Understanding dispatch_async
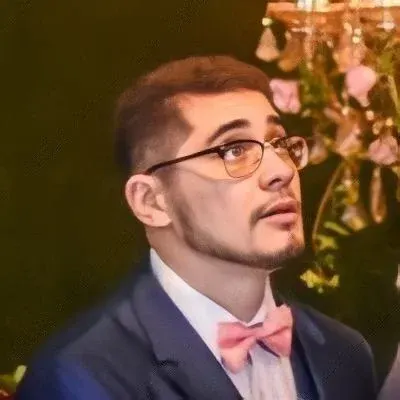
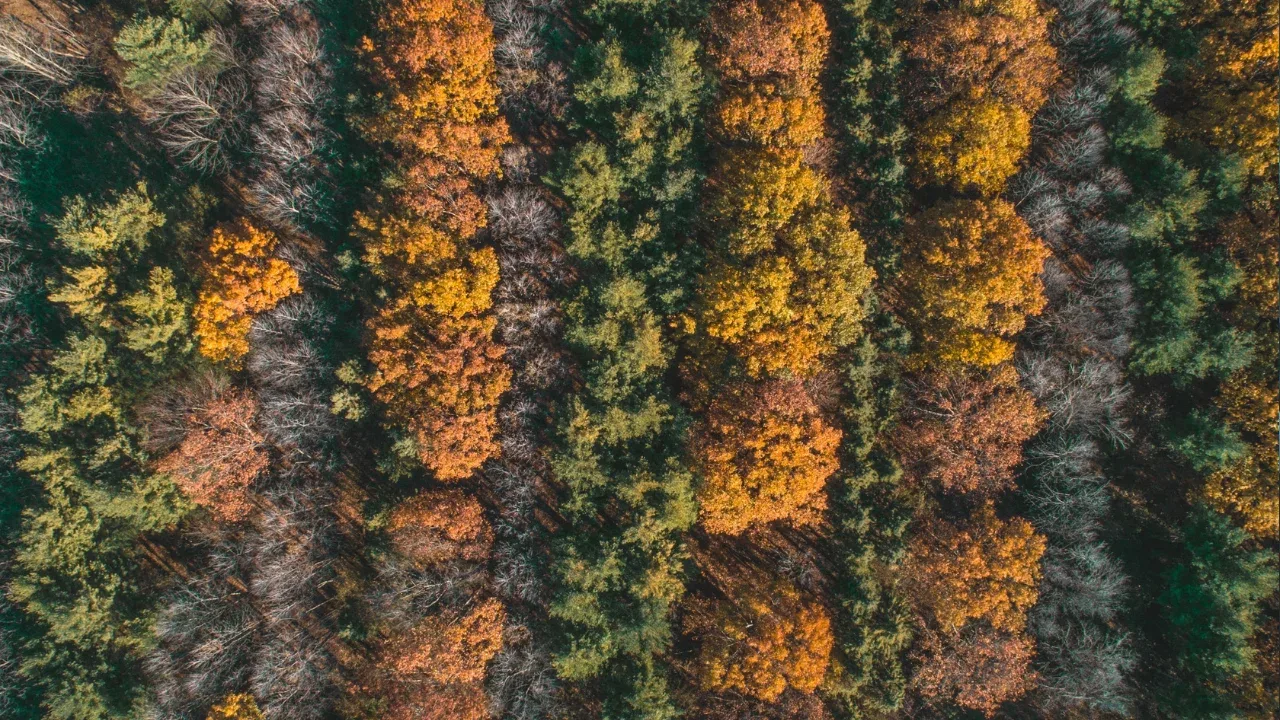
Understanding dispatch_async
🔄
In the world of multithreading, one of the most powerful tools at our disposal is dispatch_async
. It allows us to perform tasks off the main thread asynchronously, ensuring smoother user experience and optimal performance. 🚀
🔎 Let's break down the code snippet you provided and understand its purpose:
dispatch_async(dispatch_get_global_queue(DISPATCH_QUEUE_PRIORITY_DEFAULT, 0), ^{
NSData* data = [NSData dataWithContentsOfURL: kLatestKivaLoansURL];
[self performSelectorOnMainThread:@selector(fetchedData:) withObject:data waitUntilDone:YES];
});
💡 The first parameter of this code is dispatch_get_global_queue(DISPATCH_QUEUE_PRIORITY_DEFAULT, 0)
. By using this, we are asking the code to perform tasks on a global concurrent queue of a given priority level.
🌟 Advantage of using dispatch_get_global_queue
over the main queue:
1️⃣ Concurrency: The main queue executes tasks sequentially, one at a time, and on the main thread. However, using dispatch_get_global_queue
allows tasks to run concurrently on different threads, speeding up time-consuming operations such as network requests or data processing.
