How do I convert NSMutableArray to NSArray?
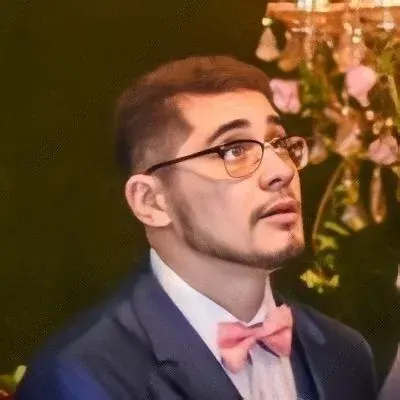
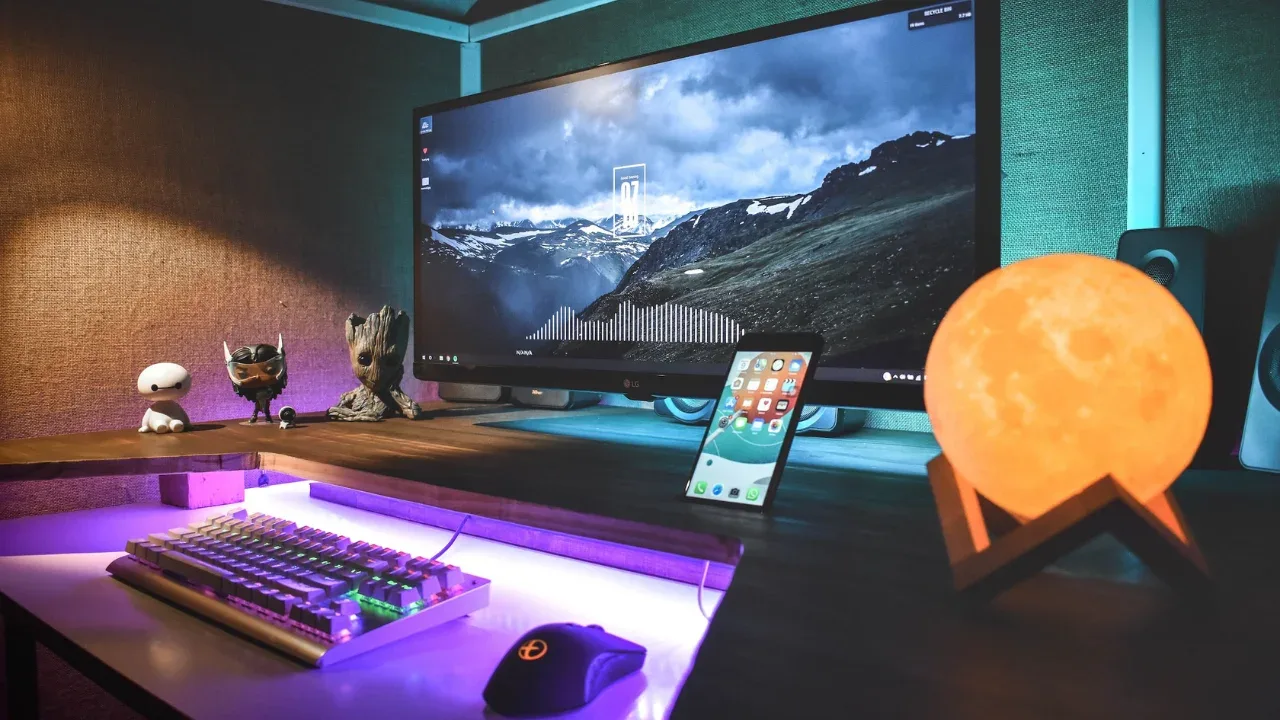
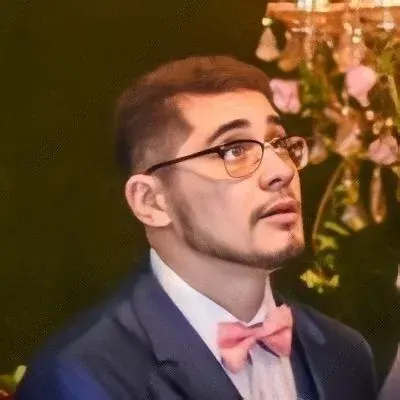
How to Convert NSMutableArray to NSArray in Objective-C? 🔄
So, you have an NSMutableArray
and you want to convert it into an immutable NSArray
in Objective-C? No worries, we've got you covered! 🙌
The Problem 🤔
NSMutableArray
and NSArray
are both classes that represent ordered collections in Objective-C. The main difference is that NSMutableArray
is mutable, meaning you can add, remove, or modify elements after its creation, while NSArray
is immutable, and its content cannot be changed once it's been initialized.
But sometimes we need to convert an NSMutableArray
into an NSArray
for various reasons, such as passing it to a method that requires an immutable array, or to make sure the data remains unchanged.
The Solution 💡
The good news is, converting an NSMutableArray
to an NSArray
is pretty straightforward. There are a couple of methods we can use for this conversion, depending on your specific requirements:
Method 1: Using the initWithArray:
Method
NSMutableArray *mutableArray = [NSMutableArray arrayWithObjects:@"Apple", @"Banana", @"Cherry", nil];
NSArray *immutableArray = [[NSArray alloc] initWithArray:mutableArray];
Here, we create an NSMutableArray
called mutableArray
with some sample elements. Then, we create an NSArray
called immutableArray
by using the initWithArray:
method and passing the mutableArray
as an argument. This method creates a new immutable array with the same contents as the mutable array.
Method 2: Using the arrayWithArray:
Class Method
NSMutableArray *mutableArray = [NSMutableArray arrayWithObjects:@"Apple", @"Banana", @"Cherry", nil];
NSArray *immutableArray = [NSArray arrayWithArray:mutableArray];
Similar to the first method, we start by creating an NSMutableArray
called mutableArray
. Then, we create an NSArray
called immutableArray
using the arrayWithArray:
class method and passing the mutableArray
. This method also creates a new immutable array with the same elements as the mutable array.
The Code in Action 💻
Let's see the conversion in action by printing the content of the mutable and immutable arrays:
NSMutableArray *mutableArray = [NSMutableArray arrayWithObjects:@"Apple", @"Banana", @"Cherry", nil];
NSArray *immutableArray = [NSArray arrayWithArray:mutableArray];
NSLog(@"Mutable Array: %@", mutableArray);
NSLog(@"Immutable Array: %@", immutableArray);
When you run the code, you will see the following output:
Mutable Array: (
Apple,
Banana,
Cherry
)
Immutable Array: (
Apple,
Banana,
Cherry
)
As you can see, the content of both arrays is the same, but the mutableArray
can be modified, whereas the immutableArray
remains unchanged.
The Call-to-Action 📣
Now that you know how to convert an NSMutableArray
to an NSArray
in Objective-C, why not try it out in your own code? Experiment and see how it fits into your project's requirements. If you have any questions or run into any issues, feel free to leave a comment below or reach out to the awesome Objective-C community for support. Happy coding! 😊
Have you ever needed to convert an NSMutableArray
to an NSArray
in Objective-C? Share your experience with us! 👇