UIRefreshControl without UITableViewController
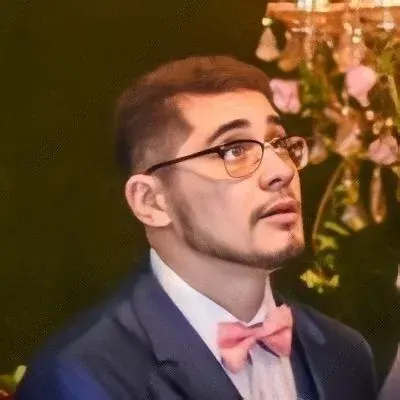
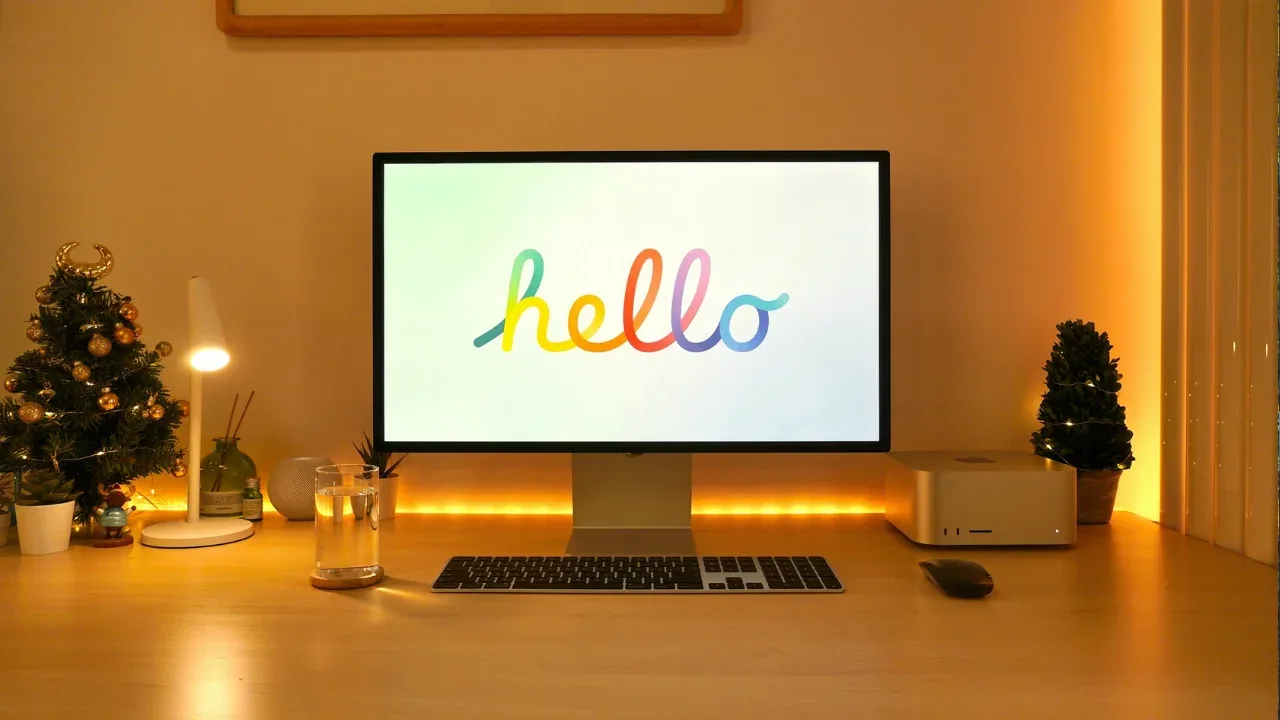
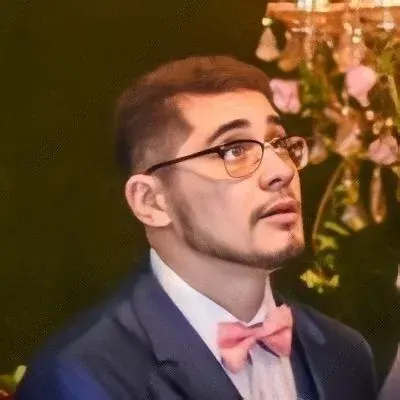
🔄 Using UIRefreshControl without UITableViewController - A Sneaky Trick! 💡📲
So, you love the cool new iOS 6 UIRefreshControl
class for implementing pull-to-refresh functionality in your app. But, there's just one problem - you prefer using a UIViewController
with a UITableView
subview, rather than a UITableViewController
subclass. Fear not, my friend, for there is a sneaky way to achieve your desired magic! 😎
The Dilemma: 🤔🚫
The question at hand is whether it's possible to utilize the powerful UIRefreshControl
without the need for a UITableViewController
subclass. Luckily, the answer is YES. You can definitely leverage UIRefreshControl
in your UIViewController
with a UITableView
subview! 🎉
The Solution: ✔️🤓
To make this happen, you need to follow a few simple steps:
Create a Reference to Your
UITableView
: First, create an outlet for yourUITableView
in yourUIViewController
class. You can do this by adding the following code snippet to yourUIViewController
subclass:
@IBOutlet weak var tableView: UITableView!
Instantiate
UIRefreshControl
: Next, you'll need to instantiate an instance ofUIRefreshControl
in yourUIViewController
class. This is done as follows:
let refreshControl = UIRefreshControl()
Add
UIRefreshControl
toUITableView
: Now, you'll need to add theUIRefreshControl
instance to yourUITableView
subview. Place the following code snippet inside yourviewDidLoad()
method:
if #available(iOS 10.0, *) {
tableView.refreshControl = refreshControl
} else {
tableView.addSubview(refreshControl)
}
Implement Refresh Action: 👆➡️💫 Lastly, implement the action that will be triggered when the user pulls down to refresh. You can add the following code snippet to your
UIViewController
subclass:
refreshControl.addTarget(self, action: #selector(refresh), for: .valueChanged)
Make sure to also implement the refresh()
function anywhere within your UIViewController
class. This function will contain the logic to refresh your data and update your table view.
Example: 🌟💻
Here's a quick example to reinforce the steps discussed above:
In your
UIViewController
subclass, add the following code snippet to create an outlet for yourUITableView
:
@IBOutlet weak var tableView: UITableView!
Next, create an instance of
UIRefreshControl
:
let refreshControl = UIRefreshControl()
Add the
UIRefreshControl
instance to yourUITableView
subview:
if #available(iOS 10.0, *) {
tableView.refreshControl = refreshControl
} else {
tableView.addSubview(refreshControl)
}
Implement the
refresh()
function and the associated action:
@objc private func refresh() {
// Add your data refreshing and table view updating logic here
}
Conclusion and Call-to-Action: 🏁📣
Congratulations, you've successfully unleashed the power of UIRefreshControl
in your UIViewController
with a UITableView
subview! Now, go ahead and implement this sneaky trick in your app to create a delightful pull-to-refresh experience for your users. Happy refreshing! 😄
If you found this guide helpful or have any other interesting tricks up your sleeve, let me know in the comments below. Don't forget to share this post with your fellow iOS developers, and together, we can make the world of iOS coding a little more refreshing! 🙌✨