2️⃣ Preventing UI blocking: By offloading heavy tasks to a background thread, we prevent the main thread (responsible for UI rendering and user interaction) from being blocked. This ensures a smooth and responsive user interface.
3️⃣ Improved performance: Using multiple threads can greatly improve overall system performance, as it takes advantage of available resources and optimizes resource usage.
🤔 Now, let's address the confusion you mentioned. In the provided code, an asynchronous block is dispatched to a global queue. Inside this block, it performs a synchronous network request using dataWithContentsOfURL:
. Finally, it calls fetchedData:
on the main thread using performSelectorOnMainThread
. The purpose of this code seems to be fetching data asynchronously and updating the UI when done.
🔑 However, there is an issue with the code provided. Blocking the global queue by performing a synchronous network request defeats the purpose of using dispatch_async
. It's recommended to use asynchronous networking APIs or wrap the synchronous network request in an asynchronous block to avoid blocking the global queue.
🛠 Here's an updated version of the code, addressing this issue using NSURLSession
:
dispatch_async(dispatch_get_global_queue(DISPATCH_QUEUE_PRIORITY_DEFAULT, 0), ^{
NSURLSession *session = [NSURLSession sharedSession];
NSURL *url = [NSURL URLWithString:kLatestKivaLoansURL];
NSURLSessionDataTask *dataTask = [session dataTaskWithURL:url completionHandler:^(NSData *data, NSURLResponse *response, NSError *error) {
if (data) {
dispatch_async(dispatch_get_main_queue(), ^{
[self fetchedData:data];
});
} else {
NSLog(@"%@", error);
// Handle error gracefully
}
}];
[dataTask resume];
});
✅ In this updated code, we use NSURLSession
and its asynchronous dataTaskWithURL
method. Once the data is received, we dispatch the UI update to the main queue using dispatch_async(dispatch_get_main_queue())
, ensuring it's performed on the main thread.
🤩 Now that you have a better understanding of dispatch_async
and its benefits, go forth and conquer the world of multithreading! If you have any more questions or want to share your experiences, leave a comment below. We'd love to hear from you! 🎉
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
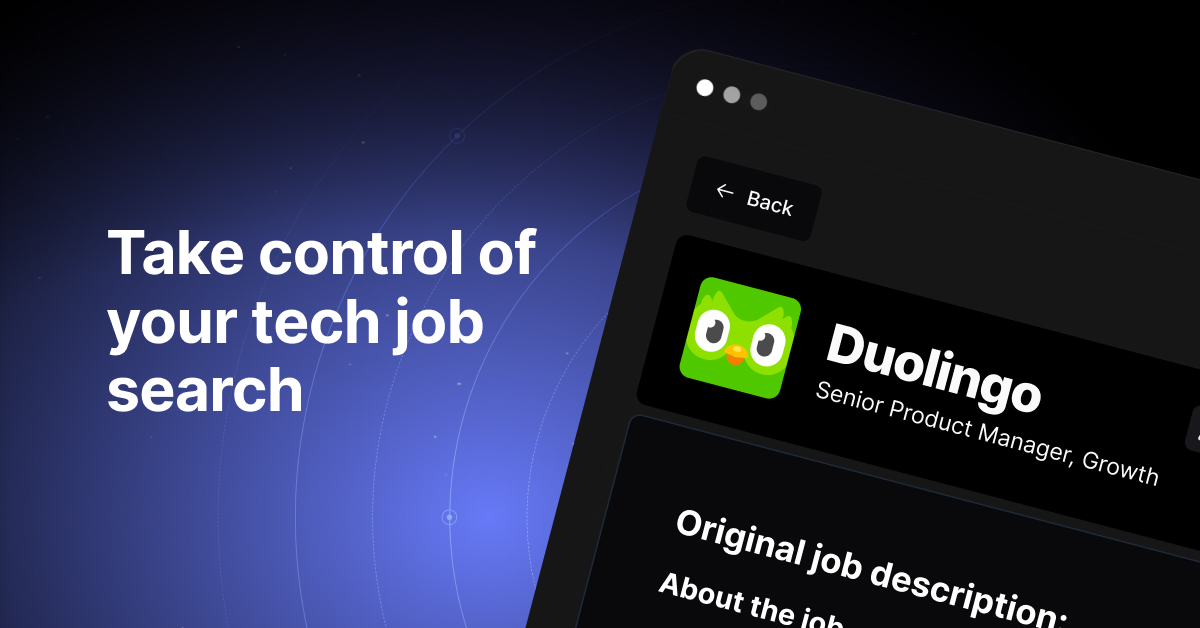