GCD to perform task in main thread
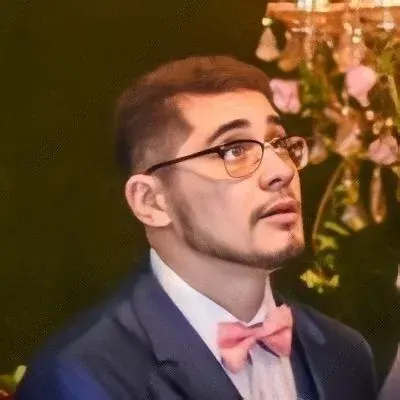
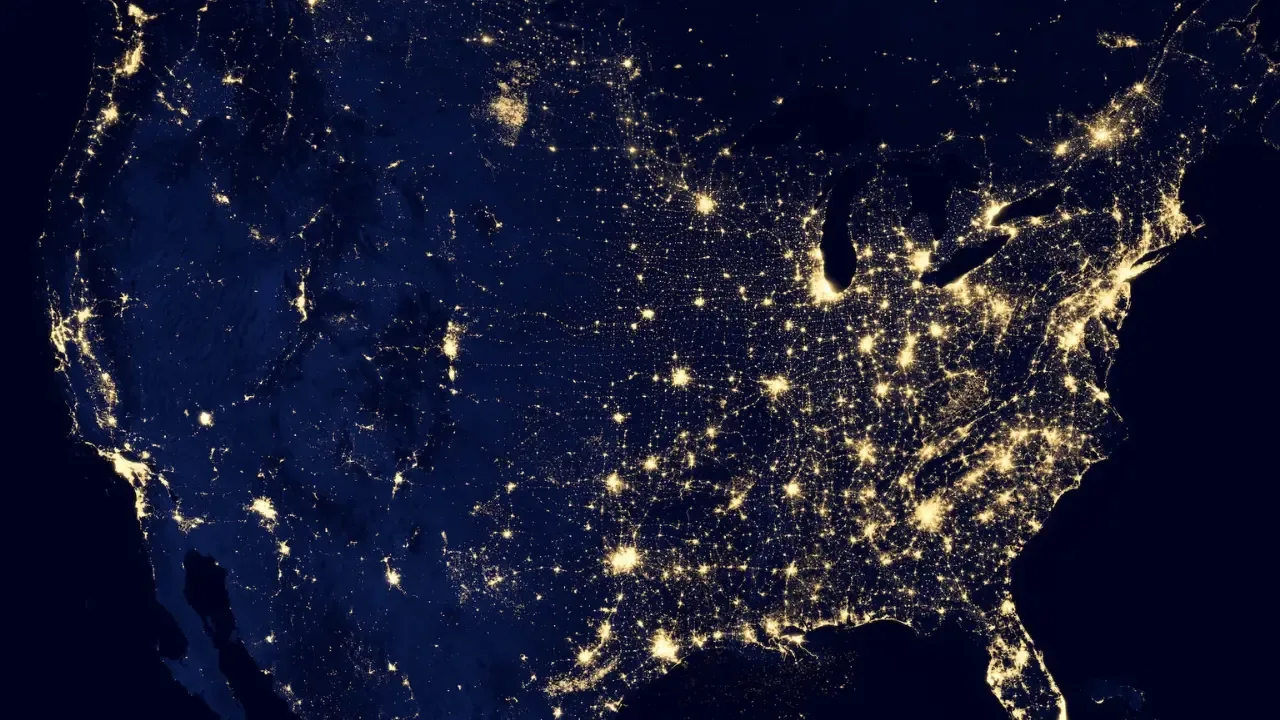
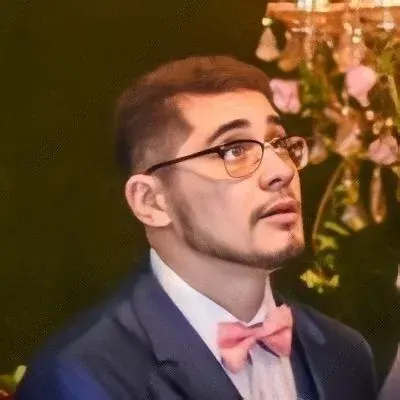
😎📝✨ Supercharge Your Main Thread Tasks with GCD!
So, you're a ⭐ rockstar developer who understands the importance of main thread optimization, and you want to level up your app's performance. 🚀 You've stumbled upon a concept called GCD (Grand Central Dispatch) and you're wondering how to use it to execute tasks on the main thread efficiently. 🎯
In this blog post, we'll explore the question of whether you need to check if you're already on the main thread before using GCD. We'll dive into common issues, provide easy solutions, and empower you to make informed decisions. Let's go! 💪
💡 Understanding the Problem
Imagine you have a callback method that can occur on any thread. However, there's a certain piece of code inside that callback which must run exclusively on the main thread. For instance, you might have UI updates or any other task that requires the main thread's context. 🎨
To perform this task on the main thread, the go-to approach is to use GCD with the dispatch_async
function and pass dispatch_get_main_queue()
as the queue parameter. This queues your task on the main thread's queue, ensuring it will run on the main thread. 🏃♂️
dispatch_async(dispatch_get_main_queue(), ^{
// do work here
});
Now, the big question is: Do you need to check if you're already on the main thread before executing this code? 💭
🚦 To Check or Not to Check?
The short answer: It depends on your situation.
If you know with absolute certainty that your callback is happening on a background thread, and you have no doubt about it, you can safely skip the check. GCD will handle executing your task on the main thread without any issues. ✅
However, if there's even the slightest possibility that your callback might occur on the main thread itself, then it's crucial to perform the check to avoid potential troubles. 🚨
🐇 The "Am I On The Main Thread?" Check
To check if you're currently on the main thread, you can use the NSThread
class method isMainThread
. It's a simple, straightforward way to determine your current thread. 💡
if ([NSThread isMainThread]) {
// Already on the main thread
// Perform task directly
// No need for GCD
} else {
// Execute task on the main thread using GCD
dispatch_async(dispatch_get_main_queue(), ^{
// do work here
});
}
By incorporating this check, you ensure that you're not unnecessarily queuing tasks on the already present main thread, reducing potential overhead and improving performance. 🏋️♂️
⭐ The Sweet Spot - Striking a Balance
Now that you know when to check and when to rely solely on GCD, you have the power to optimize your app's main thread tasks. But remember, like every decision in software development, finding the right balance is crucial. ⚖️
Overusing the main thread check can lead to unnecessary complexity and redundant code, while neglecting the check can introduce subtle bugs and performance issues. It's important to assess your specific use case and make an informed decision. 💪🤓
📣 Your Turn!
We've covered the essentials of using GCD to perform tasks on the main thread, and we're delighted to have you on this ride! 🎉
Now, it's time for you to put this knowledge into practice. Share your thoughts, experiences, or interesting use cases in the comments section below. Let's facilitate a vibrant discussion that helps everyone grow! 🌟🗣️
And don't forget to hit that share button to spread the word about efficient main thread development with GCD. Together, we can optimize our apps and make the world of coding a better place! 🌐💻
Happy coding, and until next time! 👋
📌🔖 Further Reading:
*[GCD]: Grand Central Dispatch