How do I iterate over an NSArray?
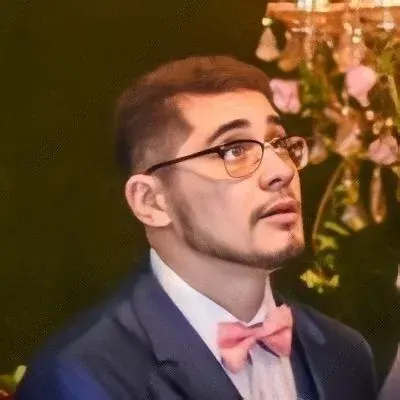
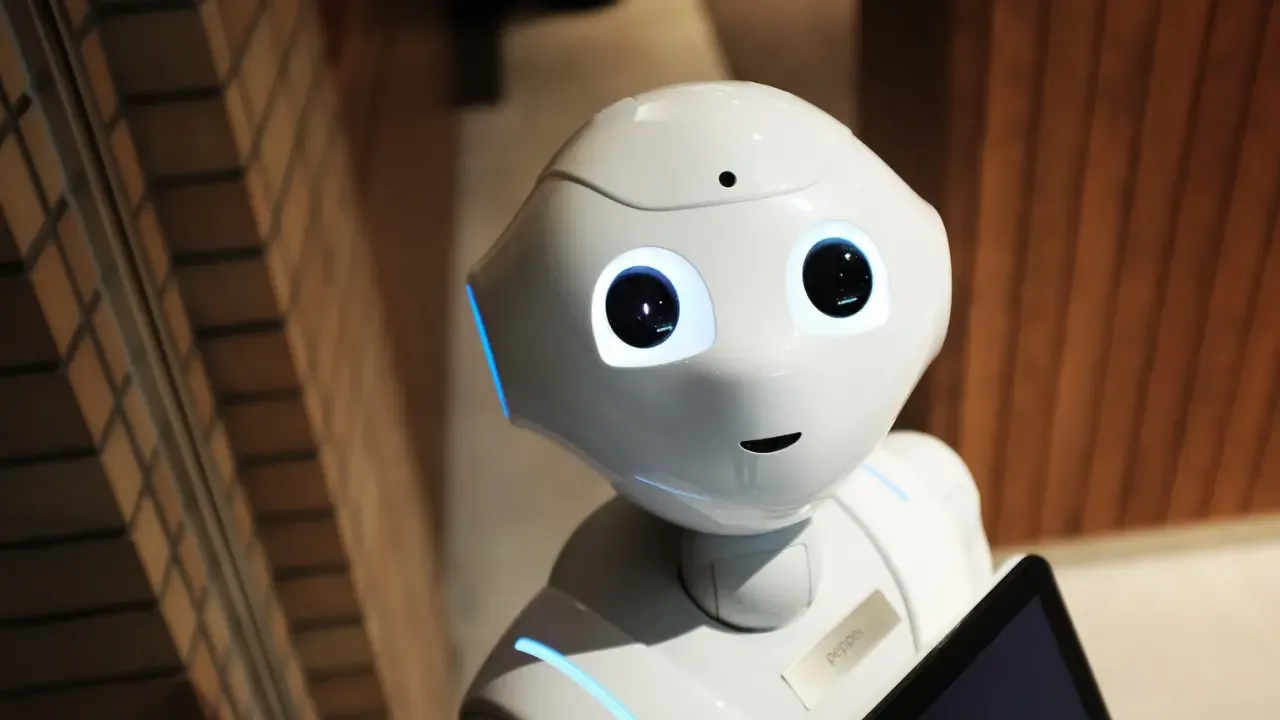
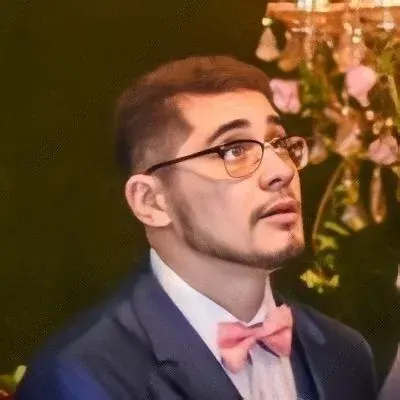
ššš Arrays Are Fun: A Beginner's Guide to Iterating Over an NSArray ššš
š¤ Are you scratching your head, wondering how to iterate over an NSArray? Don't worry! We've got you covered. Whether you're a beginner or an experienced developer, this guide will help you conquer the challenges associated with NSArray iteration. š»š
šÆ Let's dive right in and address the common issues and specific problems developers face when dealing with NSArrays.
š The Problem: A developer is looking for the standard idiom to iterate over an NSArray that's suitable for OS X 10.4+.
š” The Solution: There are several ways to iterate over an NSArray, but let's focus on the two most common and efficient methods: fast enumeration and using a traditional loop.
1ļøā£ Fast Enumeration:
This method is sleek, modern, and compatible with OS X 10.5+ (which we assume is not a concern for our developer).
for (id object in myArray) {
// Do something with 'object'
}
š object
represents each element in the array, ensuring seamless iteration. It's like a magic wand that gives you access to each item one by one, without causing any runtime errors. šŖāØ
š„ With just three lines of code, the fast enumeration approach saves time and keeps your code succinct.
2ļøā£ Traditional Loop:
This method may be preferred if you're working with older versions of macOS (OS X 10.4 or earlier).
NSUInteger arrayCount = [myArray count];
for (NSUInteger i = 0; i < arrayCount; i++) {
id object = [myArray objectAtIndex:i];
// Do something with 'object'
}
š In this approach, i
serves as an index to access elements within the array, and object
grabs each item using objectAtIndex:
method. It's like a treasure hunt, where you know the exact location of each object. šš
š„ Though it requires a few extra lines of code, the traditional loop provides backward compatibility, ensuring your code runs smoothly on older systems.
š Now that you know the ABCs of NSArray iteration, it's time for you to rock the coding world! šŖ
š Engagement Call-to-Action: Did this guide help you tame the wild NSArray? Tell us in the comments below! Share this blog post with fellow developers who might find it useful. Let's spread the knowledge and make coding simpler for everyone! š¬š¤š
⨠Stay tuned for more exciting tech tips and tricks. Happy coding! āØ