How to print out the method name and line number and conditionally disable NSLog?
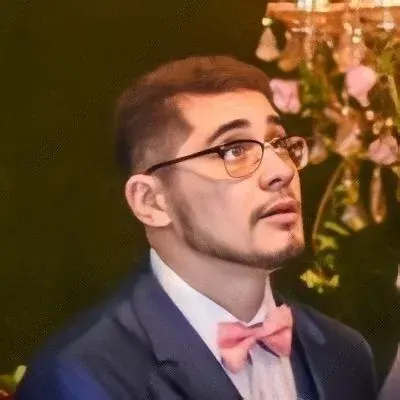
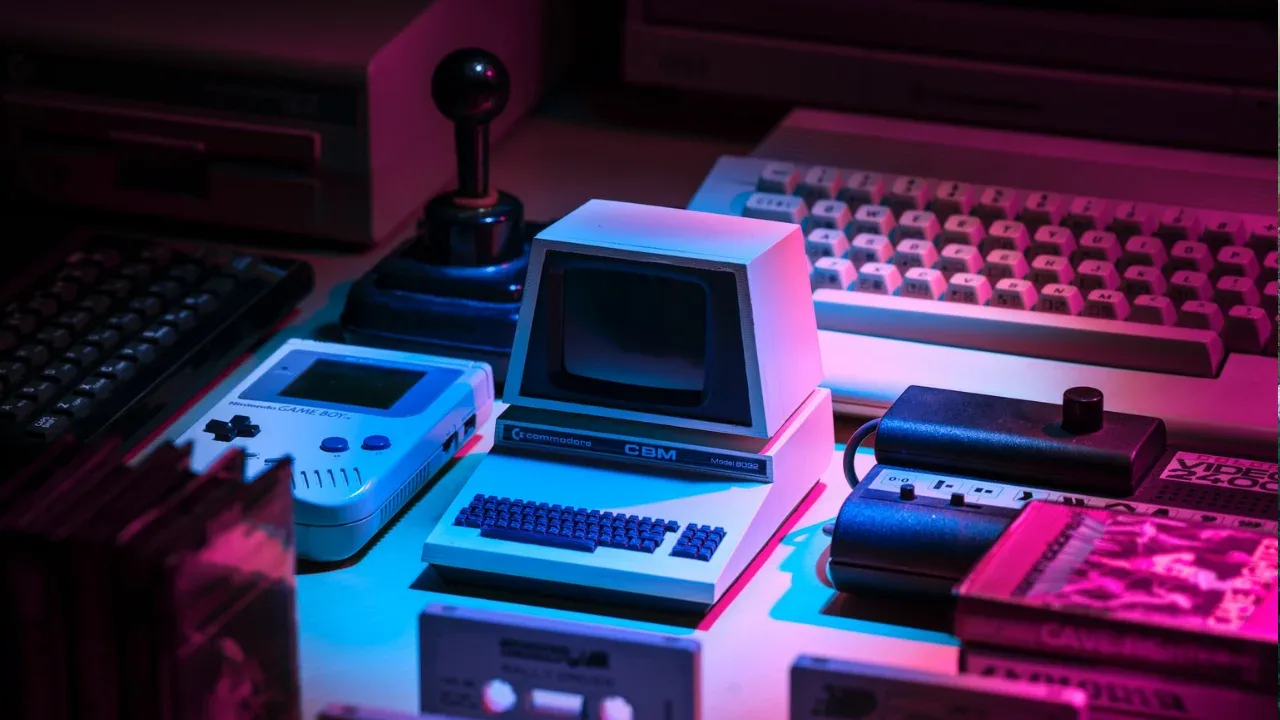
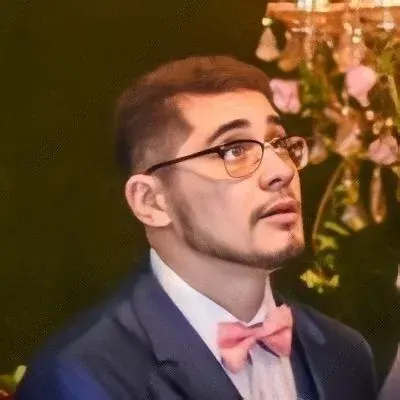
How to Print Method Name and Line Number in Xcode + How to Conditionally Disable NSLog
Are you tired of spending hours trying to find bugs in your Xcode projects? Do you want to be able to easily track the method names and line numbers where issues occur? And what about disabling NSLog statements when you want to compile your code for release?
In this blog post, we will address these common issues and provide you with easy solutions that will save you time and frustration in your debugging process. So, let's dive in and level up your Xcode debugging skills!
Logging the Current Method's Name and Line Number
Logging the current method's name and line number can be extremely helpful when trying to track down issues in your code. It allows you to quickly identify where a problem is occurring and can provide valuable context when analyzing logs.
To achieve this in Xcode, we can use the __PRETTY_FUNCTION__
and __LINE__
macros. These macros are pre-defined in Objective-C and automatically expand into the current method's name and line number, respectively.
NSLog(@"Current Method: %s:%d", __PRETTY_FUNCTION__, __LINE__);
By placing this line of code in your project's source code, you will see the method name and line number printed out in the console whenever this line is executed. This information can greatly aid in troubleshooting and pinpointing the source of a bug.
Disabling NSLog Statements for Release Code
During development, NSLog statements are often our best friends. They provide us with valuable information about what's happening behind the scenes. However, when it comes time to ship our app, these NSLog statements can impact performance and unnecessarily clutter the console output.
To disable NSLog statements for release code, one common approach is to define a macro, let's call it ENABLE_LOGS
, that we can easily enable or disable based on our needs. Here's an example:
#ifdef DEBUG
#define ENABLE_LOGS 1
#else
#define ENABLE_LOGS 0
#endif
// Usage example
#if ENABLE_LOGS
NSLog(@"This message will only appear in DEBUG builds!");
#endif
In this example, we define the ENABLE_LOGS
macro to 1 when we are in the DEBUG configuration (development) and 0 when we are in any other configuration, such as RELEASE.
By wrapping your NSLog statements with #if ENABLE_LOGS
and #endif
, the NSLog statements will only be executed when ENABLE_LOGS
is set to 1. When compiling your code for release, you can easily disable all NSLog statements by changing the value of ENABLE_LOGS
to 0.
Take Your Debugging Game to the Next Level!
Now that you know how to print out the current method's name and line number as well as conditionally disable NSLog statements, you are well-equipped to level up your Xcode debugging skills!
Start implementing these techniques in your projects and experience the benefits for yourself. Save time and frustration with easier bug tracking, improve your app's performance by disabling unnecessary logging, and ship your code with confidence.
If you found this blog post useful, don't forget to share it with your fellow Xcode developers! And if you have any other Xcode debugging tips or tricks, feel free to share them in the comments below.
Happy debugging! 😎💻🚀