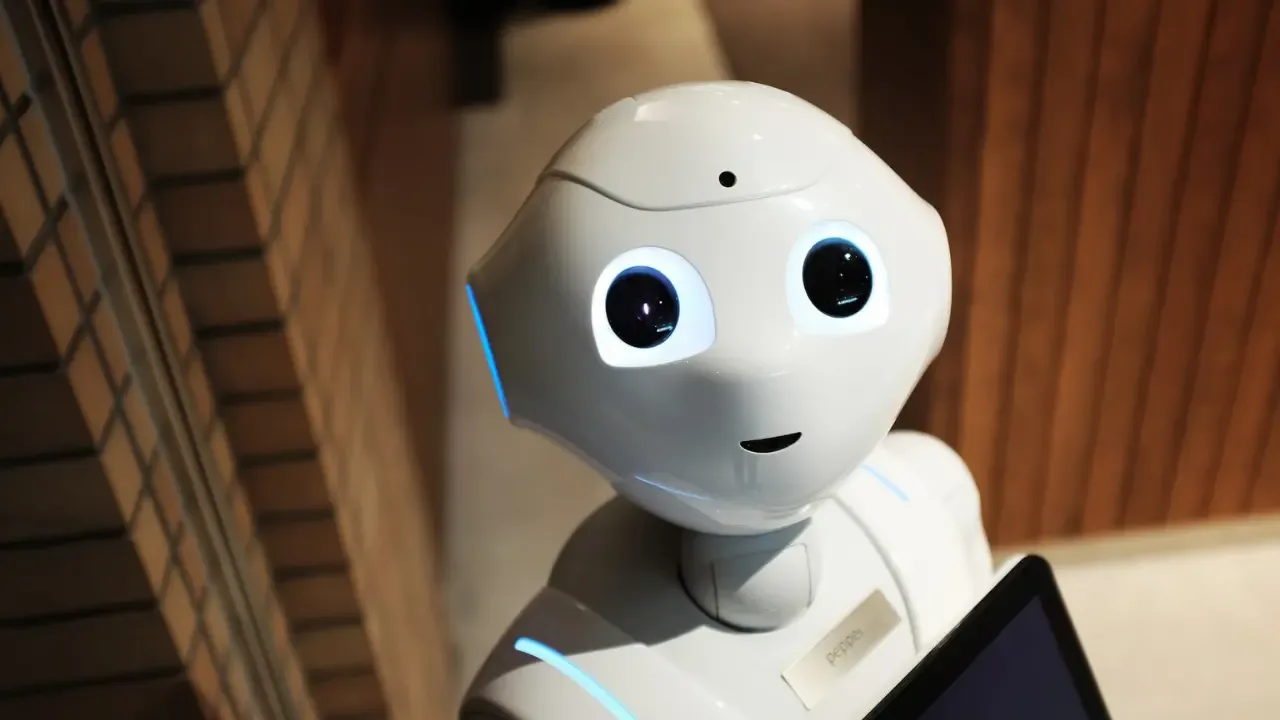
💻🕐 Testing Running Time of VBA Code: A Guide for Smarter Comparisons
Do you ever wonder how long your VBA code takes to run? 🤔 Whether you're optimizing your code or comparing the performance of different functions, measuring the running time is essential. But fear not! 💪 We've got you covered with this easy guide to testing VBA code running time. Let's dive in and make your code faster and more efficient! ⚡
The Common Issue: Track VBA Code Running Time ⏰
Many VBA developers struggle with measuring the running time of their code. Without accurate measurements, it's challenging to identify bottlenecks or evaluate the effectiveness of optimizations. Developers often ask if there's a way to wrap a function and track the time it takes to run. 🤔 Well, the answer is YES! 🎉
The Easy Solution: Time Your Code with VBA 🕑
To measure VBA code running time, we can leverage simple techniques:
1. Using the Timer Function ⏲️
VBA comes with a built-in Timer function that returns the number of seconds since midnight. By using this function, you can easily track the running time of your code. Here's an example:
Sub MeasureCodeRunningTime()
Dim startTime As Double
Dim endTime As Double
Dim elapsedTime As Double
startTime = Timer
' Your code goes here
endTime = Timer
elapsedTime = endTime - startTime
MsgBox "The code took " & elapsedTime & " seconds to run."
End Sub
In this example, we start the timer (Timer
function) before executing the code and stop it after the code execution. The elapsed time is then calculated by subtracting the start time from the end time.
