What is a typedef enum in Objective-C?
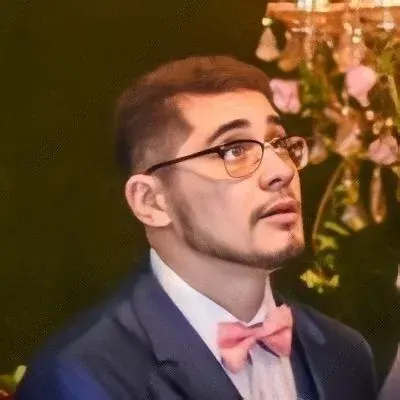
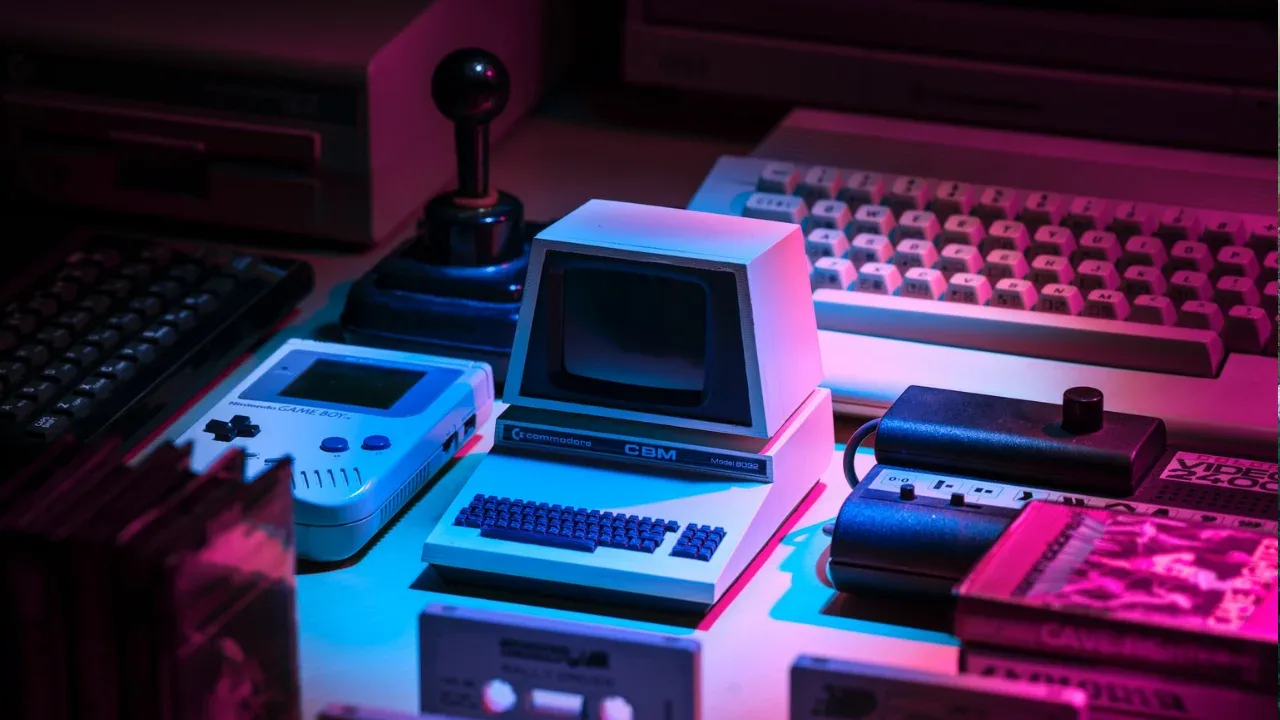
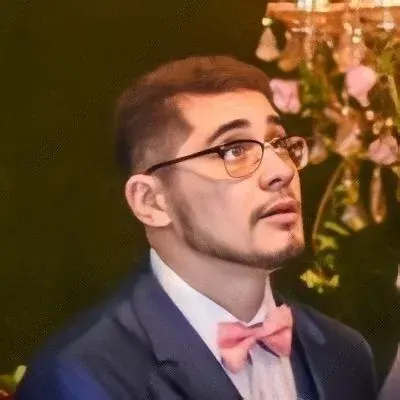
Understanding the Magic of typedef enum
in Objective-C 😎💫
Are you curious about the mysterious world of typedef enum
in Objective-C? 🤔 Having trouble understanding what it exactly does and when to use it? 🤯 Don't worry, we've got your back! In this blog post, we'll unravel the magic behind typedef enum
and provide you with easy-to-understand explanations and practical examples. Let's dive in! 🚀
Introducing typedef enum
🎉
At its core, typedef enum
in Objective-C allows you to create custom types with a set of named values. It combines two powerful features: enum
and typedef
. Let's break it down step by step. 👇
enum
- A Blueprint for Values 🏗️
First, let's talk about enum
. Think of it as a blueprint or design specification for a set of related values. In our example code snippet:
typedef enum {
kCircle,
kRectangle,
kOblateSpheroid
} ShapeType;
We are declaring a custom enum
with three possible values: kCircle
, kRectangle
, and kOblateSpheroid
. These values represent different shapes that our code can work with. 🎨
typedef
- Giving a Name to the Blueprint 🏷️
Now, let's add a pinch of typedef
to the mix! It allows us to give a name to our enum
blueprint, making it easier to use throughout our codebase. In our example, we've named our enum
ShapeType
:
typedef enum {
kCircle,
kRectangle,
kOblateSpheroid
} ShapeType;
The typedef
statement tells the compiler to treat ShapeType
as a custom type that is synonymous with the enum
containing our shape values. Now, instead of using the cumbersome declaration enum {...} ShapeType
, we can simply use ShapeType
to refer to our custom type. 🎉
The Big Picture 🖼️
To put it all together, when we write:
typedef enum {
kCircle,
kRectangle,
kOblateSpheroid
} ShapeType;
We are declaring a custom type named ShapeType
that represents a set of possible shapes (i.e., kCircle
, kRectangle
, kOblateSpheroid
). 🌈
Why Should I Care? 🤷♀️
The typedef enum
construct may seem like just another confusing syntax trick, but it brings several benefits to the table. Understanding why and when to use it can level up your Objective-C game! Let's explore why you should care: 💪
1. Improved Code Readability 👓
By using typedef enum
, you create meaningful names for sets of related values. It improves code readability by making your intentions clear. When you encounter ShapeType
in the code, you instantly know that it represents a shape, rather than just a random number.
2. Increased Type Safety 🔒
With typedef enum
