What should my Objective-C singleton look like?
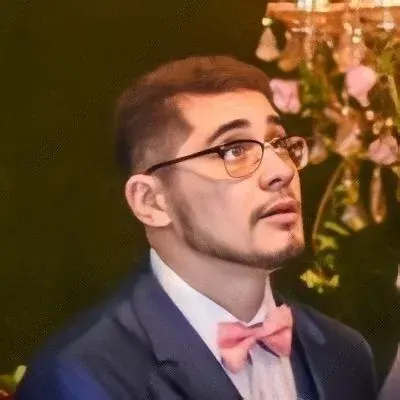
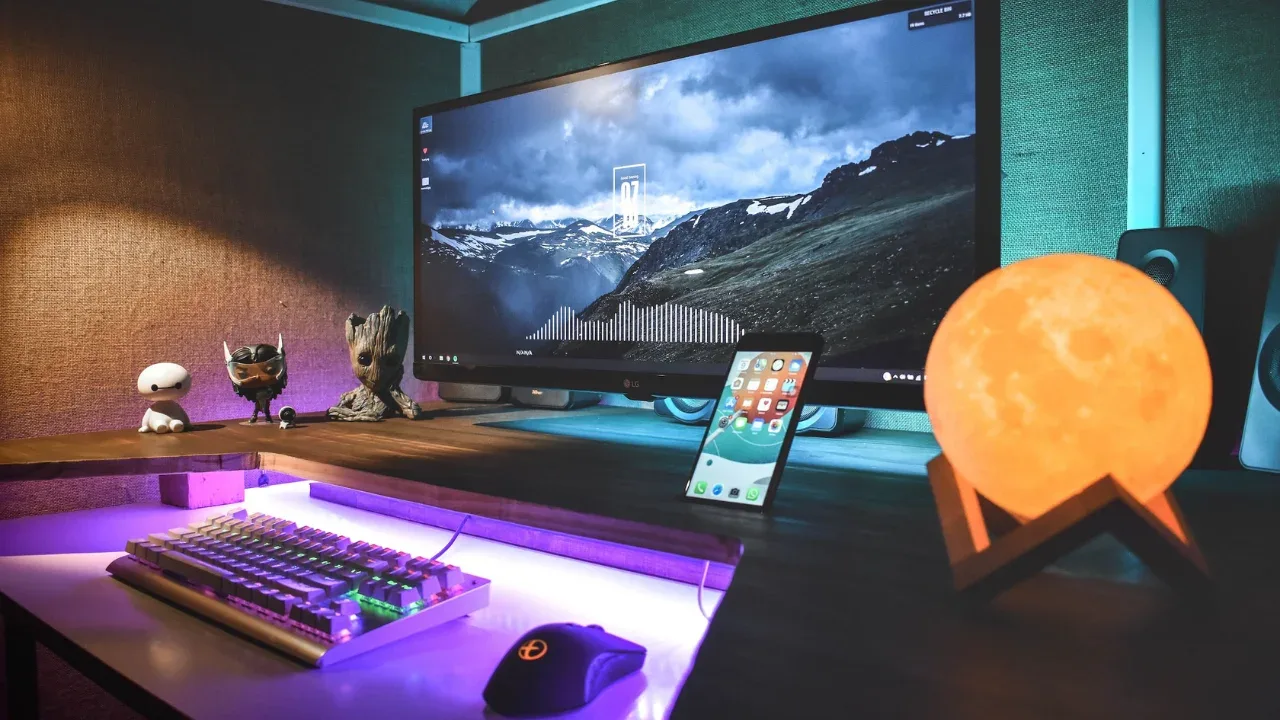
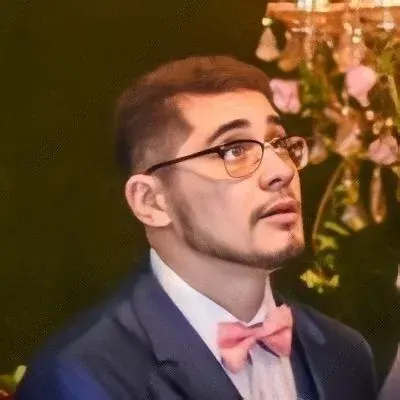
📝 Hey there tech enthusiasts! Are you wondering what your Objective-C singleton should look like? 🤔 Don't worry, I've got you covered! In this blog post, we'll address common issues, provide easy solutions, and help you level up your singleton game! 💪🏼
Let's dive right into the code snippet you provided:
static MyClass *gInstance = NULL;
+ (MyClass *)instance
{
@synchronized(self)
{
if (gInstance == NULL)
gInstance = [[self alloc] init];
}
return(gInstance);
}
At first glance, this implementation seems fine. It ensures thread-safety by using the @synchronized
directive. However, there is still room for improvement. Here's what you could do to enhance this singleton:
1️⃣ Use GCD for Thread-Safety
Instead of using @synchronized
, we can leverage the power of Grand Central Dispatch (GCD) to achieve thread-safety more efficiently. Here's an alternative implementation:
+ (instancetype)sharedInstance
{
static MyClass *sharedInstance;
static dispatch_once_t onceToken;
dispatch_once(&onceToken, ^{
sharedInstance = [[self alloc] init];
});
return sharedInstance;
}
In this implementation, we're using the dispatch_once
function from GCD to ensure that the initialization block is executed only once, regardless of the number of threads accessing it. This reduces the overhead and improves performance.
2️⃣ Improve Initialization
The previous implementation assumes that your class has an init
method. However, what if your class has a custom initializer? 🤔 To handle this situation properly, you can modify the code like this:
+ (instancetype)sharedInstance
{
static MyClass *sharedInstance;
static dispatch_once_t onceToken;
dispatch_once(&onceToken, ^{
sharedInstance = [[super allocWithZone:NULL] init];
});
return sharedInstance;
}
+ (instancetype)allocWithZone:(NSZone *)zone
{
return [self sharedInstance];
}
By overriding the allocWithZone:
method, we ensure that the singleton instance is returned regardless of how the object is allocated. This guarantees consistency and prevents accidental multiple instances.
3️⃣ Handle Singleton Deallocation
The previous implementations don't handle deallocation of the singleton instance. If you want your singleton to behave properly, even when memory is low or when it's time to clean up, you can do the following:
+ (instancetype)sharedInstance
{
static MyClass *sharedInstance;
static dispatch_once_t onceToken;
dispatch_once(&onceToken, ^{
sharedInstance = [[super allocWithZone:NULL] init];
// Register for memory warnings
[[NSNotificationCenter defaultCenter] addObserver:self
selector:@selector(resetSharedInstance)
name:UIApplicationDidReceiveMemoryWarningNotification
object:nil];
});
return sharedInstance;
}
+ (void)resetSharedInstance
{
@synchronized (self) {
sharedInstance = nil;
}
}
In this updated implementation, we not only handle memory warnings through NSNotificationCenter
, but we also reset the shared instance, if necessary, and allow it to be recreated upon the next request.
Now that you have a solid understanding of how to improve your Objective-C singleton, it's time to put these enhancements into action! Implement these changes in your code, test them thoroughly, and experience the benefits firsthand. 🚀
So what are you waiting for, fellow developers? Don't settle for mediocrity in your singleton pattern. Level up your game with these easy solutions and take your code to the next level!
📢 Remember to share your thoughts, experiences, or any additional tips you have on improving singletons in Objective-C. Engage with our community by leaving a comment below. Let's learn from each other's experiences and code like pros! 👨💻👩💻
Happy coding! 💻✨