Managing relationships in Laravel, adhering to the repository pattern
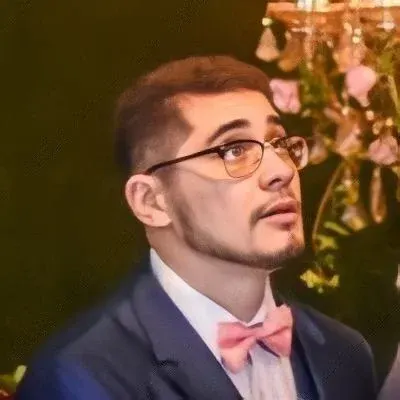
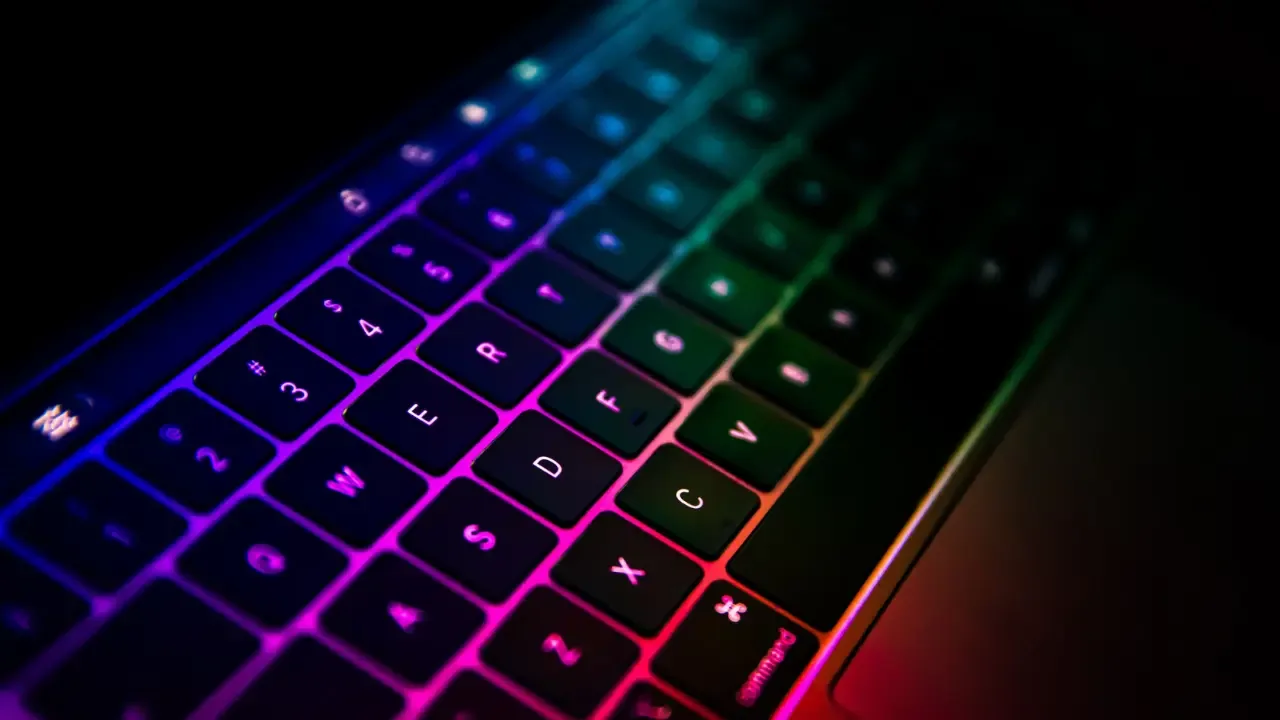
Managing Relationships in Laravel with the Repository Pattern: A Complete Guide 🚀
Are you struggling with managing relationships in your Laravel app while adhering to the repository pattern? 😫 Don't worry, we've got you covered! In this post, we'll discuss common issues, provide easy solutions, and give you a clear understanding of how to handle relationships effectively. Let's dive in! 💪
The Problem 🤔
As Laravel developers, we understand the importance of designing our apps using good design patterns. Following Taylor Otwell's advice, you have created repositories for each table in your application. But now, you're facing a challenge - how do you manage relationships between these tables? 🤷♂️
Imagine you have the following table structure:
Students: id, name
Courses: id, name, teacher_id
Teachers: id, name
Assignments: id, name, course_id
Scores (acts as a pivot between students and assignments): student_id, assignment_id, scores
You have repository classes with find, create, update, and delete methods for each of these tables. Each repository interacts with the database using an Eloquent model, following Laravel's documentation on relationships.
