How do you print out a stack trace to the console/log in Cocoa?
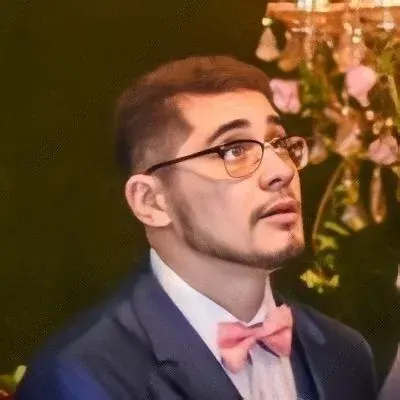
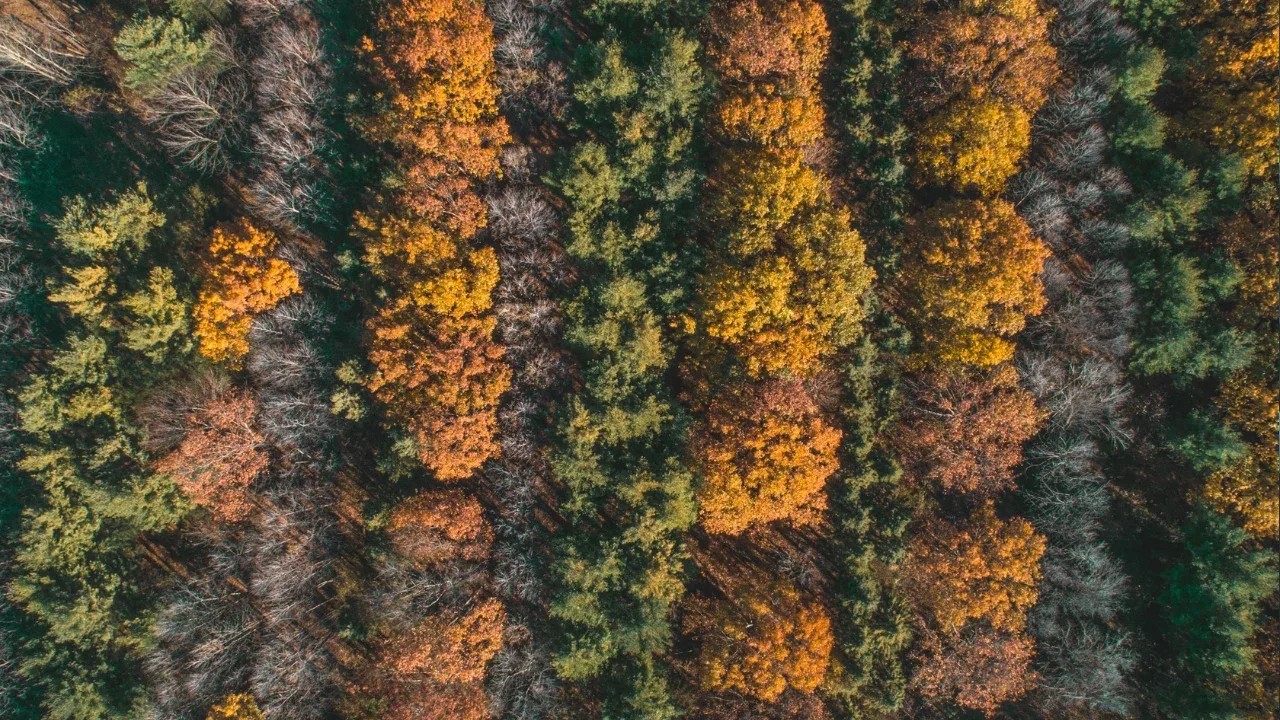
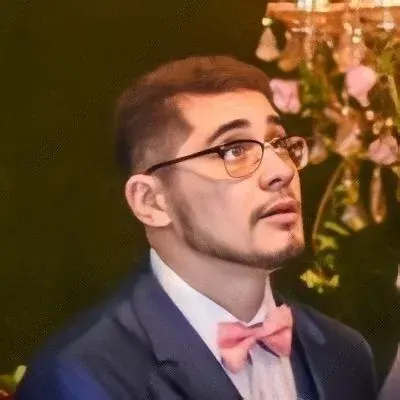
🖨️ Printing Stack Trace in Cocoa: A Guide for Developers
Are you a Cocoa developer who wants to level up their debugging skills? 🚀 In this post, we'll be exploring how to easily print out a stack trace to the console or log in your Cocoa applications. 🖥️
Understanding the Context
You're working on your Cocoa project, and you've encountered situations where you need to log the call trace at certain points, such as failed assertions or uncaught exceptions. This can be incredibly helpful for troubleshooting and finding the root cause of issues. 🐞
The Stack Trace
Before we dive into the solutions, let's quickly understand what a stack trace is. 🧐 A stack trace is a record of the active functions in a program's call stack at a specific point in time. Essentially, it shows you the path the program took to reach a certain point.
Solution 1: Using NSThread.callStackSymbols
One way to print out a stack trace in Cocoa is by using the NSThread.callStackSymbols
property. This property gives you an array of strings, each representing a function call in the call stack. You can simply log these strings to get your stack trace. 📝
Let's take a look at some example code:
func printStackTrace() {
let stackTrace = Thread.callStackSymbols
for symbol in stackTrace {
print(symbol)
}
}
By calling the printStackTrace()
function, you'll see the stack trace printed to the console. 🔍
Solution 2: Using Exception Handling
Another approach is to use exception handling in Cocoa. By catching an exception and printing its stack trace, you can get valuable information about what led to the exception being raised. Here's an example:
func handleException() {
do {
try somethingThatMightRaiseException()
} catch let error {
let exception = NSException(name: NSExceptionName(rawValue: "Exception"), reason: nil, userInfo: nil)
exception.raise()
}
}
By raising a custom NSException
and not providing any reason or additional information, the exception will contain the stack trace. 📚
Call-to-Action: Level Up Your Debugging Skills
Are you excited to take your debugging skills to the next level? 😍 Mastering the art of printing stack traces can save you hours of frustration when debugging tricky issues. Start implementing the solutions mentioned in this post and see how they can make your debugging process smoother.
Let us know in the comments below how you plan to use stack traces in your Cocoa projects. Share your experiences and tips with the community! 👇🗣️
Happy coding! 💻✨