Objective-C: Extract filename from path string
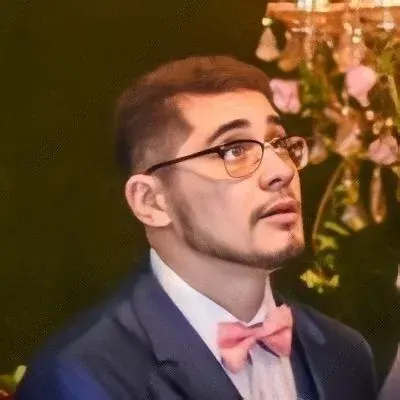
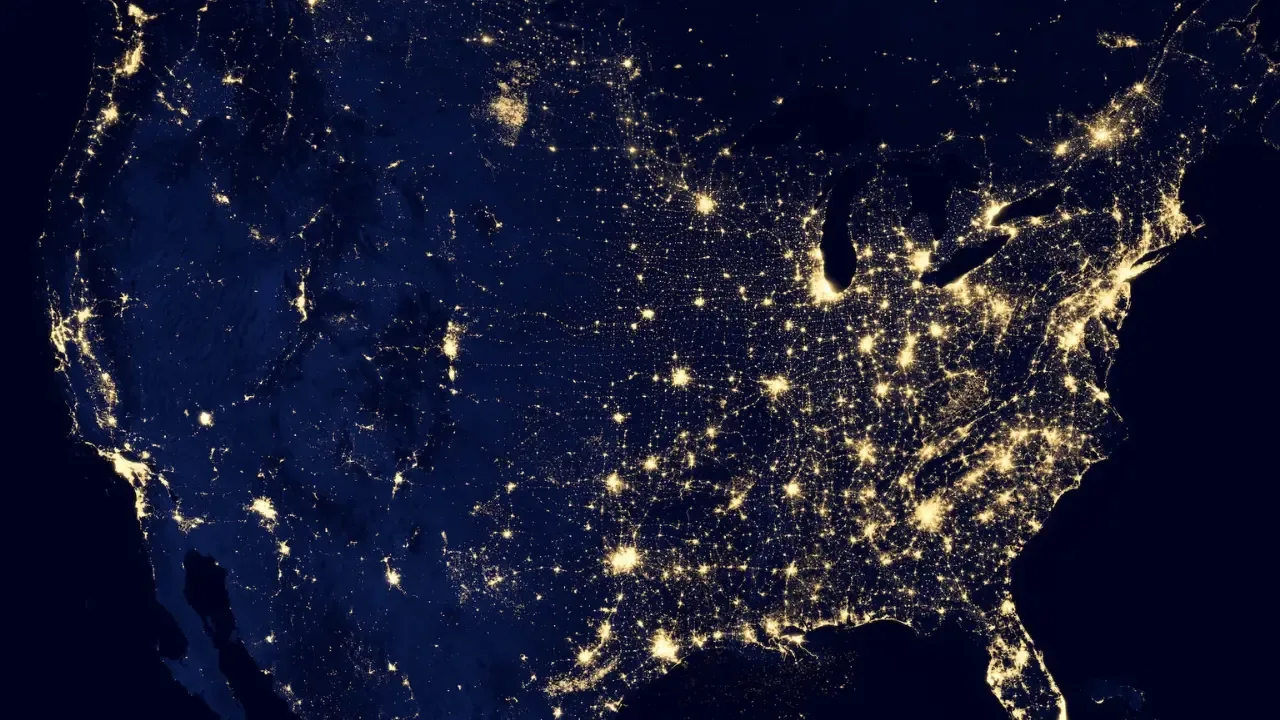
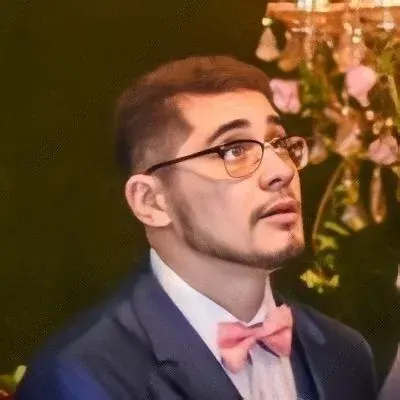
📝💡 Extracting a Filename from a Path String in Objective-C
Are you tired of struggling to extract just the filename from a path string in Objective-C? 🤔 Don't worry, we've got you covered! In this blog post, we'll walk you through an easy solution to this commonly faced problem. So, let's dive right in! 💪
The Problem
So, you have an NSString that contains a path like /Users/user/Projects/thefile.ext
, and you want to extract just the filename thefile
from it. Sounds simple, right? But figuring out the easiest way to do it can be a bit tricky. 😕
The Solution
Fortunately, Objective-C provides us with some handy methods that make extracting a filename from a path string a piece of 🍰. Let's take a look at a simple and efficient solution:
NSString *filePath = @"/Users/user/Projects/thefile.ext";
NSString *fileName = [filePath lastPathComponent];
NSString *fileNameWithoutExtension = [fileName stringByDeletingPathExtension];
NSLog(@"The extracted filename is: %@", fileNameWithoutExtension);
In the above code snippet, we're utilizing the lastPathComponent
method of NSString
to extract the filename along with the extension. However, since we only want the filename without the extension, we further use the stringByDeletingPathExtension
method to achieve our desired result. 🎉
Example
Let's put our solution to the test with a real-life example:
NSString *filePath = @"/Users/user/Projects/thefile.ext";
NSString *fileName = [filePath lastPathComponent];
NSString *fileNameWithoutExtension = [fileName stringByDeletingPathExtension];
NSLog(@"The extracted filename is: %@", fileNameWithoutExtension);
Running the code snippet above will output:
The extracted filename is: thefile
As you can see, we successfully extracted the filename thefile
from the given path string. 🎊
Conclusion
Extracting a filename from a path string in Objective-C doesn't have to be a daunting task. By utilizing the lastPathComponent
and stringByDeletingPathExtension
methods of NSString
, you can easily extract just the filename without breaking a sweat. 😎
Give it a try in your own projects and let us know if you found this solution helpful! Happy coding! 💻✨
PS: If you have any other tricky Objective-C problems or questions, feel free to leave them in the comments below. We love hearing from our readers! ❤️