What is the difference between class and instance methods?
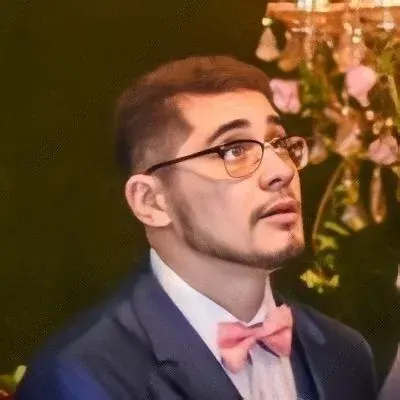
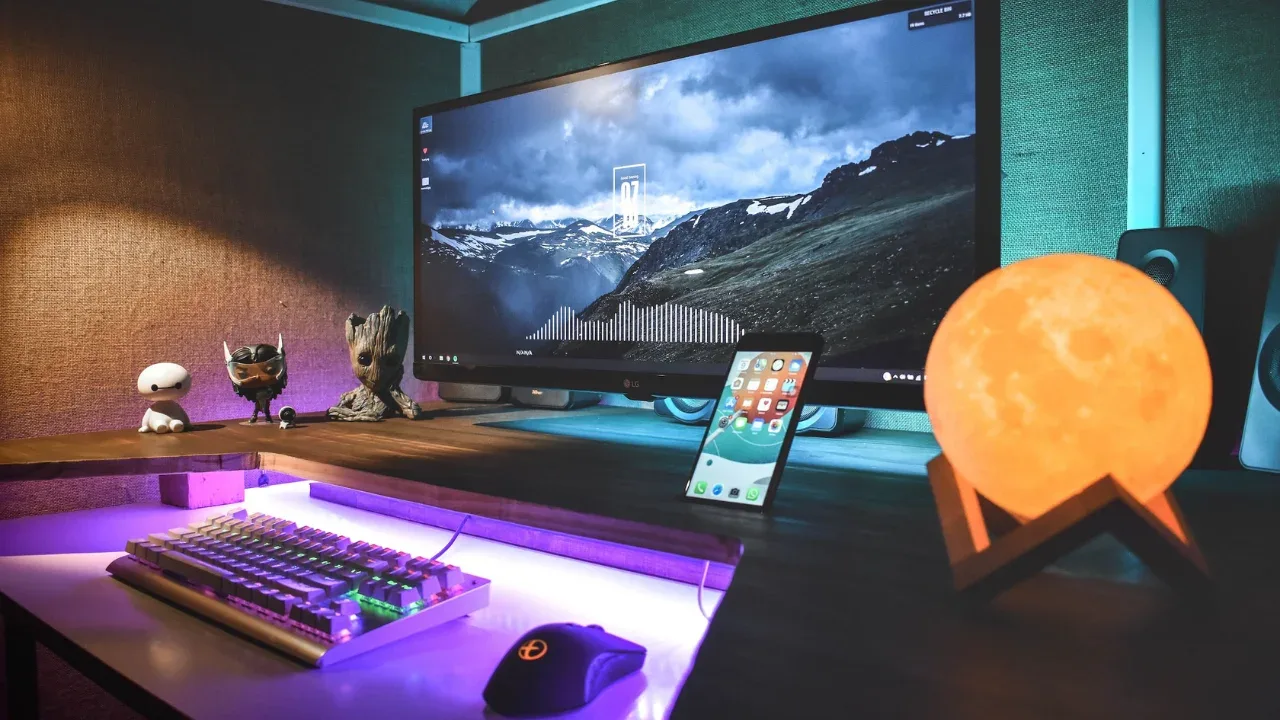
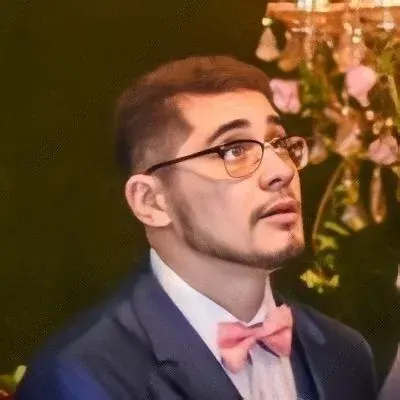
π Understanding the Difference Between Class and Instance Methods
Hitting us with some tech lingo, are we? πΆοΈ Today, we're diving into the difference between class methods and instance methods. πββοΈ So, buckle up and get ready for some code chit-chat! π»
What's the Deal with Class Methods and Instance Methods?
So, imagine you have a class called Dog
. πΆ We all love dogs, right? π Now, this class has some attributes like name
, age
, and breed
. Each Dog
object will have different values for these attributes.
An instance method is like a personalized doggy trick πΎ that can be performed by a specific instance (object) of the
Dog
class. It can operate on the individual attributes of that instance. For example, an instance methodbark()
will make a specific dog bark, like "Woof! Woof! πΎπΎ".On the other paw, a class method is like a universal doggy trick π© that can be performed by all the dogs in the
Dog
class (all instances). It can't operate on individual attributes because it doesn't know which dog it's operating on. π€·ββοΈ For example, a class methodrollOver()
will make all dogs roll over, regardless of their names, ages, or breeds. π
Confusion Unleashed: Are Instance Methods Just Getters and Setters?
Not exactly, my tech-savvy friend! While instance methods can indeed be used for getters and setters, they are not limited to just that. They can do so much more! π
Instance methods can perform any operation on the attributes of a specific object. For example, an instance method feed()
could take the name
and increase the age
of the dog by one. ππ
Additionally, they can also interact with other objects, perform calculations, and return values based on the logic defined within them. So, don't underestimate the power of instance methods! π₯
Let's Implement Some Code
To make things more paw-some, here's an example in Python π to help solidify our understanding:
class Dog:
def __init__(self, name, age, breed):
self.name = name
self.age = age
self.breed = breed
def bark(self):
print(f"{self.name} says: Woof! Woof!")
@classmethod
def rollOver(cls):
print("All dogs are rolling over!")
# Creating two dogs
dog1 = Dog("Max", 3, "Labrador")
dog2 = Dog("Bella", 5, "Poodle")
# Calling instance method
dog1.bark() # Output: Max says: Woof! Woof!
# Calling class method
Dog.rollOver() # Output: All dogs are rolling over!
Call-to-Action: Unleash Your Coding Paws!
Now that you've mastered the difference between class methods and instance methods, it's time to put your skills into action! πΎ Share this post with your fellow programmers, comment below with any questions or thoughts, and start implementing these concepts in your own projects. π
Happy coding! π