Everything seems to work fine until you encounter a situation where you need to create an assignment for a course and automatically create entries in the scores table for each student in the course. 📚
So, the question arises - should you communicate with different Eloquent models in repositories, or should this be done using other repositories instead? Should the logic reside in the Eloquent models themselves? Let's find out! 🕵️♀️
The Best Practice: Communication, Repositories, and Models 💡
As your Laravel app grows in size and complexity, it's crucial to maintain a clean and scalable codebase. To manage relationships effectively while adhering to the repository pattern, here's what you should do:
1. Leveraging Repositories
In the context of the repository pattern, it's recommended to communicate between repositories rather than directly accessing different Eloquent models within repositories. This promotes loose coupling and improves the maintainability of your code. 📦
In your scenario, when creating an assignment for a course, instead of directly calling the Assignment Repository, you can introduce a new method in the Course Repository that handles the creation of assignments and the associated entries in the scores table. This method can internally utilize the Assignment Repository for the assignment creation logic and communicate with the Student Repository to retrieve the list of students for the course. 🔄
class CourseRepository
{
// ...
public function createAssignmentForCourse($courseId, $assignmentData)
{
$course = $this->courseModel->find($courseId);
$assignment = $this->assignmentRepository->create($assignmentData);
$students = $this->studentRepository->getAllStudentsForCourse($courseId);
foreach ($students as $student) {
$scoreData = [
'student_id' => $student->id,
'assignment_id' => $assignment->id,
'score' => 0,
];
$this->scoreRepository->create($scoreData);
}
return $assignment;
}
// ...
}
By incorporating this approach, you maintain a separation of concerns and keep your codebase organized. 🧩
2. Using Pivot Tables for Many-to-Many Relationships
Regarding the use of the scores table as a pivot between assignments and students, it seems like you're on the right track! When dealing with many-to-many relationships, Laravel recommends utilizing pivot tables. The scores table serves this purpose efficiently, allowing you to associate students with their respective assignments. Keep up the good work! 👏
Conclusion and Your Next Steps 🎉
Managing relationships in Laravel while adhering to the repository pattern can be a tricky task. However, by leveraging the power of repositories, communicating between them, and utilizing pivot tables for many-to-many relationships, you can achieve a clean and maintainable codebase.
Now that you have a clear understanding of the best practices, it's time to implement them in your own Laravel app! Start by refactoring your code to introduce the new method in the Course Repository for creating assignments and entries in the scores table. Remember, clean and scalable code leads to happy developers and better apps! 😊
If you have any questions or would like to share your experiences, we'd love to hear from you in the comments below. Happy coding! ✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
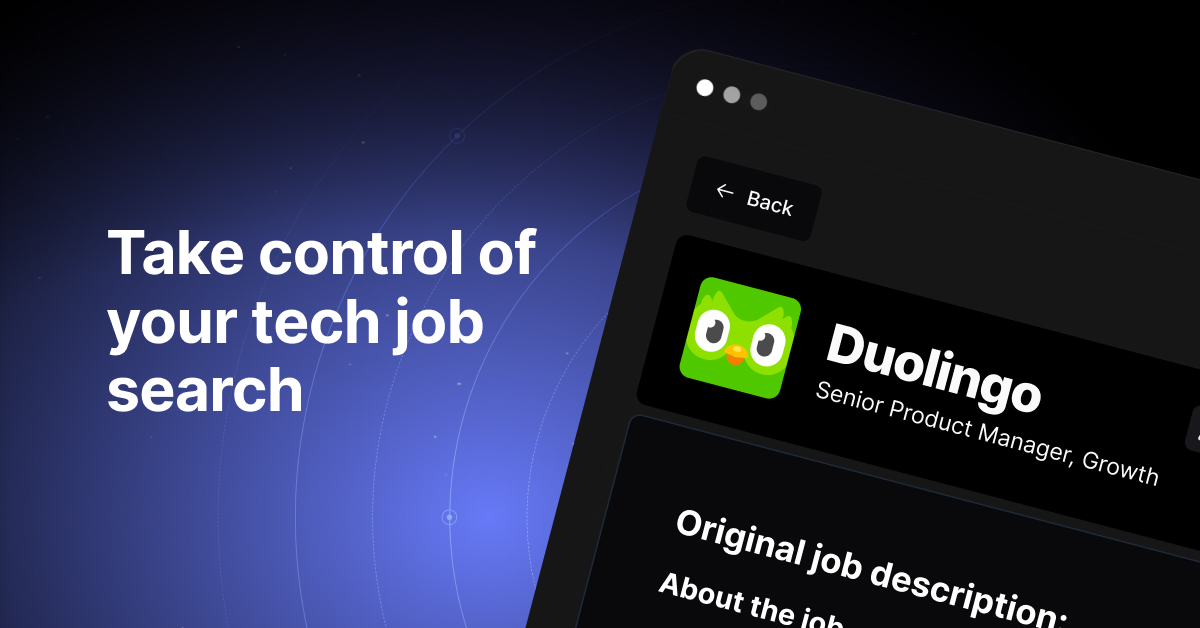