How do I add 1 day to an NSDate?
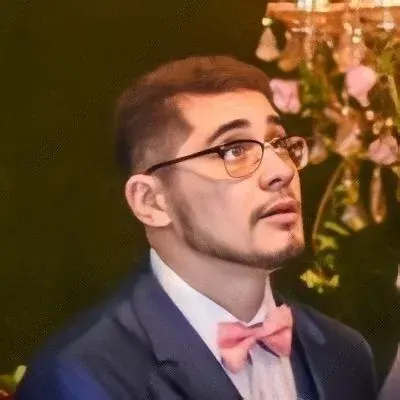
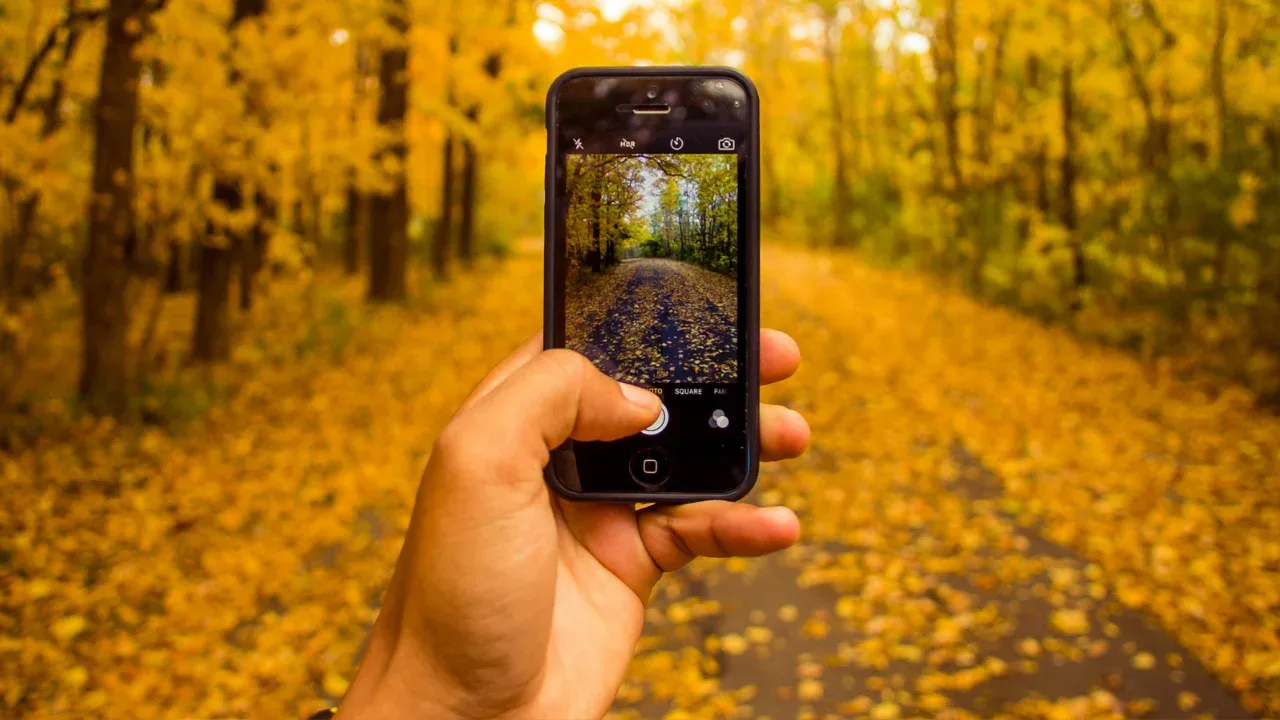
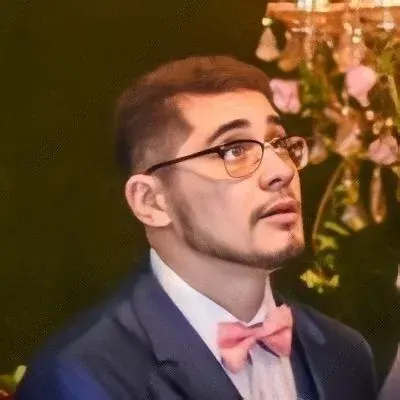
How to Add 1 Day to an NSDate
Are you facing the challenge of adding one day to an NSDate
? Don't worry, we've got you covered! In this guide, we'll explore different solutions to effortlessly add one day to a given date in Objective-C.
The Problem
So, you have an NSDate
object representing a specific date, and you need to increment it by one day. For example, you want to go from 21st February 2011 to 22nd February 2011 or from 31st December 2011 to 1st January 2012.
Easy Solutions
Solution 1: Using NSCalendar and NSDateComponents
The first solution involves using NSCalendar
and NSDateComponents
to add one day to the original date. Here's how you can achieve this:
// Assuming you have an existing `NSDate` object called `originalDate`
// Step 1: Create the calendar instance
NSCalendar *calendar = [NSCalendar currentCalendar];
// Step 2: Define the components to add (1 day)
NSDateComponents *dayComponent = [[NSDateComponents alloc] init];
dayComponent.day = 1;
// Step 3: Get the new date by adding the defined components
NSDate *newDate = [calendar dateByAddingComponents:dayComponent toDate:originalDate options:0];
Solution 2: Using NSDate's dateByAddingTimeInterval:
method
The second solution is a bit simpler and involves using the dateByAddingTimeInterval:
method provided by NSDate
. This method allows you to add a specific time interval to a given date. In our case, the time interval would be one day, which is 24 * 60 * 60 seconds (86400 seconds). Here's the code:
// Assuming you have an existing `NSDate` object called `originalDate`
// Step 1: Calculate the time interval for one day (24 hours * 60 minutes * 60 seconds)
NSTimeInterval oneDay = 24 * 60 * 60;
// Step 2: Add the time interval to the original date
NSDate *newDate = [originalDate dateByAddingTimeInterval:oneDay];
Wrap Up and Call to Action
Congratulations! 🎉 You have successfully learned two different ways to add one day to an NSDate
. You can now confidently manipulate dates in your Objective-C projects.
Now, it's your turn to put this knowledge into action. Try out the code snippets provided and let us know how it goes. If you have any questions, suggestions, or other date-related challenges, feel free to comment below. We love engaging with our readers! 🤝