How can I reverse a NSArray in Objective-C?
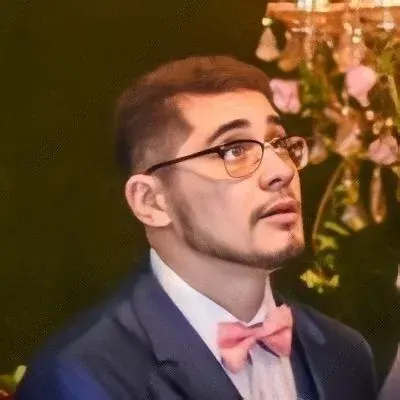
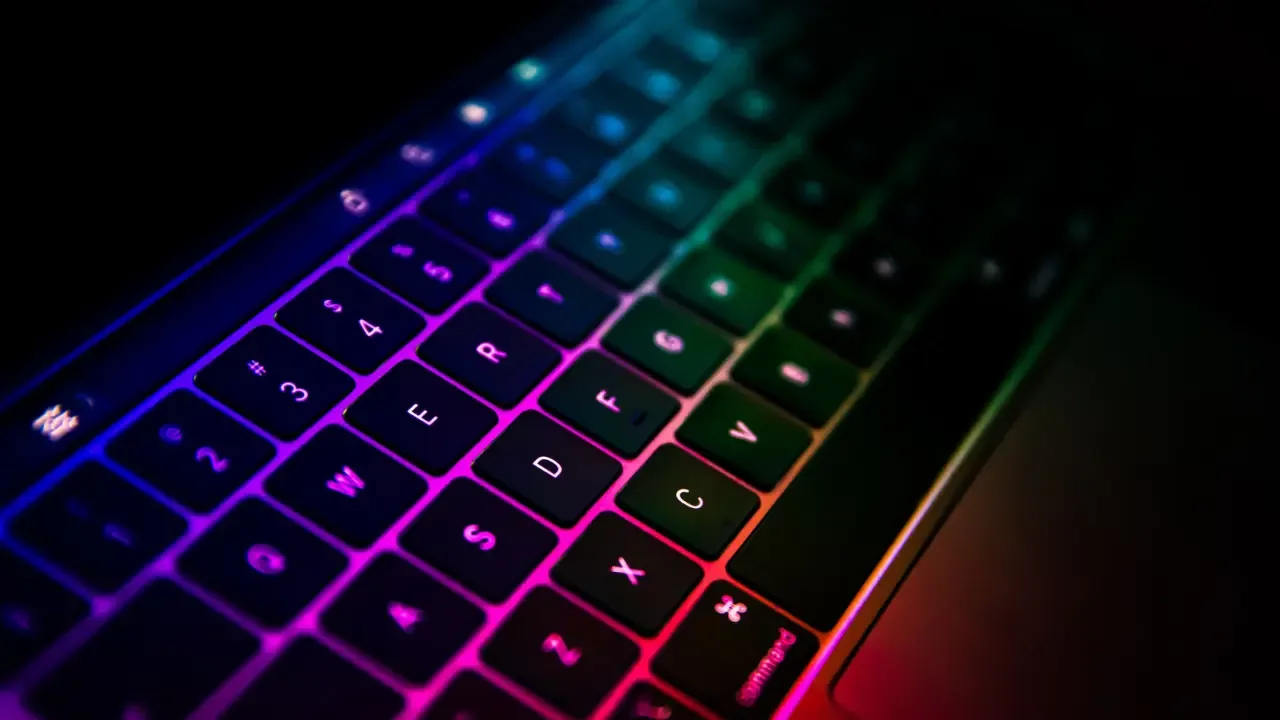
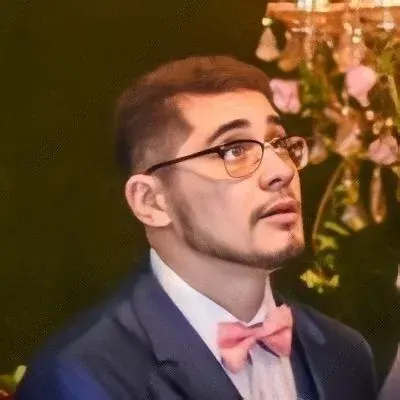
Reversing an NSArray in Objective-C: A Simple Guide 🔄
So, you have an NSArray
that you want to reverse in Objective-C. Whether you're a beginner or an experienced developer, this common problem can be easily solved. In this blog post, we will explore the best way to reverse an NSArray
and provide you with a simple and efficient solution. Let's get started!
The Problem: Reversing an NSArray
Let's take a look at the problem statement. You have an NSArray
:
NSArray *array = @[ @1, @2, @3, @4, @5 ];
And your goal is to reverse it. The desired output should be:
@[ @5, @4, @3, @2, @1 ]
Finding the Best Solution 🕵️♀️
When it comes to reversing an NSArray
in Objective-C, you might find several different approaches suggested by developers. However, we will focus on the most efficient and straightforward solution.
Solution: The Best Way to Reverse an NSArray
To reverse an NSArray
, one of the simplest approaches is to make use of the NSMutableArray
class. Here's a step-by-step guide to reverse your NSArray
:
Create an instance of
NSMutableArray
using your originalNSArray
:
NSMutableArray *mutableArray = [NSMutableArray arrayWithArray:array];
Utilize the
reverseObjectEnumerator
method to reverse the order of the elements in theNSMutableArray
:
[mutableArray reverseObjectEnumerator];
Finally, convert the
NSMutableArray
back to anNSArray
using theNSArray
class methodarrayWithArray:
:
NSArray *reversedArray = [NSArray arrayWithArray:mutableArray];
And voila! 🎉 Your NSArray
is now reversed.
Testing and Verification ✅
To ensure that our solution works as expected, let's test it with the original array [1,2,3,4,5]
:
NSArray *array = @[ @1, @2, @3, @4, @5 ];
NSMutableArray *mutableArray = [NSMutableArray arrayWithArray:array];
[mutableArray reverseObjectEnumerator];
NSArray *reversedArray = [NSArray arrayWithArray:mutableArray];
NSLog(@"%@", reversedArray);
If everything goes well, the output should be:
@[ @5, @4, @3, @2, @1 ]
Conclusion and Call-to-Action ⭐️
Reversing an NSArray
in Objective-C can be easily achieved by using the reverseObjectEnumerator
method of NSMutableArray
. With just a few lines of code, you can transform your original NSArray
into the desired reversed version.
Start implementing this solution today and streamline your array-reversing tasks in your Objective-C projects. If you found this blog post helpful, share it with your fellow developers and spread the knowledge!
If you have any other interesting topics or questions you'd like us to cover, feel free to leave a comment below. We'd love to hear from you! Happy coding! 👩💻
References:
📢 Stay connected! Follow us on Twitter for more exciting tech insights and tips!