@synthesize vs @dynamic, what are the differences?
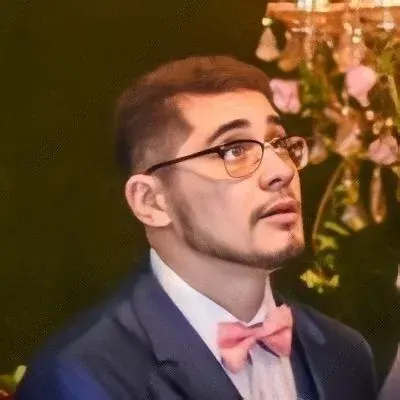
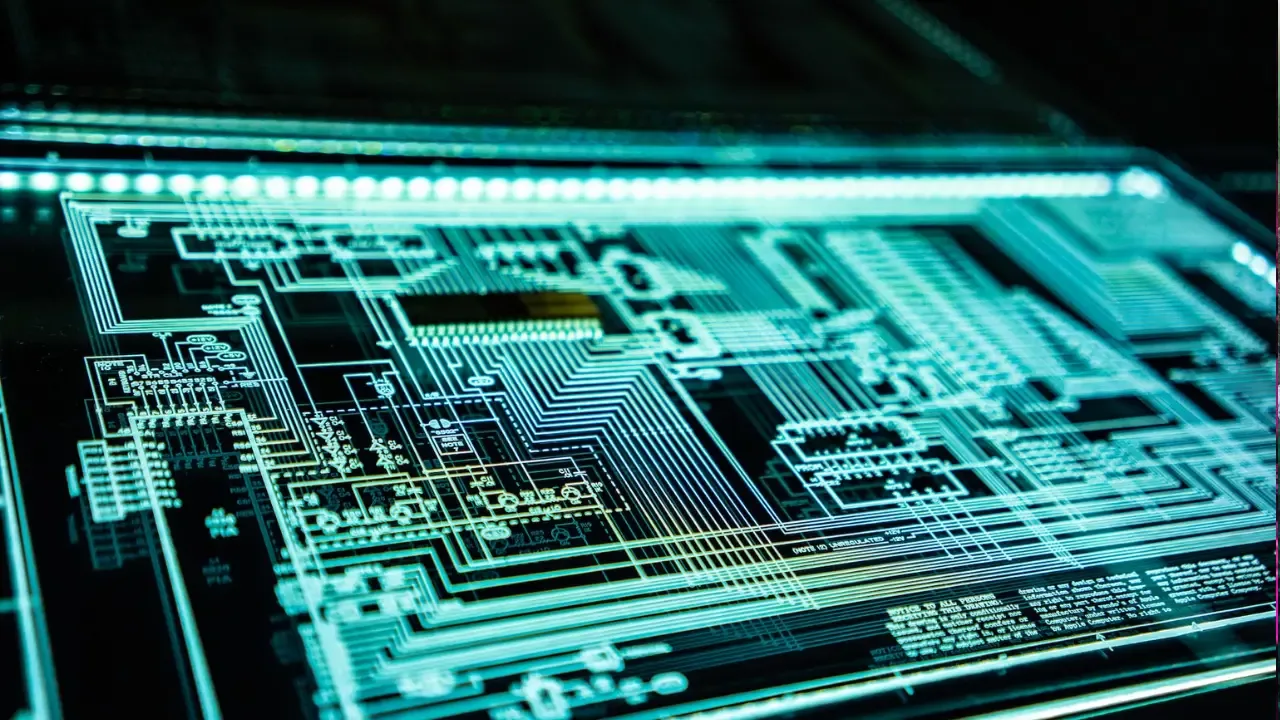
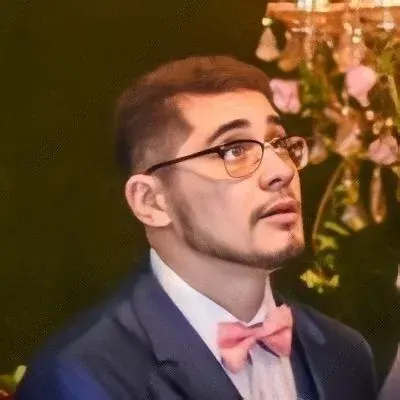
๐ Understanding @synthesize vs @dynamic: What are the differences? ๐ญ
If you've dabbled in Objective-C or Swift programming, you may have come across the keywords "@synthesize" and "@dynamic" when implementing properties. ๐ค These keywords play a crucial role in how properties are synthesized and accessed, but what exactly are the differences between them? Let's dive into the details and shed some light on this common question. ๐ต๏ธโโ๏ธ
๐ฅ Synthesize, meet Dynamic:
When it comes to Objective-C, declaring properties involves using the @property
keyword. ๐ก Prior to version 2.0, developers had to explicitly create the accessors (getter
and setter
) methods for these properties. That's where @synthesize
comes into play. It automatically generates the accessor methods based on the property definition. ๐
On the other hand, "@dynamic" tells the compiler that the accessors will be implemented at runtime or dynamically by the developer. In other words, you're taking the responsibility of manually creating the getter and setter methods yourself. ๐ ๏ธ
๐ The Difference in Implementation:
Now that we understand the basic concept, let's see how these keywords differ in their implementation. ๐ง
@synthesize ๐ค
When using @synthesize
, the compiler automatically generates the accessors for you. Let's take a look at an example:
@interface Car : NSObject
@property(nonatomic, strong) NSString *make;
@end
@implementation Car
@synthesize make;
@end
In the example above, the @synthesize
keyword generates the getter
and setter
methods for the property 'make'. No need to write those repetitive methods manually! ๐
@dynamic ๐งฉ
Now, let's see how @dynamic
differs from @synthesize
. When using @dynamic
, the developer explicitly states that they will handle the implementation of the accessors. Take a look at the following example:
@interface Car : NSObject
@property(nonatomic, strong) NSString *model;
@end
@implementation Car
@dynamic model;
- (NSString *)model {
// Custom implementation for the getter method
}
- (void)setModel:(NSString *)newModel {
// Custom implementation for the setter method
}
@end
In this example, by using @dynamic
, we're telling the compiler that the accessors for the 'model' property will be implemented manually. You have the flexibility to customize the behavior of these methods as per your requirements. ๐งฐ
๐ง When to Use Each Keyword:
So, when should you use @synthesize
or @dynamic
in your code? Let's break it down:
Use
@synthesize
when you want the compiler to automatically generate the getter and setter methods for you. This is often the preferred option when the default behavior suits your needs. โUse
@dynamic
when you want to take control over the implementation of the getter and setter methods. This can be useful when you need to handle custom logic or interact with other parts of your codebase. ๐ช
๐ The Verdict:
In conclusion, the differences between @synthesize
and @dynamic
boil down to who generates the accessors. @synthesize
automates the process, while @dynamic
requires you to manually implement the accessors. It all depends on your specific needs and the level of control you desire. ๐ค
Don't hesitate to experiment with both options to see which one best suits your project requirements ๐งช. Happy coding! ๐ป
Have any insights or experiences with @synthesize
or @dynamic
to share? Drop a comment below and let's dive into a lively discussion! ๐ฃ๏ธ