2. Using GetTickCount64 API function 🌐
If you need more precise measurements, you can use the GetTickCount64 API function. This function returns the number of milliseconds since the system started. Here's an example of how to use it:
Private Declare PtrSafe Function GetTickCount64 Lib "kernel32" () As Currency
Sub MeasureCodeRunningTime()
Dim startTime As Currency
Dim endTime As Currency
Dim elapsedTime As Currency
startTime = GetTickCount64
' Your code goes here
endTime = GetTickCount64
elapsedTime = endTime - startTime
MsgBox "The code took " & elapsedTime & " milliseconds to run."
End Sub
In this example, we declare the GetTickCount64 API function using the Private Declare PtrSafe
statement. We measure the running time by storing the start and end ticks and display the results accordingly.
The Catch: Use These Measurements Wisely 🎯
While tracking the running time of your VBA code is helpful, it's crucial to use the measurements wisely. 🧐 Here are a few tips:
Compare similar code snippets or functions: Ensure that you compare functions that serve similar purposes. It wouldn't be fair to compare code that performs entirely different tasks.
Run multiple tests: One measurement may not be representative of the typical running time. Run your code multiple times and take the average to get reliable results.
Consider the underlying system: Remember that the code's running time can be influenced by various factors like system load and available resources. Take these factors into account while making comparisons.
The Compelling Call-to-Action: Share Your Experiences! ✍️💭
Now that you know how to measure the running time of your VBA code, why not give it a try? Share your experiences in the comments below! We'd love to hear about any optimizations you discovered or challenges you faced. Let's ignite a discussion and learn from each other! 🚀🔥
Remember, improving code performance is an ongoing journey. Keep experimenting, optimizing, and sharing your knowledge with fellow developers. Together, we can write faster, more efficient, and robust VBA applications! 💪💻
We hope that this guide helps you in testing the running time of your VBA code. If you found it helpful, don't forget to share it with your developer friends! 👥💚
✨ Happy coding and may your VBA code always run at lightning speed! ⚡🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
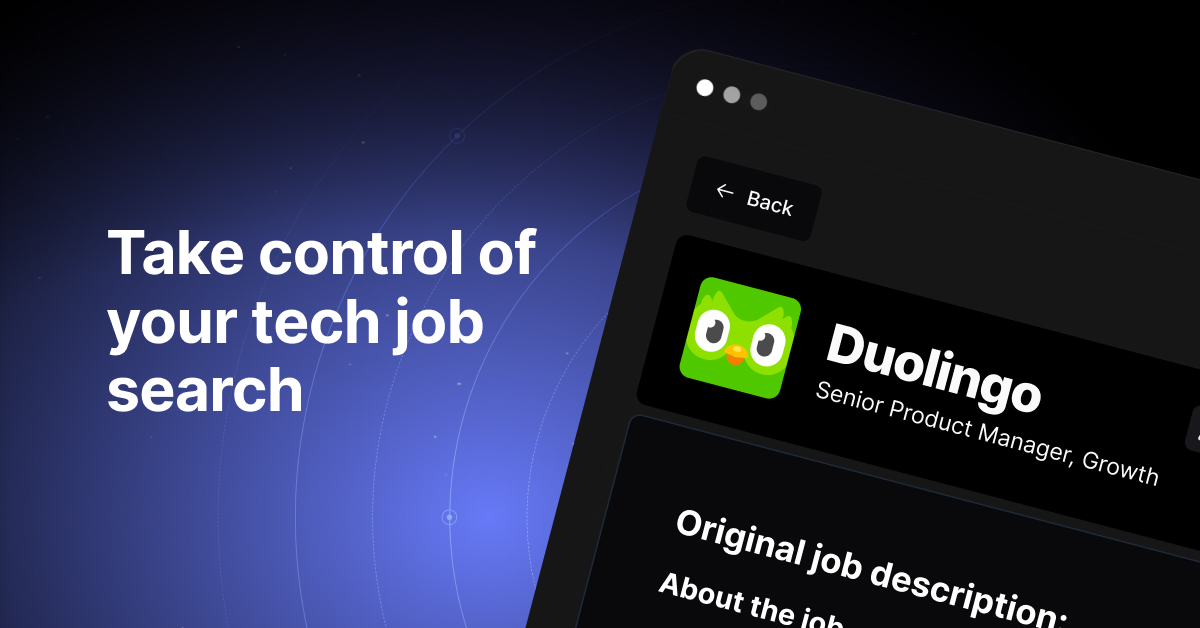