NSString property: copy or retain?
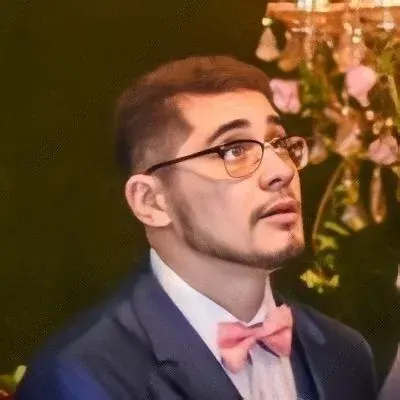
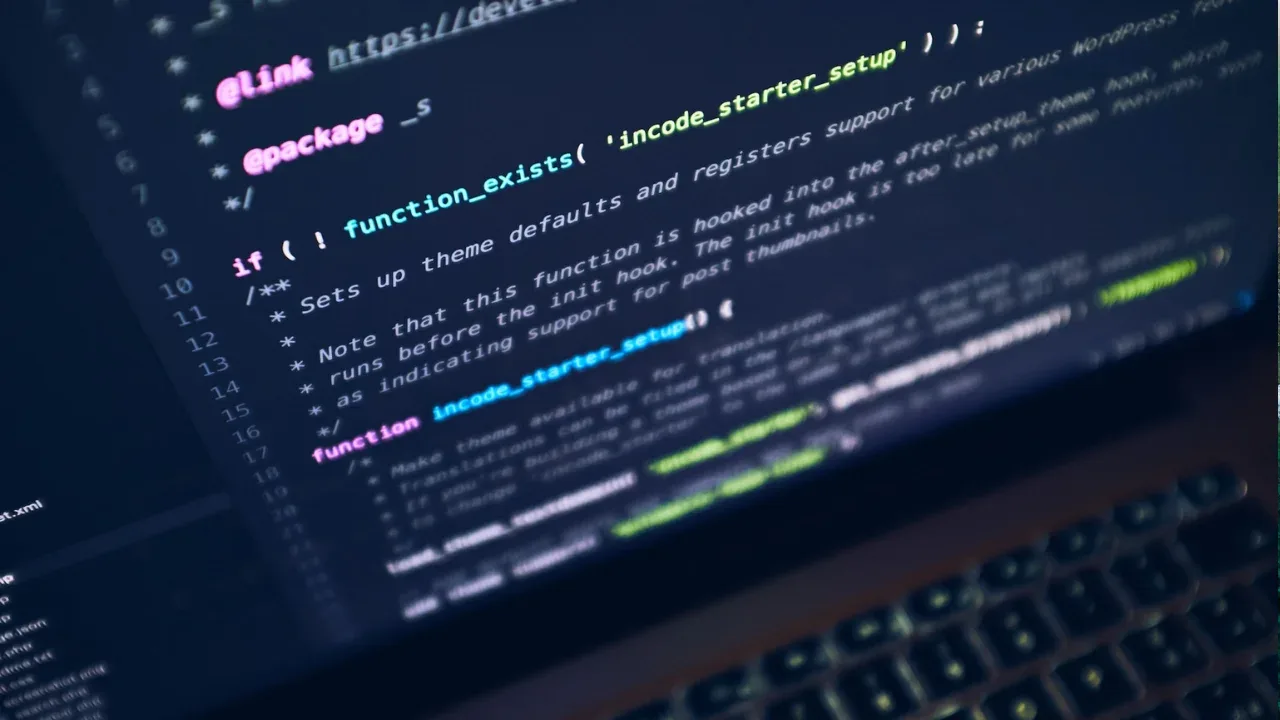
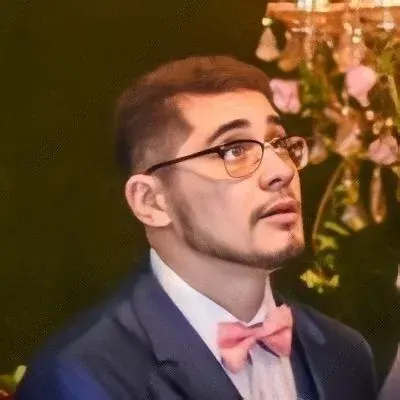
NSString property: copy or retain?
š When it comes to managing an NSString
property in Objective-C, the question of whether to use the copy
attribute or the retain
attribute often arises. It's crucial to understand the differences and potential issues to ensure your code behaves as expected. Let's dive into it! š
The Problematic Scenario
šØāš» Imagine you have a class called SomeClass
with a string property named name
. Here's a snippet to give you an idea:
@interface SomeClass : NSObject
{
NSString* name;
}
@property (nonatomic, retain) NSString* name;
@end
š¤ In this scenario, you might be wondering if using the retain
attribute for the name
property is always the best choice. This concern is particularly valid when dealing with mutable strings (NSMutableString
), as it can lead to erratic behavior. Let's explore some alternatives. š
Always Use "copy" for Strings?
š Generally, using the copy
attribute instead of retain
for string properties is considered a good practice. By doing so, you ensure that each time the property is set, a new immutable copy of the string is created. This approach protects against potential issues caused by modifying the original string unexpectedly. š”ļø
Efficiency Matters
š Now, you might be wondering whether a "copied" property is less efficient than a "retain-ed" property. It's a valid concern, as creating a copy sounds more time-consuming. However, in most cases, the performance impact is negligible. šļø
ā”ļø Plus, remember that the copy
attribute only creates a shallow copy of the string. It's not a deep copy, which means it doesn't duplicate the entire string. Instead, it creates a new reference to the existing string, which is usually a fast operation. So in terms of efficiency, the difference is generally insignificant. š
A Call to Action
š¤ Hopefully, this guide has shed some light on the question of whether to use copy
or retain
for NSString
properties. By opting to use copy
, you ensure a more consistent and reliable behavior, especially when dealing with mutable strings.
š Do you have any other questions or tech dilemmas you'd like us to cover? Let us know in the comments below! We're here to help and provide you with more informative content. Together, we can master the tech universe! ššŖ
š Happy coding! š