, you enforce type safety. Instead of using plain integers or #define for your shape values, you use your custom type. This prevents accidental misuse and enables the compiler to catch more potential bugs.
3. Easy to Extend 🧩
If you need to add more shape values in the future, it's as simple as extending your enum
declaration. Because you have a named type, you only need to update your enum
, and the changes will automatically propagate throughout your codebase.
Easy Solutions for Common Issues 👍
Let's address some commonly faced issues when working with typedef enum
and provide you with easy-to-implement solutions! 🚀
Q: What if I want to assign custom values to each member of the enum
?
Sometimes, you may want to assign specific values to each member of your enum
. For instance, you may want to assign kCircle
a value of 1, kRectangle
a value of 2, and so on. To achieve this, you can manually assign values to the members:
typedef enum {
kCircle = 1,
kRectangle = 2,
kOblateSpheroid = 3
} ShapeType;
Now, kCircle
will be represented as 1, kRectangle
as 2, and kOblateSpheroid
as 3. Feel free to customize these values as needed. 🎨
Q: How can I assign custom names to my enum
values?
If you prefer more descriptive names instead of kCircle
and kRectangle
, you can assign custom names to your enum
values. For example:
typedef enum {
ShapeTypeCircle,
ShapeTypeRectangle,
ShapeTypeOblateSpheroid
} ShapeType;
Now, you can refer to the shape types using ShapeTypeCircle
, ShapeTypeRectangle
, and ShapeTypeOblateSpheroid
. Choose names that make sense in the context of your code! 🌈
Time to Embrace typedef enum
! 👏
Now that you have a solid understanding of typedef enum
in Objective-C, it's time to unleash its power in your code! 🔥 By creating custom types for related values, you can improve code clarity, increase type safety, and make your codebase easily extensible.
So, the next time you find yourself working with a set of related values in Objective-C, consider reaching for the typedef enum
magic! 🎩✨
We hope this blog post has helped you unravel the mysteries of typedef enum
and provided you with practical solutions to common issues. Feel free to share your thoughts, questions, or code snippets in the comments below! Let's level up our Objective-C skills together! 🚀💪
💌🎁 Time for Reader Engagement!
Did you find this blog post enlightening? 🌟 Tell us in the comments below and share it with your fellow Objective-C developers! Let's spread the knowledge and make coding a more enjoyable experience for everyone! 🎉
Connect with us on Twitter at @TechGuru for more tech tips, tricks, and exciting discussions. And don't forget to subscribe to our newsletter 📧 to stay up to date with the latest developments in the tech world!
Until next time, happy coding! 😄👩💻👨💻