Difference between objectForKey and valueForKey?
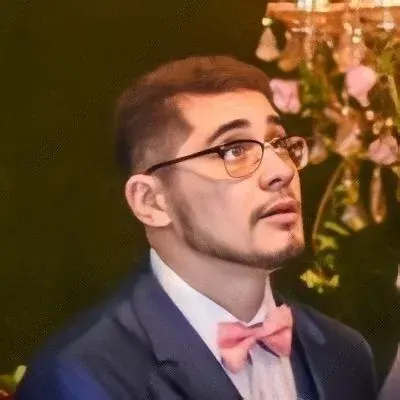
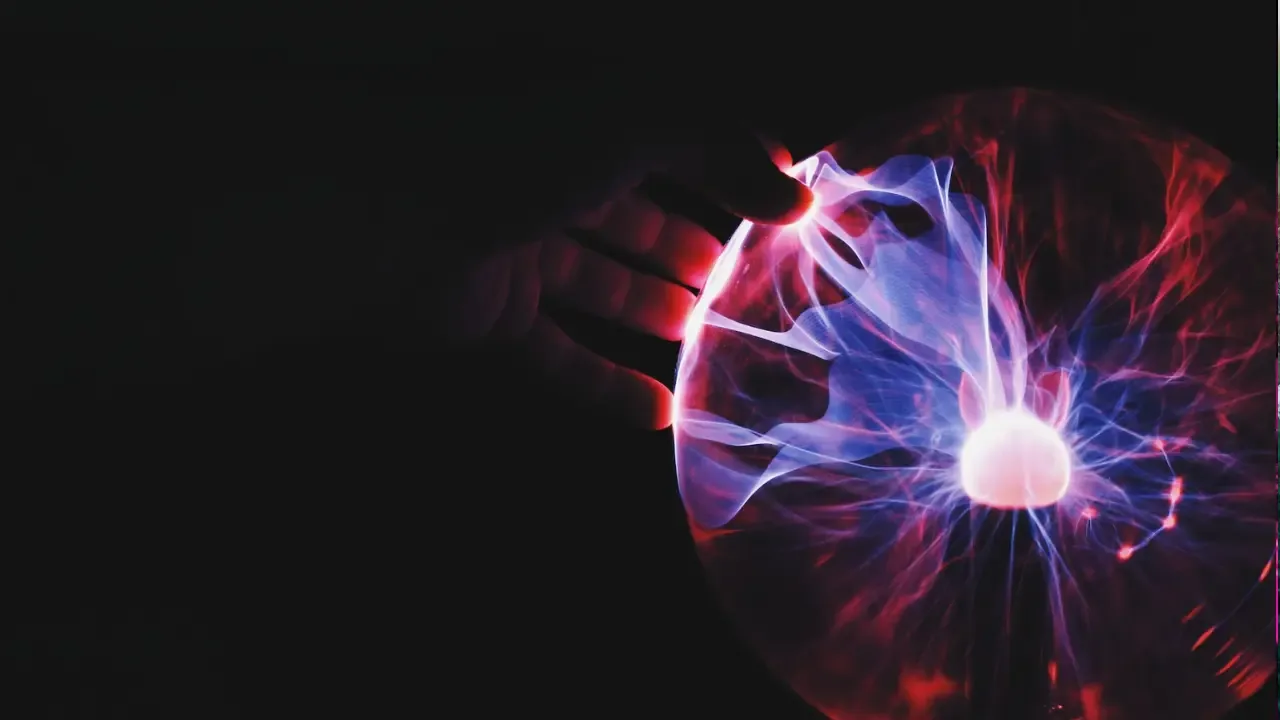
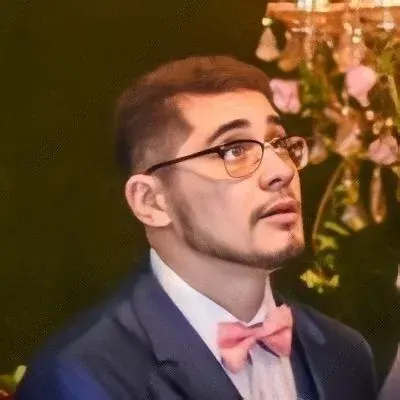
š Understanding the Difference between objectForKey and valueForKey
š” Have you ever encountered the perplexing dilemma of choosing between objectForKey
and valueForKey
? š¤ Don't worry! You're not alone. These two methods may seem similar at first glance, but there are crucial differences that you should be aware of. Let's dive into this topic and unravel the mysteries!
š In a nutshell, both objectForKey
and valueForKey
are methods used in iOS development to access values stored in key-value pairs. However, they have distinct purposes and behaviors.
š The Basics of objectForKey āØ
š§ objectForKey
is a method specifically used with instances of NSDictionary
or NSMutableDictionary
classes in Objective-C. This method allows you to retrieve the object associated with a given key.
š Here's an example to help you understand:
NSDictionary *myDictionary = @{@"name": @"John", @"age": @25};
NSString *name = [myDictionary objectForKey:@"name"];
š In this example, we retrieve the value associated with the key "name" from the myDictionary
object. The variable name
would now hold the value "John".
š Unveiling the Powers of valueForKey āØ
š” On the other hand, valueForKey
is a more versatile method that can be used with various classes such as NSArray
, NSSet
, and NSManagedObject
. It allows you to extract the value for a specific key from an object, including nested keys or keys from a collection of objects.
š Here's a practical example:
class Person: NSObject {
@objc var name: String
init(name: String) {
self.name = name
}
}
let person = Person(name: "John")
let value = person.value(forKey: "name")
š In this Swift example, we create a Person
class with a name
property. By using value(forKey:)
on the person
object, we can extract the value of the name
property, which would be "John" in this case.
ā ļø Beware of Key-Value Coding (KVC) ā ļø
š¢ It's important to note that valueForKey
employs a mechanism called Key-Value Coding (KVC). This means that behind the scenes, it relies on a series of method invocations to fetch the value. This can be advantageous in certain scenarios, such as when working with collections or performing advanced data manipulations. However, it comes with some performance considerations and should be used judiciously.
š” So, Which One Should You Choose? š”
š¤ Now that the differences are clearer, deciding between objectForKey
and valueForKey
becomes easier.
š If you are working with an NSDictionary
or NSMutableDictionary
, stick with objectForKey
for its simplicity and efficiency.
š If you need a more flexible approach to access values, especially with nested keys or when working with collections like NSArray
, consider using valueForKey
.
š§ However, always remember the performance considerations and potential implications of using Key-Value Coding.
šŖ Level Up Your Coding Skills! šŖ
š Now that you have a better grasp of the dissimilarities between objectForKey
and valueForKey
, take your skills to the next level! Experiment with these methods in your projects, and see how they can simplify your code and enhance your development process.
š What's your experience with objectForKey
and valueForKey
? Share your thoughts and any valuable insights with us in the comments below. Let's learn from each other and push boundaries together! š