dispatch_after - GCD in Swift?
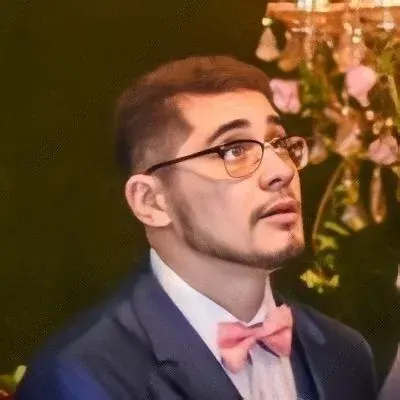
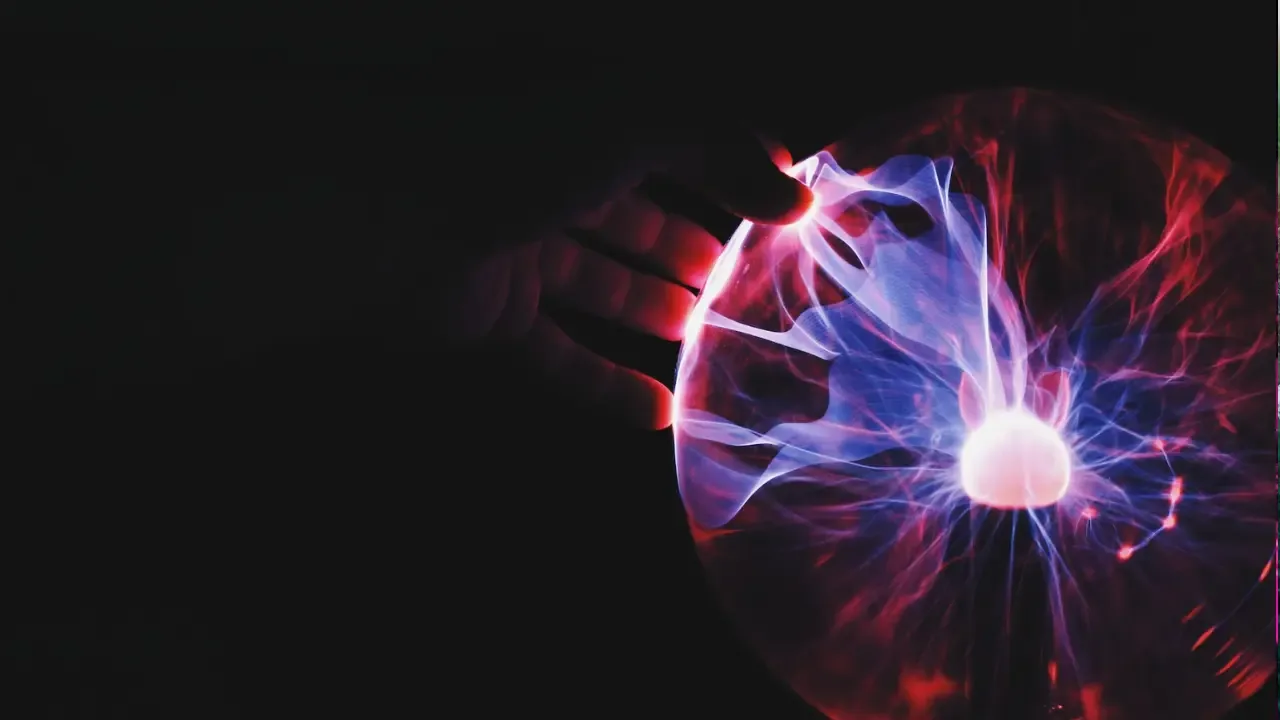
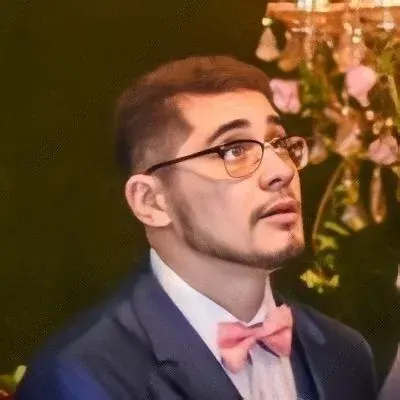
Dispatch_after - GCD in Swift: A Complete Guide 🚀
So, you've stumbled upon the mysterious dispatch_after
function in Swift and you're wondering what it's all about. Don't worry, you're in the right place! In this guide, we'll break down the structure of dispatch_after
and provide easy-to-understand solutions to common issues. Let's dive in! 💪
Understanding the structure of dispatch_after 🕒
The dispatch_after
function is part of Apple's Grand Central Dispatch (GCD) framework, which allows you to perform tasks asynchronously on different queues. Its purpose is to execute a block of code after a specified delay. Here's the structure of dispatch_after
:
dispatch_after(<#when: dispatch_time_t#>, <#queue: dispatch_queue_t?#>, <#block: dispatch_block_t?#>)
<#when>
: Determines when the block should be executed, specified as adispatch_time_t
value. This value can be obtained using functions likedispatch_time
ordispatch_walltime
.<#queue>
: Optional parameter to specify the dispatch queue on which the block should be executed. If you want the block to run on the main queue, you can passnil
.<#block>
: The block of code that you want to execute after the specified delay. This is of typedispatch_block_t
.
Common Issues and Easy Solutions 💡
Issue 1: How do I use dispatch_after
to delay the execution of code?
To delay the execution of code using dispatch_after
, you need to provide a valid when
parameter. One common mistake is not correctly calculating the dispatch_time_t
value. Here's an example that delays the execution of a block by 2 seconds:
let delay: dispatch_time_t = dispatch_time(DISPATCH_TIME_NOW, Int64(2 * NSEC_PER_SEC))
dispatch_after(delay, dispatch_get_main_queue()) {
// Code to be executed after 2 seconds
}
In the above example, we're using dispatch_time
to calculate the appropriate dispatch_time_t
value and passing it to dispatch_after
. We're also specifying that the block should be executed on the main queue.
Issue 2: How do I cancel a block scheduled with dispatch_after
?
Sometimes, you may need to cancel a block that is scheduled with dispatch_after
. To achieve this, you can leverage the power of dispatch_block_cancel
. Here's an example:
var delayBlock: dispatch_block_t?
// Schedule the block
let delay: dispatch_time_t = dispatch_time(DISPATCH_TIME_NOW, Int64(2 * NSEC_PER_SEC))
delayBlock = dispatch_after(delay, dispatch_get_main_queue()) {
// Code to be executed after 2 seconds
}
// Cancel the block
if let delayBlock = delayBlock {
dispatch_block_cancel(delayBlock)
}
In the above example, we're assigning the dispatch_after
call to a variable called delayBlock
. Later, if we decide to cancel the block, we can simply call dispatch_block_cancel
and pass delayBlock
as the parameter.
Conclusion and Next Steps 🏁
Congratulations, you've successfully understood the structure of dispatch_after
and resolved some common issues! Now it's time to put your newfound knowledge into practice. Experiment with different delay durations, dispatch queues, and blocks of code to gain a deeper understanding of how dispatch_after
works.
If you found this guide helpful, make sure to share it with your fellow Swift developers who might be struggling with dispatch_after
. Don't hesitate to leave a comment below if you have any questions or additional tips to share.
Happy coding! ✨🚀