Get the current first responder without using a private API
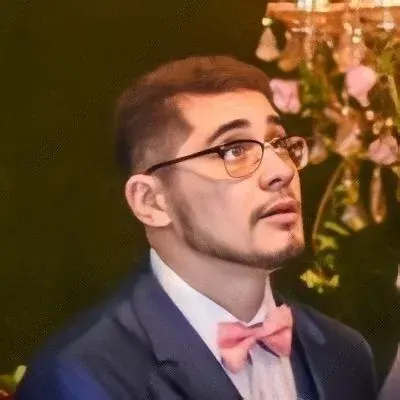
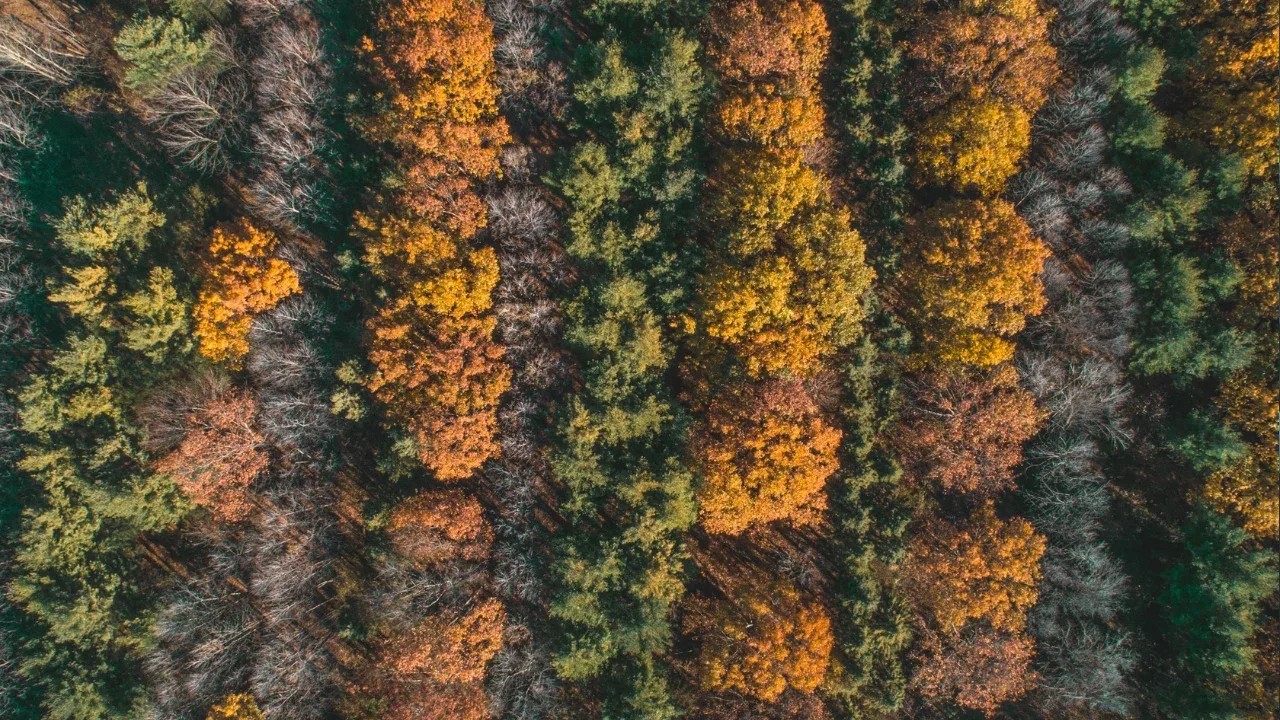
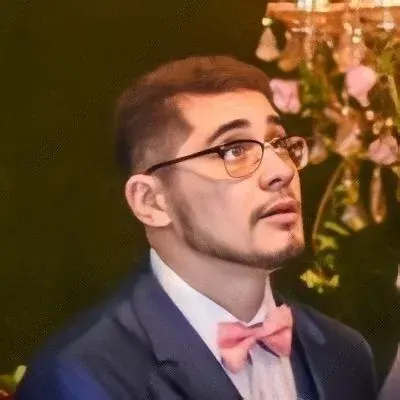
Get the Current First Responder Without Using a Private API
๐ Hey there, fellow app developer! So, you've encountered the dreaded rejection email stating that your app cannot be accepted because you're using a non-public API, more specifically, the firstResponder
.
โ Don't worry, we've got your back! In this blog post, we'll address your problem and provide you with easy solutions to get the current first responder on the screen while ensuring your app doesn't get rejected. ๐ฑ๐ฅ
Understanding the Issue
So, what exactly is this firstResponder
API that got your app rejected? โ๏ธ The firstResponder
is a private API, which means it's not publicly accessible and using it violates Apple's App Store guidelines.
In your code snippet, you're wisely trying to get the current first responder by using the keyWindow
and performSelector:
methods. However, this approach uses the private firstResponder
API, leading to the rejection of your app. ๐ข
Easy Solutions
Fortunately, there are alternative ways to achieve the desired functionality without using private APIs. Let's explore some of these easy solutions:
Solution 1: UIResponder Extension
One way to get the current first responder is by extending the UIResponder
class. By doing this, you can create a custom method that returns the first responder for a given window. ๐๏ธ
Here's an example of how you can implement this solution:
extension UIResponder {
static weak var currentFirstResponder: UIResponder?
func findFirstResponder() {
UIResponder.currentFirstResponder = self
}
}
With this extension in place, you can call findFirstResponder()
on any UIResponder
object (e.g., UIViewController
, UIView
) to set it as the current first responder.
Solution 2: Notification Observers
Another approach involves utilizing notification observers. iOS sends various notifications when the first responder changes, and we can leverage that to accomplish our goal.
Here's a code snippet to demonstrate how you can implement this solution:
NotificationCenter.default.addObserver(forName: UIResponder.keyboardDidShowNotification,
object: nil,
queue: .main) { notification in
guard let firstResponder = notification.userInfo?[UIResponder.keyboardFirstResponderUserInfoKey] as? UIResponder else {
return
}
// Use the firstResponder here
}
By observing the UIResponder.keyboardDidShowNotification
notification, you can capture the first responder whenever the keyboard appears.
Time to Take Action!
Now that you have some easy-to-implement solutions, it's time to put them into action! Remember, the key is to ensure you're not using any private APIs to avoid rejection from the App Store. ๐
Choose the solution that suits your app's requirements and start integrating it into your code. And don't forget to test thoroughly to ensure everything works as expected. ๐งช
Share Your Success Story!
We would love to hear about your experience implementing the solution and whether it solved your problem. Share your success story with us in the comments below! ๐ฌ๐
If you have any other questions or additional solutions you'd like to share, feel free to join the discussion. Let's help each other overcome challenges and make our apps even better!
Happy coding! ๐ปโจ