When converting a project to use ARC what does "switch case is in protected scope" mean?
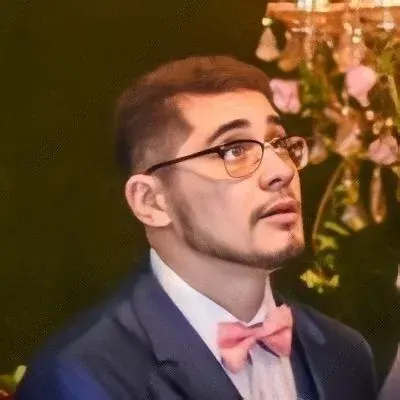
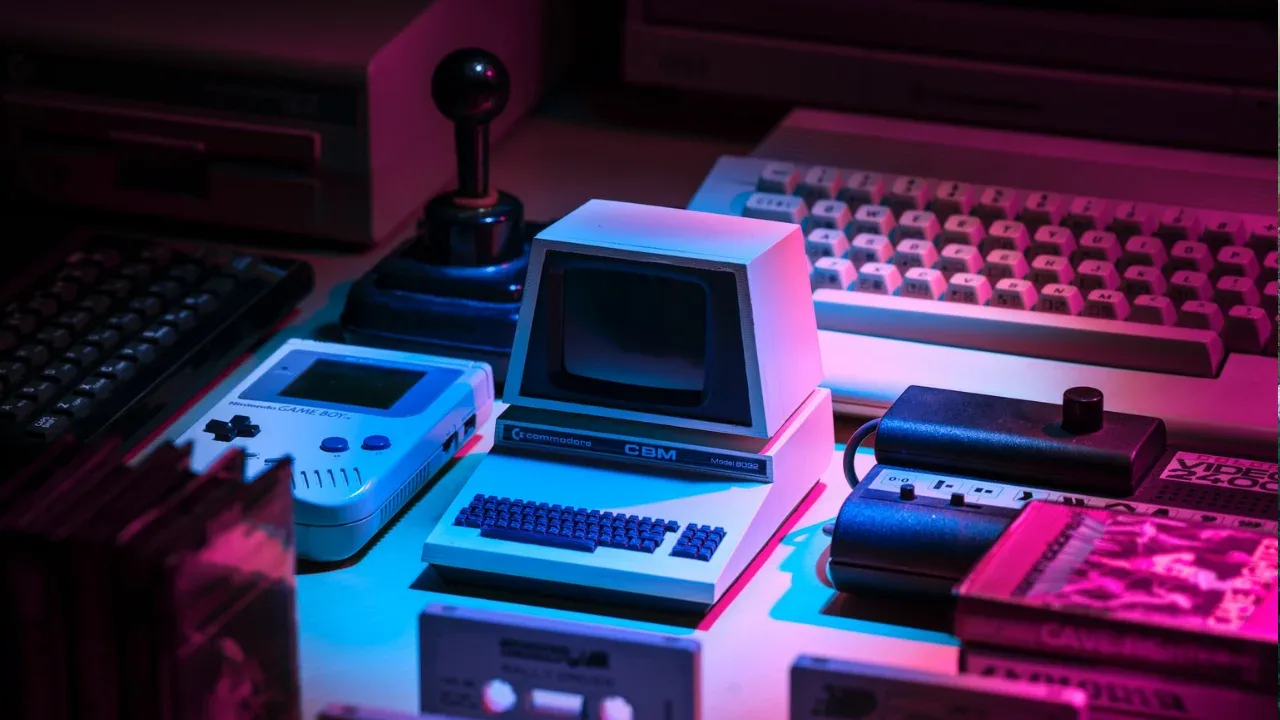
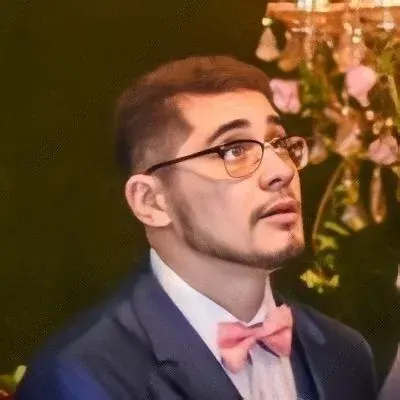
Converting a Project to Use Automatic Reference Counting (ARC)
So, you've decided to convert your project to use ARC (Automatic Reference Counting) to make memory management easier and less error-prone. Great choice! 🙌
But, during the conversion process, you encountered an error that said "switch case is in protected scope." What does that mean? And more importantly, how can you resolve it?
Let's dive into the details and find easy solutions to this problem.
Understanding the Error
First, let's understand what "switch case is in protected scope" means in the context of ARC conversion.
When you convert your project to use ARC, it analyzes your code and determines when and where to insert retain
, release
, and autorelease
statements automatically. However, there are some situations where ARC cannot safely determine the memory management behavior, and that's when this error occurs.
In the code snippet you provided, the error is marked on the default
case of the switch
statement. This means that the conversion process couldn't determine the proper memory management behavior for that particular case.
Easy Solutions
Fear not! We have some easy solutions to resolve this error and successfully convert your project to use ARC.
Solution 1: Assign nil
to the cell
variable
One way to handle this error is by assigning nil
to the cell
variable in the default
case. This ensures that any previous assignments to cell
are properly handled by ARC.
default:
CellIdentifier = @"Cell";
cell = nil;
if (cell == nil) {
cell = [[UITableViewCell alloc] initWithStyle:UITableViewCellStyleDefault reuseIdentifier:CellIdentifier];
}
break;
By assigning nil
to cell
, you let ARC handle the memory management without any ambiguity.
Solution 2: Wrap the default
case code in an @autoreleasepool
block
Another solution is to wrap the code inside the default
case in an @autoreleasepool
block. This tells ARC to handle memory management within the scope of the block, addressing any potential issues.
default:
@autoreleasepool {
CellIdentifier = @"Cell";
cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier];
if (cell == nil) {
cell = [[UITableViewCell alloc] initWithStyle:UITableViewCellStyleDefault reuseIdentifier:CellIdentifier];
}
}
break;
This solution ensures that the memory management is correctly handled within the block, regardless of the conversion process's limitations.
Concluding Thoughts
Converting a project to use ARC can greatly simplify memory management and reduce the risk of memory leaks. However, there might be situations where ARC needs some assistance to determine the correct memory management behavior.
In this case, the "switch case is in protected scope" error can be resolved by either assigning nil
to the variable or wrapping the code in an @autoreleasepool
block.
Remember, these solutions are specific to the error you encountered, but they can be applied generally to similar situations where ARC needs additional guidance.
Have you encountered any other interesting challenges during your ARC conversion process? Let us know in the comments below! 🗣️👇
And if you found this article helpful, don't forget to share it with your fellow developers. Happy coding! 💻🚀