In Objective-C, how do I test the object type?
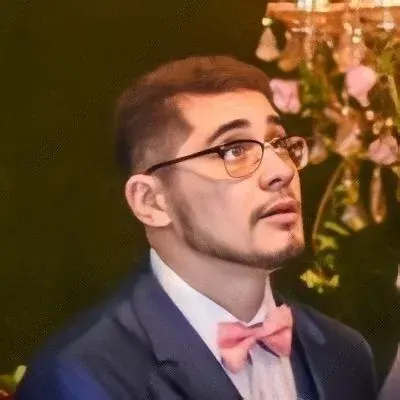
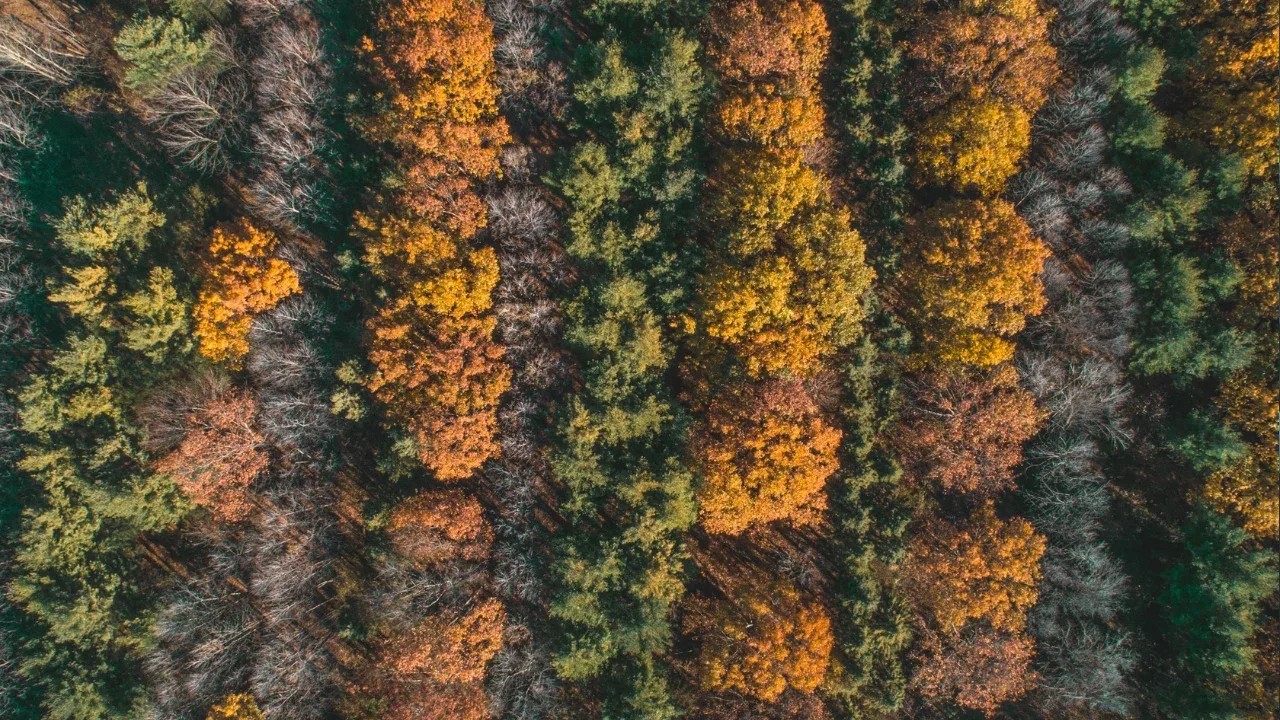
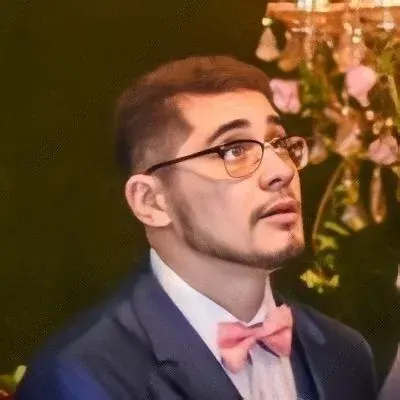
š Title: "Objective-C Type Testing Made Easy: How to Determine Object Types in a Snap! šµļøāāļø"
š Introduction: Hey tech enthusiasts! Are you struggling with determining the type of an object in Objective-C? š¤ Fear not! In this guide, we'll dive into the world of type testing and uncover the easiest solutions to help you ace this common challenge. Whether you're dealing with an NSString or UIImageView, we've got you covered! Let's get started! š
š” Understanding the Problem: So, you want to test whether an object is of type NSString or UIImageView. However, you're not sure if there's a specific "isoftype" method to achieve this. Let's clarify the confusion and point you in the right direction! šŖ
š§ Easy Solutions:
šÆ Using
isKindOf:
Method: TheisKindOf:
method helps you determine if an object is of a certain class or subclass. Here's an example of how to use it:BOOL isNSString = [myObject isMemberOfClass:[NSString class]]; BOOL isUIImageView = [myObject isMemberOfClass:[UIImageView class]];
In this code snippet,
myObject
is the object you want to test. TheisMemberOfClass:
method compares the object's class to the specified class, returning aBOOL
value indicating whether it matches. Easy peasy! ššÆ Using
isKindOfClass:
Method: Another useful method in Objective-C isisKindOfClass:
, which checks if an object is of a specific class or any of its subclasses. Here's an example of how to use it:BOOL isNSStringOrSubclass = [myObject isKindOfClass:[NSString class]]; BOOL isUIImageViewOrSubclass = [myObject isKindOfClass:[UIImageView class]];
By using
isKindOfClass:
, you can expand the scope of your type testing to include subclasses of the desired class. How convenient! āØšÆ Using
respondsToSelector:
Method: Sometimes, you might want to check if an object responds to a certain selector, regardless of its class. Here's an example:BOOL respondsToStringMethod = [myObject respondsToSelector:@selector(length)]; BOOL respondsToImageMethod = [myObject respondsToSelector:@selector(image)];
In this case,
length
andimage
are method names specific to NSString and UIImageView, respectively. By utilizingrespondsToSelector:
, you can determine if an object supports a particular method. Isn't that neat? š©
š Reader Engagement: Now that we've unravelled the mysteries of Objective-C type testing, it's time for you to put your knowledge into practice! Try out the code snippets provided and see the magic happen before your eyes.
Leave a comment below to let us know which method worked best for you or share any other tips and tricks you have for testing object types in Objective-C. We'd love to hear from you! š£ļø
š Conclusion: Congratulations on conquering the Objective-C type testing challenge! We hope this guide helped you gain a better understanding of how to determine object types with confidence.
Remember, with the isKindOf:
, isKindOfClass:
, and respondsToSelector:
methods in your toolkit, you'll be able to handle any type-testing scenario like a pro. š
Stay curious and keep exploring the fascinating world of Objective-C! Until next time, happy coding! š»āØ